Why Every Serialization Framework Needs a Unique Transient Annotation
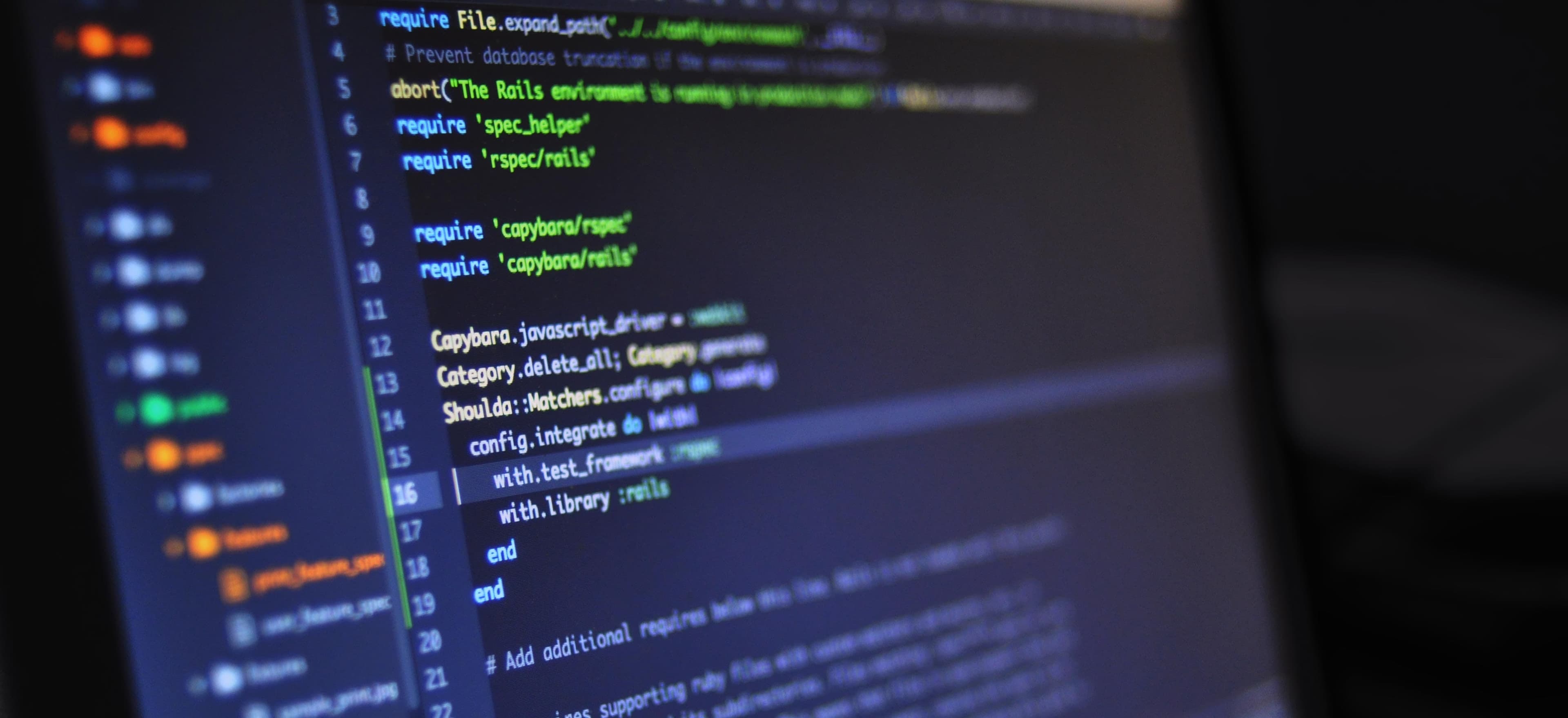
- Published on
Why Every Serialization Framework Needs a Unique Transient Annotation
Serialization is a crucial aspect of software development, especially in Java, where objects frequently need to be converted into a format suitable for storage or transmission. This process can sometimes be confusing due to the nuances of how different frameworks handle this function. One fundamental concept in serialization is the idea of transient fields. In this blog post, we will delve into why every serialization framework should have a unique transient annotation to better manage data during serialization.
