Common Gradle Build Issues: Fixing Property Misconfigurations
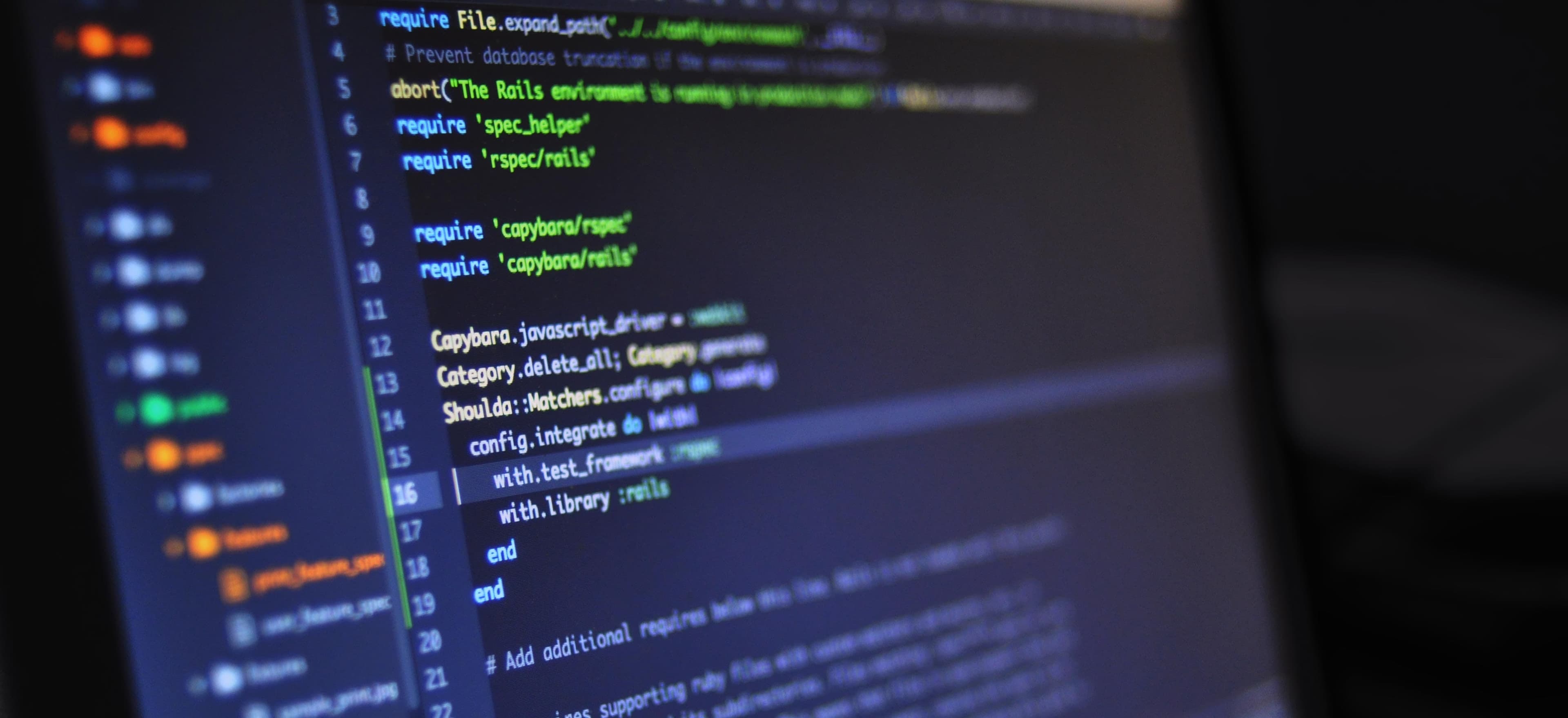
- Published on
Common Gradle Build Issues: Fixing Property Misconfigurations
Gradle is an immensely popular build automation tool widely used in Java development. It provides a flexible way to build, test, and deploy applications. However, developers often run into build issues, especially when it comes to property misconfigurations. In this blog post, we will explore these common issues, how to identify them, and, more importantly, how to fix them effectively.
