Common Challenges in Java Microservices Deployment
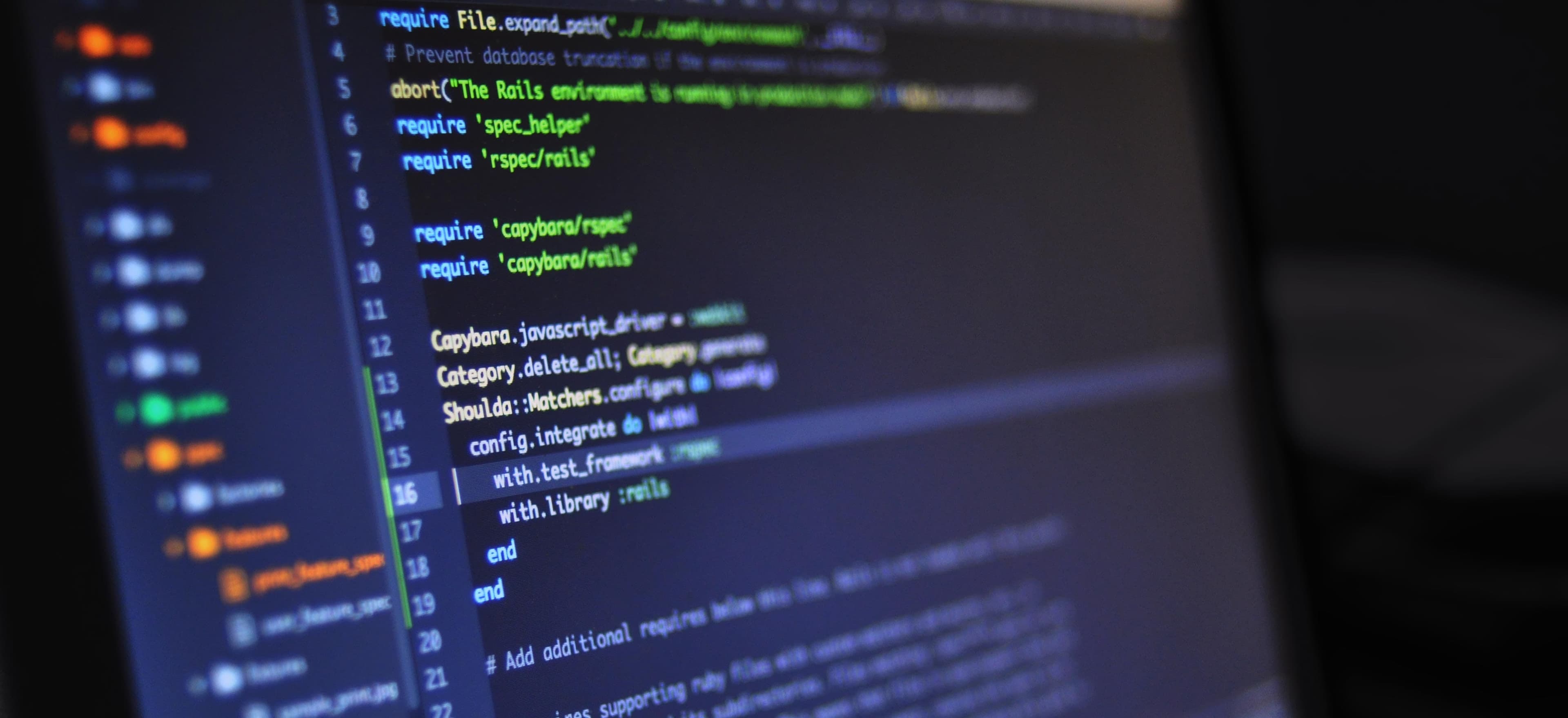
- Published on
Common Challenges in Java Microservices Deployment
In today's era of cloud computing and agility in software development, microservices architecture has gained prominence. By breaking down an application into smaller, independent services, it offers flexibility, scalability, and resilience. However, deploying Java microservices brings with it a set of challenges. In this blog post, we will dive deep into these challenges and provide guidance on how to tackle them effectively.
