Overcoming Performance Issues in Java Memory Serialization Cloning
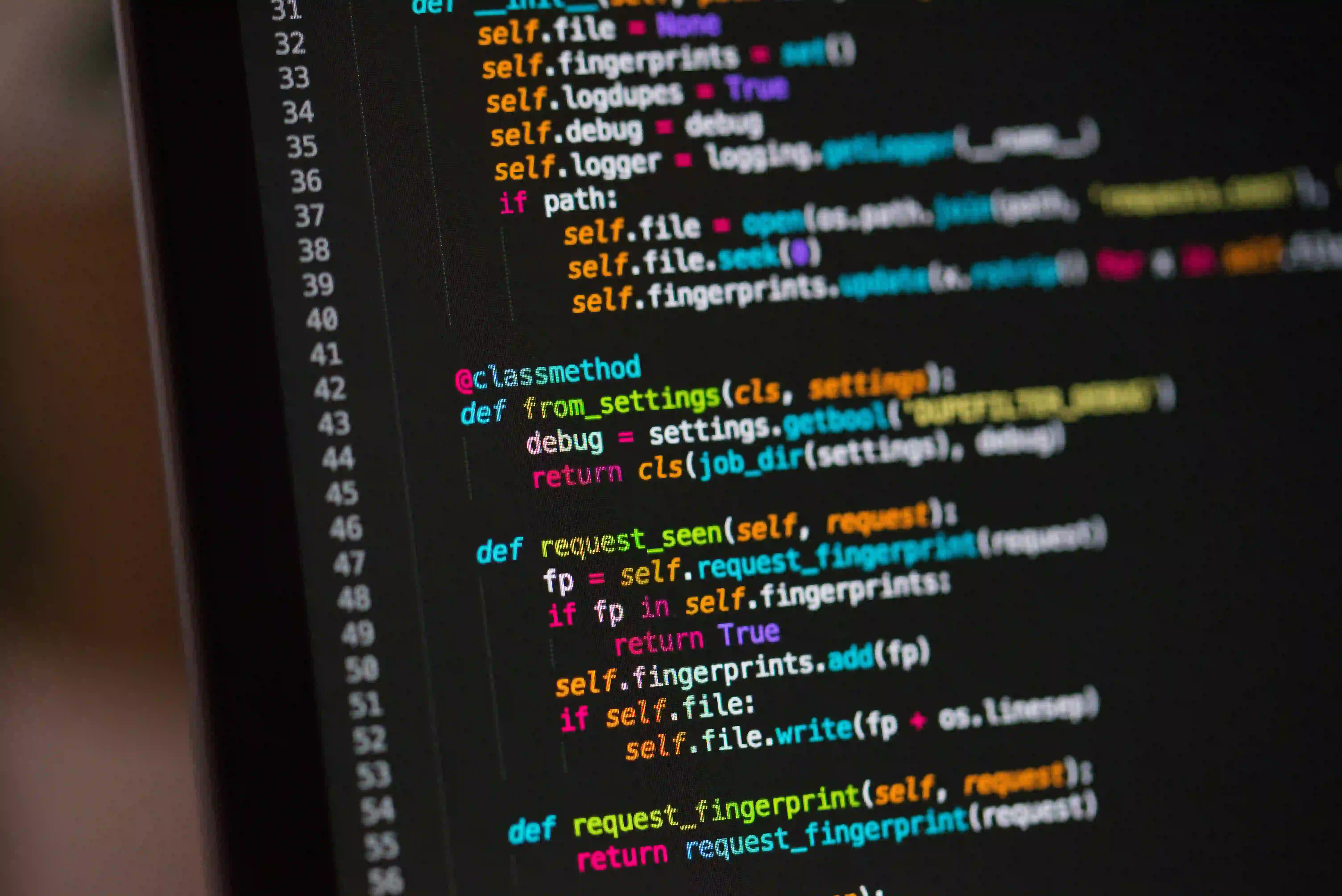
Overcoming Performance Issues in Java Memory Serialization Cloning
In Java programming, serialization and cloning are crucial aspects often used for object persistence and duplication. However, they can lead to serious performance issues if not managed correctly. In this blog post, we will explore the concept of Java serialization and cloning, highlight potential performance pitfalls, and provide solutions to optimize these processes.
Understanding Serialization and Cloning
What is Serialization?
