Common Pitfalls When Deploying Spring Boot Apps on Tomcat
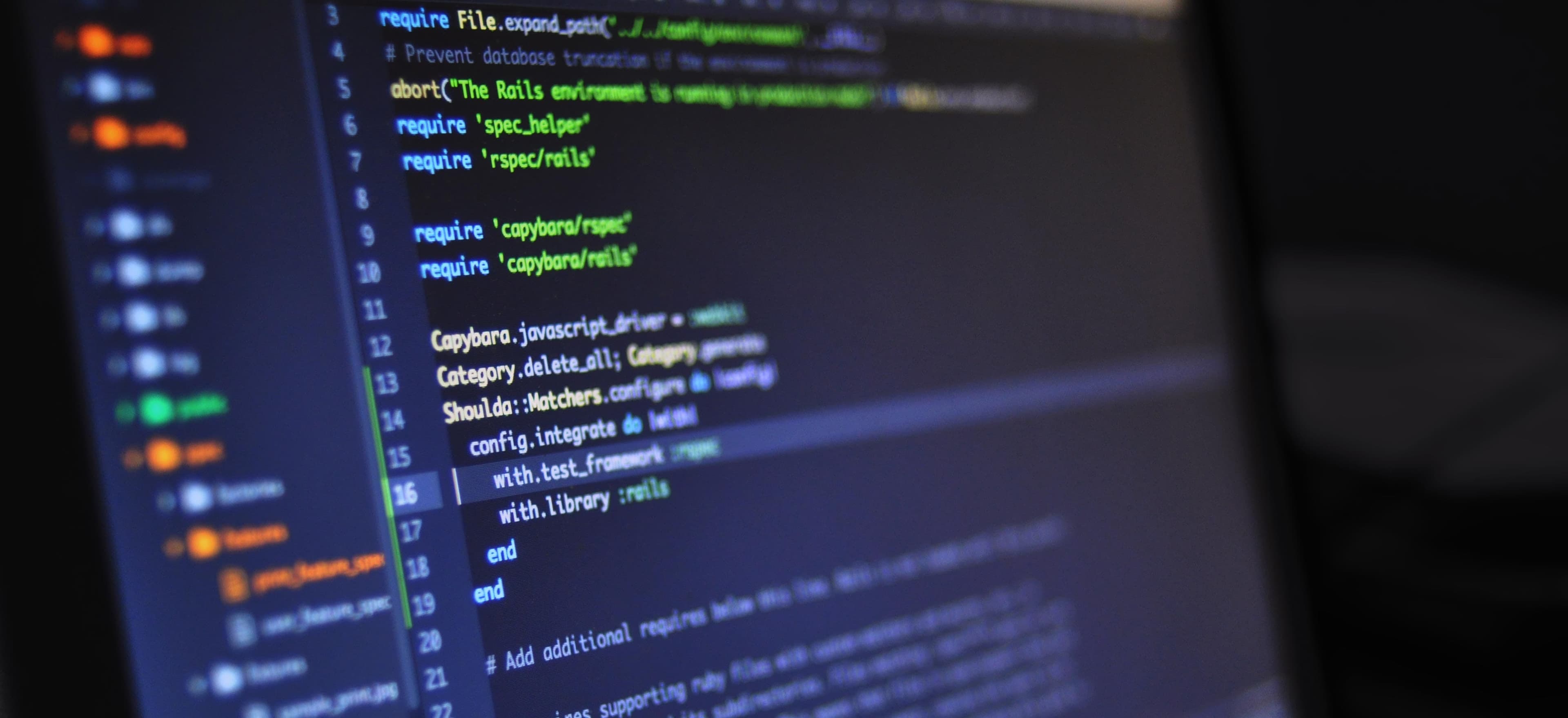
- Published on
Common Pitfalls When Deploying Spring Boot Apps on Tomcat
Spring Boot is a popular framework for building enterprise-grade applications in Java. Combining its simplicity with the robust capabilities of Apache Tomcat creates a powerful development and deployment environment. However, deploying Spring Boot applications on Tomcat can introduce several common pitfalls. In this blog post, we'll explore these challenges, provide solutions, and share best practices to ensure a smooth deployment process.
Understanding Spring Boot and Tomcat
Spring Boot is designed to allow developers to create stand-alone, production-grade Spring-based applications with minimal configuration. While Spring Boot comes with its own embedded server, it also allows for deployment on traditional servlet containers like Tomcat.
