Mastering IDE Inspections for Custom Java Annotations
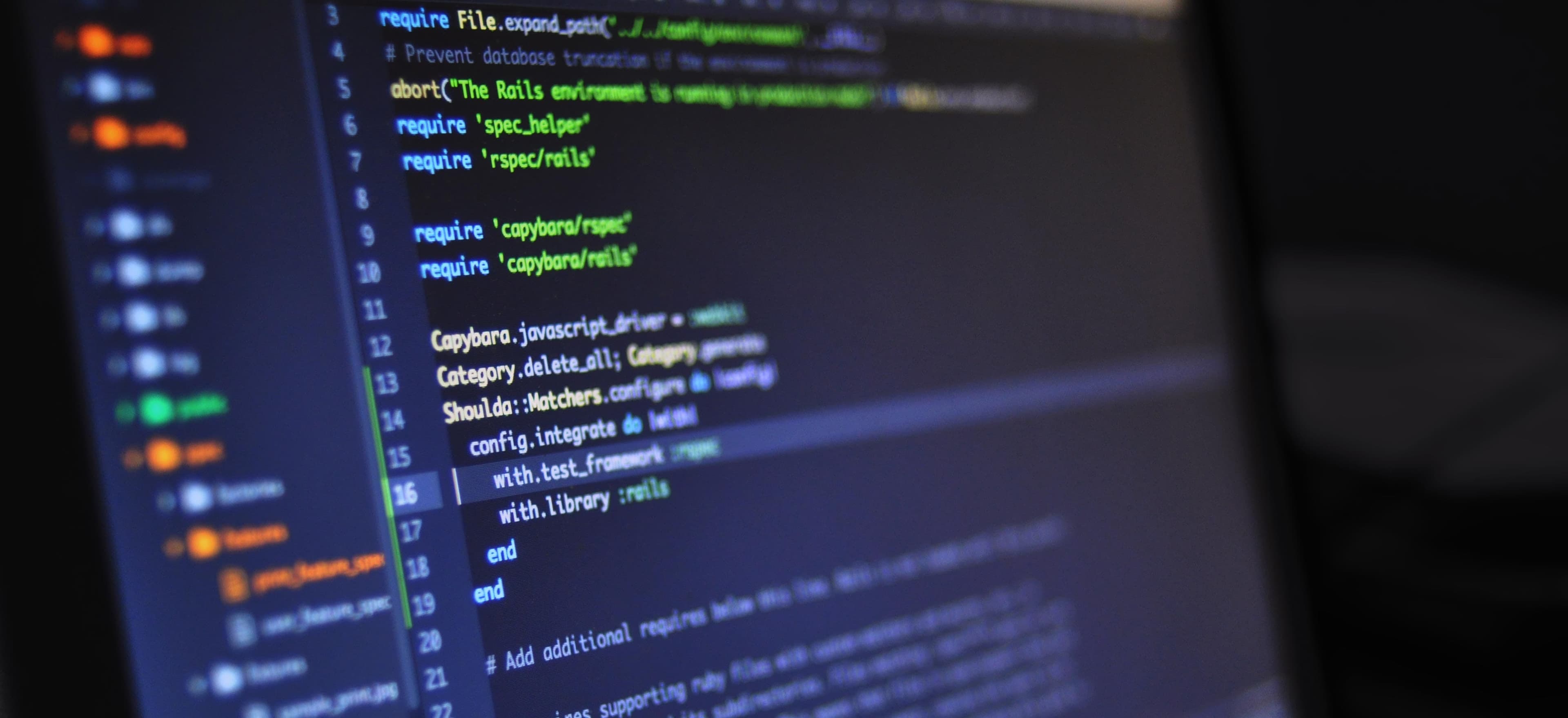
- Published on
Mastering IDE Inspections for Custom Java Annotations
In software development, maintaining clean, efficient, and understandable code is paramount. Java annotations offer a powerful way to add metadata to your code. Custom annotations can streamline processes significantly. With growing complexity in modern applications, having an effective Integrated Development Environment (IDE) can further enhance your coding efficiency. In this post, we will dive into custom Java annotations, understand how to implement them, and leverage IDE inspections for better code quality.
Table of Contents
- What Are Java Annotations?
- Creating Custom Annotations
- IDE Inspections: Purpose and Utility
- Implementing IDE Inspections for Custom Annotations
- Examples and Best Practices
- Conclusion
1. What Are Java Annotations?
Java annotations are predefined markers that add metadata information to your Java code (classes, methods, fields, etc.). They do not change the way your code operates but offer additional context that tools or libraries can utilize.
