Overcoming Spring Boot Integration Challenges with AWS
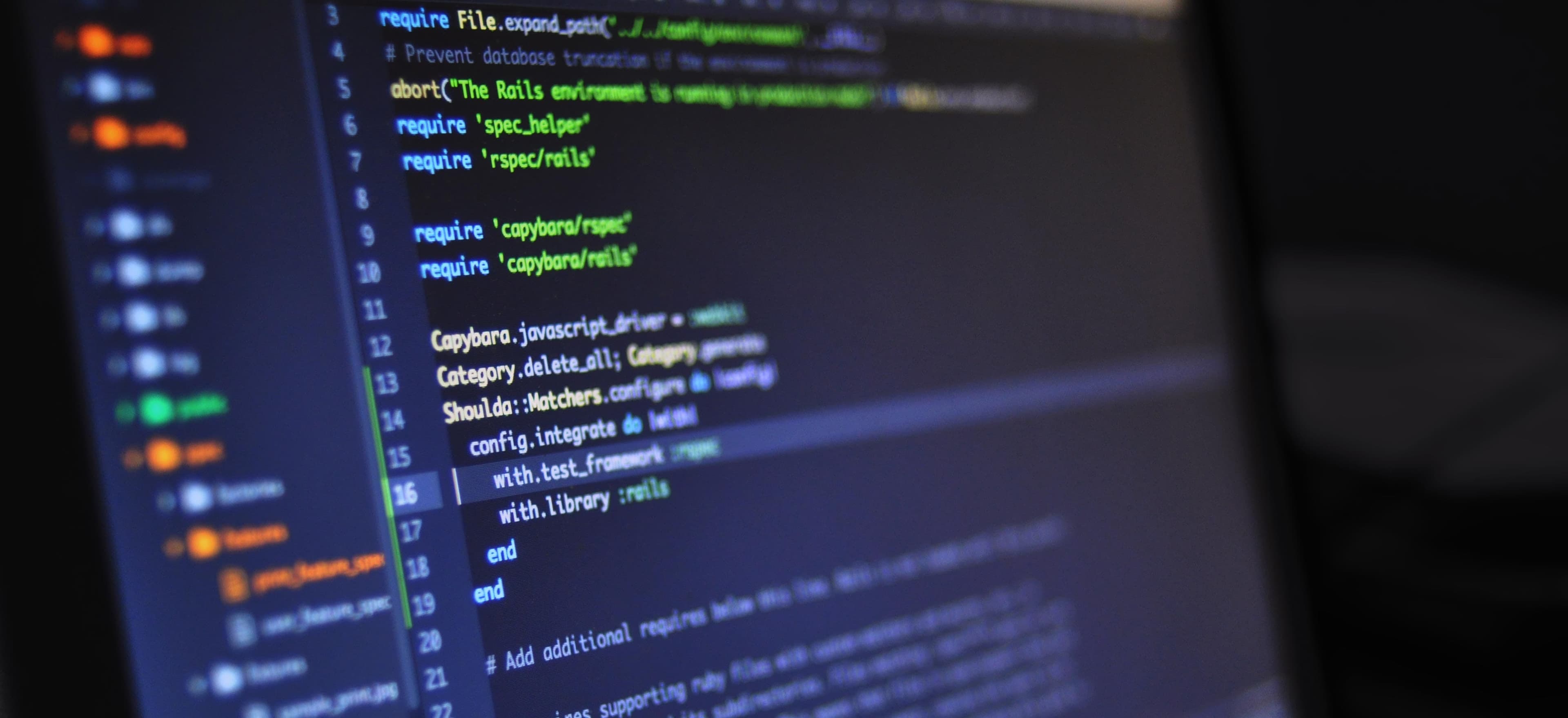
- Published on
Overcoming Spring Boot Integration Challenges with AWS
As developers dive deeper into cloud-based solutions, integrating Spring Boot applications with Amazon Web Services (AWS) has become increasingly common. While this combination presents vast opportunities for creating responsive, scalable applications, it is often coupled with a series of challenges. In this post, we explore common pitfalls when integrating Spring Boot with AWS and provide steps to overcome them.
Understanding Spring Boot and AWS Integration
Spring Boot is an open-source Java-based framework that makes it easy to create stand-alone, production-grade Spring-based applications. AWS is a comprehensive cloud platform offered by Amazon, providing infrastructure as a service (IaaS), platform as a service (PaaS), and software as a service (SaaS).
Together, these technologies amplify a developer's capability to build microservices, enhance data storage options, and scale applications seamlessly.