Table of Contents
Understanding Microservices Architecture
Microservices architecture allows developers to create a suite of small services, each running in its own process and communicating with lightweight mechanisms. As opposed to monolithic applications, microservices enable independent upgrades, scalability, and responsiveness to changing business needs. Java, with its rich ecosystem and frameworks like Spring Boot and Micronaut, is a popular choice for building these services.
Common Challenges
Deploying microservices can be daunting, even for seasoned developers. Here, we will discuss several challenges that can arise when deploying Java microservices, helping you prepare for a smoother deployment journey.
Service Communication
The Challenge
As you deploy multiple services, managing their interactions becomes complex. These inter-service communications can lead to issues such as latency, network failures, and message loss. Standard practices for communication include REST, gRPC, and message brokers.
Why it Matters
Proper communication is vital for maintaining performance and reliability. A failure in service communication can cascade, affecting the entire application.
Code Example: Using Spring Cloud Feign
@FeignClient(name = "payment-service")
public interface PaymentServiceClient {
@PostMapping("/payment")
PaymentResponse initiatePayment(@RequestBody PaymentRequest request);
}
In this example, we create a Feign client to simplify the process of interacting with another microservice (payment-service
). Using Feign alleviates the complexities of HTTP requests and error handling, making service communication easier.
Configuration Management
The Challenge
With all services having unique configurations (database connections, API keys, etc.), managing these configurations can become cumbersome. Hardcoding configurations can compromise security and scalability.
Why it Matters
Effective configuration management ensures that your microservices can adapt to various environments without code changes.
Code Example: Spring Cloud Config
# application.yml
spring:
application:
name: payment-service
cloud:
config:
uri: http://config-server:8888
In this YAML configuration, we define the uri
of our configuration server where all service configurations are centralized. This approach enhances not only management but also maintains consistency across environments.
Data Management
The Challenge
Microservices often use decentralized data storage, leading to potential data consistency issues. Managing data across multiple services can be tricky, especially when ensuring ACID properties.
Why it Matters
Maintaining data integrity is crucial in microservice architecture. Poor data management can result in data discrepancies and ultimately affect business logic.
Possible Solutions
- Event Sourcing: Instead of storing just the current state, this method stores changes as a sequence of events.
- CQRS (Command Query Responsibility Segregation): Separates read and write operations, allowing each to scale independently.
Monitoring and Logging
The Challenge
With multiple microservices, traditional logging and monitoring become insufficient. Tracking issues across services can be a complex task. Lack of visibility can hinder debugging and lead to downtime.
Why it Matters
Comprehensive monitoring is essential for identifying performance bottlenecks and enhancing service reliability.
Code Example: Using Spring Boot with Sleuth and Zipkin
Integrate Spring Cloud Sleuth and Zipkin for tracing.
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-sleuth</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-zipkin</artifactId>
</dependency>
By adding these dependencies, your application can automatically generate trace IDs for requests, making it easier to follow the flow across services and diagnose issues.
Security
The Challenge
Microservices introduce multiple entry points, which can lead to potential security risks. Implementing authentication and authorization across services can be cumbersome.
Why it Matters
Security must be a priority in any deployment architecture. A security breach in one service can compromise the entire system.
Best Practices
- Implement Service-to-Service Authentication using JWT.
- Use an API Gateway for centralized management of API access.
The Bottom Line
Deploying Java microservices can indeed come with its challenges, but understanding these issues and employing strategic practices can alleviate many pain points.
By focusing on service communication, configuration management, data management, monitoring, and security, developers can lay the groundwork for resilient and flexible microservices.
Best Practices Summary:
- Utilize frameworks such as Spring Cloud for simplified service communication and configuration.
- Incorporate monitoring tools like Zipkin to enhance visibility.
- Prioritize security with effective authentication mechanisms.
For further reading, the Spring Cloud documentation provides excellent resources on microservices deployment in Java.
Embrace the microservices architecture and unlock the full potential of your applications while navigating the associated challenges effectively!
Checkout our other articles