Why Deploy on Tomcat?
- Familiarity: Many organizations are accustomed to deploying applications on Tomcat.
- Scalability: Tomcat can handle multiple web applications easily.
- Management: Tomcat comes with management tools for admin tasks and logging.
Common Pitfalls
1. Misconfigured Context Path
Problem: When deploying a Spring Boot application as a WAR file on Tomcat, if the context path isn’t set correctly, it can prevent proper access to the application.
Solution: Specify the context path properly in the application.properties
file.
server.servlet.context-path=/myapp
Commentary: Setting the context path ensures that your application is accessible at the desired URL. If not configured, Tomcat defaults to using the application name as the context path, which may not be what you expect.
2. .WAR File Structure Issues
Problem: Spring Boot applications have a specific structure. Packaging the application incorrectly can lead to ClassNotFound
or NoClassDefFound
errors.
Solution: Ensure you package your application as a WAR in your pom.xml
or build.gradle
file.
Maven Example
<packaging>war</packaging>
Commentary: By defining your project as a WAR, you're informing the build tool to package the application appropriately for deployment onto Tomcat, which is essential for proper functionality.
3. Dependency Conflicts
Problem: Spring Boot automatically includes many dependencies. However, deploying on Tomcat may lead to version conflicts with libraries already bundled in Tomcat.
Solution: Use the provided
scope for dependencies that are already available in Tomcat.
Maven Example
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
Commentary: This approach ensures that you do not bundle the servlets with your application, avoiding conflicts and reducing the final WAR size.
4. Profile Misconfiguration
Problem: Spring profiles can change the application behavior based on the environment. Not configuring profiles correctly can lead to using incorrect database settings or other environment-specific properties.
Solution: Specify your active profile in the context.xml
file in Tomcat.
<Context>
<Parameter name="spring.profiles.active" value="prod" override="false"/>
</Context>
Commentary: By setting the active profile through the Tomcat configuration, you can ensure your application behaves appropriately in the production environment.
5. Resource Path Issues
Problem: Static resources like CSS and JavaScript files may not be served properly if the resource path is not correctly configured.
Solution: Set the resource handler in your Spring Boot application.
Code Example
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/static/**")
.addResourceLocations("classpath:/static/");
}
}
Commentary: This configuration allows Spring to serve static resources from the specified directory, ensuring that they are loaded correctly when accessed.
6. Thread Pool Configuration
Problem: Tomcat has its own threading model, and not configuring it properly can lead to performance bottlenecks.
Solution: Adjust the Tomcat thread pool settings in the server.xml
file.
<Connector port="8080" protocol="HTTP/1.1"
connectionTimeout="20000"
redirectPort="8443"
maxThreads="200" />
Commentary: Tuning these settings helps handle concurrent requests more efficiently, especially under high load.
7. Lack of Logging Configuration
Problem: Not configuring logging can result in missing valuable insights, especially in production.
Solution: Use Spring Boot's built-in logging with a proper configuration in the application.properties
.
logging.file.name=myapp.log
logging.level.root=INFO
logging.level.org.springframework=DEBUG
Commentary: Effective logging is crucial for diagnosing issues in production. Properly configuring your logging can simplify troubleshooting.
8. Missing Exception Handling
Problem: Unhandled exceptions may lead to uncaught errors, resulting in a poor user experience.
Solution: Implement a global error handler using @ControllerAdvice
.
Code Example
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleAllExceptions(Exception ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Commentary: A global exception handler ensures you capture issues that might arise throughout your application, allowing for a more controlled response rather than default error pages.
To Wrap Things Up
Deploying Spring Boot applications on Tomcat is a robust solution for many web applications. However, it's essential to be aware of the common pitfalls that can lead to deployment issues. By addressing context paths, packaging structures, dependency management, profile configurations, resource paths, threading, logging, and exception handling, you can avoid these typical challenges.
For further reading on Spring Boot deployment, check out the Spring Boot official documentation. Understanding these best practices will not only boost your confidence as a developer but also enhance the reliability of your applications in production.
By diligently adhering to these guidelines, you'll ensure that your Spring Boot application seamlessly integrates with Apache Tomcat, allowing you to focus on delivering powerful functionality without worrying about deployment mishaps.
Checkout our other articles