Key Benefits of Integration
Before diving into the challenges, it is essential to understand why the integration is noteworthy:
- Scalability: AWS services scale effortlessly according to demand.
- Resilience: AWS ensures high availability of services.
- Ease of Deployment: Spring Boot simplifies the deployment process.
- Extensive Tooling: AWS offers a range of tools for monitoring, analytics, and management.
Common Challenges
When integrating Spring Boot with AWS, several challenges frequently arise, including but not limited to:
- Configuration Management
- Security Concerns
- Network Latency
- Data Synchronization
- Testing and Debugging
Let's explore each challenge and the solutions to overcome them.
1. Configuration Management
The Challenge
Spring Boot applications often require numerous configurations, particularly when interacting with various AWS services (like RDS, S3, DynamoDB, etc.). Managing these configurations can quickly become complex.
The Solution
Use AWS Parameter Store or AWS Secrets Manager to store environment configurations securely. This approach not only minimizes hardcoding but also centralizes configuration management.
@Configuration
public class AppConfig {
@Value("${aws.parameter.name}")
private String awsParameter;
@Bean
public SomeService someService() {
return new SomeService(awsParameter);
}
}
Why: Using environment variables enhances security and makes your application flexible across different environments (development, staging, production).
2. Security Concerns
The Challenge
Security is paramount, especially when sensitive information is exchanged between Spring Boot applications and AWS services. Misconfigured IAM roles and credentials can lead to vulnerabilities.
The Solution
Leverage AWS IAM roles and Spring Security to manage access permissions effectively.
@Bean
public AmazonS3 s3client() {
return AmazonS3ClientBuilder.standard()
.withCredentials(new DefaultAWSCredentialsProviderChain())
.withRegion(Regions.US_EAST_1)
.build();
}
Why: By employing IAM roles, applications can avoid hardcoding credentials and utilize managed permissions instead.
3. Network Latency
The Challenge
When your Spring Boot application requests services from AWS (like S3 or DynamoDB), network latency can impact overall performance.
The Solution
Consider utilizing AWS services closer to your application infrastructure. For instance, deploy your Spring Boot application on AWS Elastic Beanstalk or AWS Lambda, optimizing for lower latency.
# Sample configuration for AWS Elastic Beanstalk
environment:
Variables:
SPRING_DATASOURCE_URL: jdbc:mysql://<db-endpoint>
Why: By minimizing the distance between your application and AWS services, you reduce round-trip times and enhance performance.
4. Data Synchronization
The Challenge
Synchronizing data across distributed systems can prove to be a nightmare if not correctly managed. Data discrepancies can lead to system failures and user dissatisfaction.
The Solution
Utilize AWS EventBridge or AWS SNS for event-driven architectures where state changes trigger updates across systems.
@EventListener
public void handleOrderCreated(OrderCreatedEvent event) {
// Business logic to handle event
}
Why: Event-driven architectures promote loose coupling, allowing systems to react to events in real-time, thus maintaining state consistency.
5. Testing and Debugging
The Challenge
When integrating with AWS, the lack of a unified approach for testing and debugging can complicate development.
The Solution
Employ AWS LocalStack to simulate AWS services locally. This solution allows developers to run integration tests without incurring costs or requiring cloud access.
# Running LocalStack with Docker
docker run -e SERVICES=s3,sqs -p 4566:4566 -p 4510-4581:4510-4581 localstack/localstack
Why: LocalStack mimics AWS services, providing a controlled environment for testing and improving debugging processes.
Best Practices for Integration
To maximize efficacy in your Spring Boot and AWS integration, consider the following best practices:
- Use Dependency Injection: Leverage Spring's dependency injection to manage AWS service clients effectively.
- Focus on Resilience: Implement retry patterns and circuit breakers using libraries like Resilience4j or Spring Cloud for fault tolerance.
- Monitoring and Logging: Utilize services like AWS CloudWatch, along with Spring Actuator, to keep an eye on application health and performance.
- Automated Deployment: Incorporate CI/CD practices with AWS CodePipeline to streamline deployment processes.
Closing the Chapter
Integrating Spring Boot with AWS offers immense benefits to developers. While the integration journey is lined with challenges, employing the solutions outlined above can lead to a more secure and efficient application architecture. By focusing on configuration management, security, latency, synchronization, and testing, you can build a robust system ready to take full advantage of the cloud.
For further reading, check out the following resources:
By embracing these patterns and solutions, you can transform your Spring Boot applications into powerful, cloud-native solutions that meet the demands of modern software development. Happy coding!
Checkout our other articles