For example:
@SuppressWarnings("unchecked")
void myMethod() {
// code
}
Here, the @SuppressWarnings
annotation tells the compiler to ignore specific warnings. Annotations are defined with the @interface
keyword, and they can serve various purposes including configuration, documentation, and runtime processing, making them an essential project feature.
2. Creating Custom Annotations
Creating custom annotations in Java is quite straightforward. Through custom annotations, you can design a specific behavior for your application that fits precisely into your requirements.
Step 1: Define the Annotation
Here is a simple example of a custom annotation named @DeprecatedMethod
:
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* Annotation to mark a method as deprecated.
*/
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface DeprecatedMethod {
String reason() default "No reason provided";
}
Explanation:
- @Retention: Indicates how long the annotation is retained.
RetentionPolicy.RUNTIME
means it will be available at runtime. - @Target: Specifies the types of elements the annotation can be applied to, in this case, methods.
Step 2: Use the Annotation
You can now use the @DeprecatedMethod
annotation in your code:
public class Example {
@DeprecatedMethod(reason = "Use newMethod() instead.")
public void oldMethod() {
// old implementation
}
public void newMethod() {
// new implementation
}
}
By marking oldMethod
with @DeprecatedMethod
, you essentially communicate to other developers that this method should no longer be used.
3. IDE Inspections: Purpose and Utility
IDE inspections help to identify potential code quality issues before they become real bugs. Modern IDEs like IntelliJ IDEA or Eclipse come with built-in inspections to detect problems related to code style, performance, and correctness.
Why Use Inspections?
- Immediate Feedback: Catch issues as you code.
- Consistency: Enforce coding standards across your project.
- Improved Maintenance: Easier to manage and refactor with fewer surprises down the line.
4. Implementing IDE Inspections for Custom Annotations
Create a Custom Inspection
To enhance your development experience, you can create a custom inspection in your IDE aimed at your custom annotations.
Step 1: Configure the Custom Inspection
In IntelliJ IDEA, write a custom inspection by extending the AbstractBaseUastInspection
class. This approach allows you to define the specific behavior you want when the custom annotation is present.
Here is a basic example:
import com.intellij.codeInspection.LocalInspectionTool;
import com.intellij.codeInspection.ProblemsHolder;
import com.intellij.psi.PsiMethod;
import com.intellij.psi.JavaElementVisitor;
public class DeprecatedMethodInspection extends LocalInspectionTool {
@Override
public void checkMethod(PsiMethod method, ProblemsHolder holder, boolean isOnTheFly) {
if (method.hasAnnotation("your.package.DeprecatedMethod")) {
holder.registerProblem(method.getNameIdentifier(), "This method is deprecated, please use the recommended method.");
}
}
}
Explanation:
- checkMethod: This method checks each method for the presence of
@DeprecatedMethod
. - registerProblem: If the annotation is found, it registers a problem that highlights the method in the IDE.
Step 2: Testing the Inspection
Now that the inspection is in place, writing a test case can help ensure the inspection behaves as expected. Use the following code for your test:
import org.junit.Test;
public class DeprecatedMethodInspectionTest {
private final DeprecatedMethodInspection inspection = new DeprecatedMethodInspection();
@Test
public void testDeprecatedMethod() {
String code = "@your.package.DeprecatedMethod\n" +
"public void oldMethod() {}";
// Invoke inspection logic
// Validate results in IDE
}
}
This simplified example illustrates how you can set up a test case to validate the inspection you just created.
5. Examples and Best Practices
-
Use Clear Descriptions: Always ensure that your annotations have clear purposes. For instance, if you're creating a
@ForTesting
, clearly describe that it is meant for testing scenarios. -
Follow Naming Conventions: Maintain standard naming conventions for your annotations (
@UseCase
,@Tested
, etc.). -
Document Your Annotations: Consider adding Javadocs to your annotations that clearly specify their purpose and usage.
-
Leverage Existing Tools: Utilize tools like Checkstyle or SonarQube to automate some inspections as part of your CI/CD pipeline.
-
Integrate with Build Tools: If you're using Gradle or Maven, ensure that the annotations are processed correctly during the build phase.
To Wrap Things Up
Custom Java annotations are powerful tools that, when paired with effective IDE inspections, can lead to cleaner, more maintainable code. By utilizing the structure and flexibility of annotations alongside robust inspection processes, you enhance not only your individual coding practices but also the quality of projects as a whole.
Facilitating a clear communication medium through annotations and establishing coding standards through inspections ensures a unified understanding across development teams. Whether you're managing a small project or a large enterprise application, mastering these tools is a step towards efficient and error-free programming.
For more information about annotations, visit the Oracle Java Documentation. Happy coding!