Understanding Serialization and Transient Fields
What is Serialization?
Serialization is the process of converting an object into a byte stream. This byte stream can then be saved to disk or sent over the network. In Java, the Serializable
interface plays a key role in this process. When an object's class implements Serializable
, it indicates that instances of this class can be serialized.
What are Transient Fields?
Transient fields are those which should not be serialized. In Java, you can declare a field as transient by using the transient
keyword.
Example:
public class User implements Serializable {
private String username;
private transient String password; // This field will not be serialized
public User(String username, String password) {
this.username = username;
this.password = password;
}
// Getters and Setters
}
In this example, the password
field will not be included in the serialized representation of the User
object, making it a crucial security feature.
Why Different Serialization Frameworks Handle Transient Differently
In Java, many libraries like Gson, Jackson, and Kryo provide serialization functionalities. Each has its own way of handling transient annotations.
- Gson relies on the
transient
keyword but can also use its own@Expose
annotation for controlling serialization fields. - Jackson has its own
@JsonIgnore
annotation, which provides more flexibility and is quite popular amongst developers. - Kryo simply skips the transient fields during the serialization process.
With these differences, managing transient fields can become quite complicated, and developers may face unexpected behaviors.
The Need for a Unique Transient Annotation
1. Consistency Across Frameworks
When working with multiple serialization frameworks, it's essential to have a standard method of defining transient fields. A unique transient annotation would provide a consistent way to manage data that should not be serialized, regardless of which framework you are using.
For example:
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface TransientField {
}
This annotation can now be used across different frameworks, facilitating easy identification of transient fields:
public class User {
private String username;
@TransientField
private String password;
}
2. Enhanced Code Readability
Using a unique transient annotation enhances code readability. When another developer looks at your code, they will instantly understand which fields are intended to be excluded from serialization.
Additionally, it can serve as a documentation tool, reinforcing the importance of security and data management practices in the code.
3. Easier Integration of Custom Serialization Logic
Sometimes, developers want to implement custom serialization logic. Having a unique transient annotation can streamline this process.
Imagine you have a custom serializer that checks for the presence of transient fields:
public class CustomSerializer {
public static String serialize(Object obj) {
Field[] fields = obj.getClass().getDeclaredFields();
StringBuilder json = new StringBuilder("{");
for (Field field : fields) {
if (field.isAnnotationPresent(TransientField.class)) {
continue; // Skip transient fields
}
// Access the field value and append to json (not fully implemented for brevity)
}
json.append("}");
return json.toString();
}
}
In this code, the custom serializer explicitly checks for the @TransientField
annotation, leading to more understandable and maintainable code.
4. Better Control Over Serialization Policy
By adopting a unified annotation, developers can centralize the management of serialization policies. For instance, imagine needing to change the serialization behavior globally for transient fields. You would only need to adjust the code related to the unique annotation rather than modifying each framework's specific implementation.
5. Improved Data Security
Lastly, from a security standpoint, a unique transient annotation helps ensure that sensitive data does not accidentally get serialized. Developers would have a clearer and more explicit way to mark sensitive fields for exclusion.
Best Practices
Having realized the need for a unique transient annotation, it is crucial to adopt some best practices:
- Use Consistently: Ensure all team members understand and utilize this annotation uniformly.
- Document Your Fields: Add comments explaining why certain fields are marked as transient.
- Unit Tests: Write unit tests to verify that transient fields are indeed being skipped during serialization.
Final Thoughts
In the dynamic landscape of software development, especially when dealing with serialization, the absence of a unique transient annotation can lead to inconsistencies, confusing code, and potential security risks.
By implementing a unique transient annotation across all serialization frameworks, developers can achieve better code consistency, readability, security, and control over serialization policies.
If you are using a serialization framework in your Java application, consider adopting or advocating for a standard transient annotation. This small step can provide significant long-term benefits to both developers and the applications they create.
Further Reading
- Java Serialization Tutorial - Oracle Documentation
- Gson User Guide - Official Gson GitHub
- Jackson Documentation - Official Jackson GitHub
By continuously refining our practices, we can craft cleaner, safer, and more maintainable code. Let's redefine how we approach serialization in Java!