Understanding Gradle Properties
Gradle properties are essentially key-value pairs that allow you to configure various aspects of your build process. They play a crucial role in customizing builds based on your needs. There are different types of properties:
- Project Properties: Defined in
build.gradle
, local to the project. - System Properties: Defined as JVM arguments.
- Environment Variables: Defined in the operating system, available globally.
Common Misconfigurations
Misconfiguration of properties can lead to build failures or unexpected behavior. Some common misconfigurations include:
- Incorrectly defined property values
- Missing properties
- Overriding necessary properties
- Incompatible property types
Identifying Property Misconfigurations
Before jumping into fixes, it's important to identify the misconfiguration. Gradle provides several ways to help diagnose issues:
- Verbose Logging: Running Gradle with the
--info
or--debug
flag provides detailed output on which properties are being used. - Build Scan: Using the Gradle Build Scan by adding the
--scan
flag not only gives you insights into your builds but also highlights any properties that may not be set correctly.
Here's an example command:
gradle build --info
Example of a Common Issue
Consider the following scenario, where a Java project fails to compile because the source compatibility is not set correctly. You might see an error like this:
Compilation failed; see the compiler error output for details.
Fixing the Issue
The first step to fixing this is to ensure your properties are set in your build.gradle
file appropriately. For instance:
// build.gradle
plugins {
id 'java'
}
sourceCompatibility = '1.8' // Ensures Java 8 compatibility
targetCompatibility = '1.8'
repositories {
mavenCentral()
}
dependencies {
// Define your dependencies
}
Why is Source Compatibility Important?
Setting the source and target compatibility in your build file is crucial. It ensures that your code is compiled for the right Java version. If this is misconfigured, you might end up compiling code that uses features not available in an older Java version, leading to compile-time errors.
Common Gradle Property Misconfigurations and Their Fixes
1. Undefined Properties
When you try to access a property that hasn't been defined, it can lead to runtime exceptions. For instance, if you try to access a custom property like version
without specifying it, you may see an error stating that the property doesn't exist.
Fix
Define the property in your build.gradle
:
ext {
version = '1.0.0'
}
2. Incorrect Property Types
Another common error occurs when properties are assigned incompatible data types. For example, if you assign a string instead of an integer where an integer is expected.
Debugging
If you encounter errors suggesting type mismatches, verify the expected type of the property against your assignment.
Fix
Ensure that the data types match:
ext {
maxVersion = 10 // Integer type
}
3. Overriding Default Properties
Overriding properties like group
or version
can lead to unwanted side effects in multi-module projects.
Fix
Use Gradle's conventions wisely or define your own properties in a way that does not interfere with defaults:
group = 'com.example'
version = '0.1.0'
4. Environment-Dependent Issues
Sometimes properties depend on environment variables. If an environment variable is not set correctly, it can lead to build failures.
Debugging
Check whether your environment variable is correctly configured using the command line:
echo $YOUR_ENV_VARIABLE
Fix
Set the variable correctly in your operating system or IDE, or provide a default value within your build.gradle
like so:
def envVar = System.getenv('YOUR_ENV_VARIABLE') ?: 'default_value'
Best Practices for Gradle Properties
- Use Ext Properties: Use
ext
to define custom properties globally within the project. - Comment Your Properties: Always comment properties in your
build.gradle
to make it clear what they are for. - Keep Defaults Consistent: Maintain consistent naming and defaults across buildings, especially in multi-module setups.
Exploring Further
If you want to dive deeper into Gradle and how to resolve various build issues, consider checking the official Gradle documentation at docs.gradle.org or explore more on troubleshooting at Gradle Troubleshooting.
The Last Word
Gradle property misconfigurations can often be the culprits behind build failures or behavior that was not anticipated. By understanding how to define, override, and troubleshoot these properties, you can streamline your development process and avoid common pitfalls.
Regularly check your properties, use logging tools for diagnostics, and adhere to Gradle's conventions for a smooth build experience. Whether you’re new to Gradle or an experienced developer, understanding the nuances of build properties can significantly enhance your project outcomes.
By implementing these strategies, you can mitigate potential issues and lead your Gradle projects to success. Happy coding!
Checkout our other articles