Serialization is the process of converting an object into a byte stream, allowing it to be easily stored on a disk or transferred over a network. The key Java interface for serialization is java.io.Serializable
. An object that implements this interface can be serialized, thus enabling its data to be written out and reconstructed later.
What is Cloning?
Cloning, on the other hand, refers to the process of creating a duplicate of an object. Java provides the Cloneable
interface for this purpose. The clone()
method of the Object
class allows an object to duplicate itself.
Why Use Serialization and Cloning?
- Persistence: Serialization allows you to save the state of an object, which can later be restored.
- Data Transmission: It facilitates the transfer of objects over networks, which is particularly useful in distributed systems.
- Object Duplication: Cloning allows for efficient object copying, avoiding performance costs associated with constructing new instances.
However, when utilized incorrectly, both techniques can lead to significant performance bottlenecks.
Common Performance Issues
1. Serialization Overhead
Serialization can introduce unnecessary overhead, particularly if it is not optimized or used inappropriately. For example, serializing large objects can consume significant CPU and memory resources.
2. Deep Cloning Complexity
Deep cloning entails replicating objects and their referenced objects, which increases time and space complexity. The naive implementation can lead to performance degradation as the size of the object tree grows.
3. Reflection Cost
Java uses reflection to perform serialization and cloning. This can result in slower performance, especially when dealing with a large number of objects or when executed frequently.
Solutions to Optimize Serialization and Cloning
To enhance performance in serialization and cloning, consider the following strategies:
1. Optimize Serialization
To optimize serialization, you can:
-
Use transient Fields: Mark fields that do not need serialization with the
transient
keyword. This reduces the serialized object size.☕snippet.javaclass User implements Serializable { private String name; private transient String password; // Not serialized public User(String name, String password) { this.name = name; this.password = password; } // Getters and Setters }
In this example, the
password
field will not be part of the serialization process, thus shrinking the byte stream. -
Implement Externalizable: Rather than relying solely on the default serialization mechanism, you can implement the
Externalizable
interface for custom serialization logic.☕snippet.javaclass User implements Externalizable { private String name; private String password; public User() {} // Required public no-arg constructor @Override public void writeExternal(ObjectOutput out) throws IOException { out.writeUTF(name); // Do not serialize password intentionally } @Override public void readExternal(ObjectInput in) throws IOException { name = in.readUTF(); // Handle deserialization without password } }
This gives you complete control over the serialization process, potentially boosting performance.
2. Avoid Deep Cloning When Possible
-
Shallow Cloning: Whenever feasible, utilize shallow copying instead of deep cloning. Shallow cloning copies primitive types and references, but avoids the overhead of replicating reference objects.
☕snippet.javaclass Book implements Cloneable { private String title; private Author author; // Reference type @Override protected Object clone() throws CloneNotSupportedException { return super.clone(); // Shallow copy } }
In this scenario, the
Book
object will not instantiate a newAuthor
, merely copying the reference. Ensure that shared references are acceptable in your application logic. -
Prototype Pattern: Consider using a prototype pattern that eliminates the need for repetitive object creation, thus potentially avoiding performance lags.
3. Minimize Reflection
- Cached Accessors: If you're frequently accessing fields during serialization or cloning, consider caching accessors rather than relying on reflection, which can be slow.
4. Use Libraries
Java libraries such as Kryo or Jackson offer faster alternatives to built-in serialization. These libraries can help mitigate common issues with performance.
- Kryo: It provides fast serialization and deserialization. By simply adding a dependency, you can improve object serialization speed dramatically.
<dependency>
<groupId>com.esotericsoftware.kryo</groupId>
<artifactId>kryo</artifactId>
<version>5.0.0</version>
</dependency>
- Jackson: Primarily focused on JSON serialization, it can also serialize Java objects efficiently.
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.13.0</version>
</dependency>
5. Profiling and Monitoring
Finally, leveraging tools like Java VisualVM or YourKit can help identify bottlenecks in serialization and cloning processes. By profiling your application, you can gain insights into the performance of these operations, allowing for targeted optimizations.
Bringing It All Together
Performance issues in Java serialization and cloning can significantly affect application efficiency. By understanding the intricacies of these processes and implementing the strategies discussed, you can mitigate common pitfalls and enhance system performance. Remember, the goal is to find the right balance between object fidelity and performance efficiency.
For further insights into Java serialization and cloning, feel free to explore the official Java documentation on Serialization and Cloning. Happy coding!