Struggling with On-Heap vs Off-Heap Memory? Here's Why!
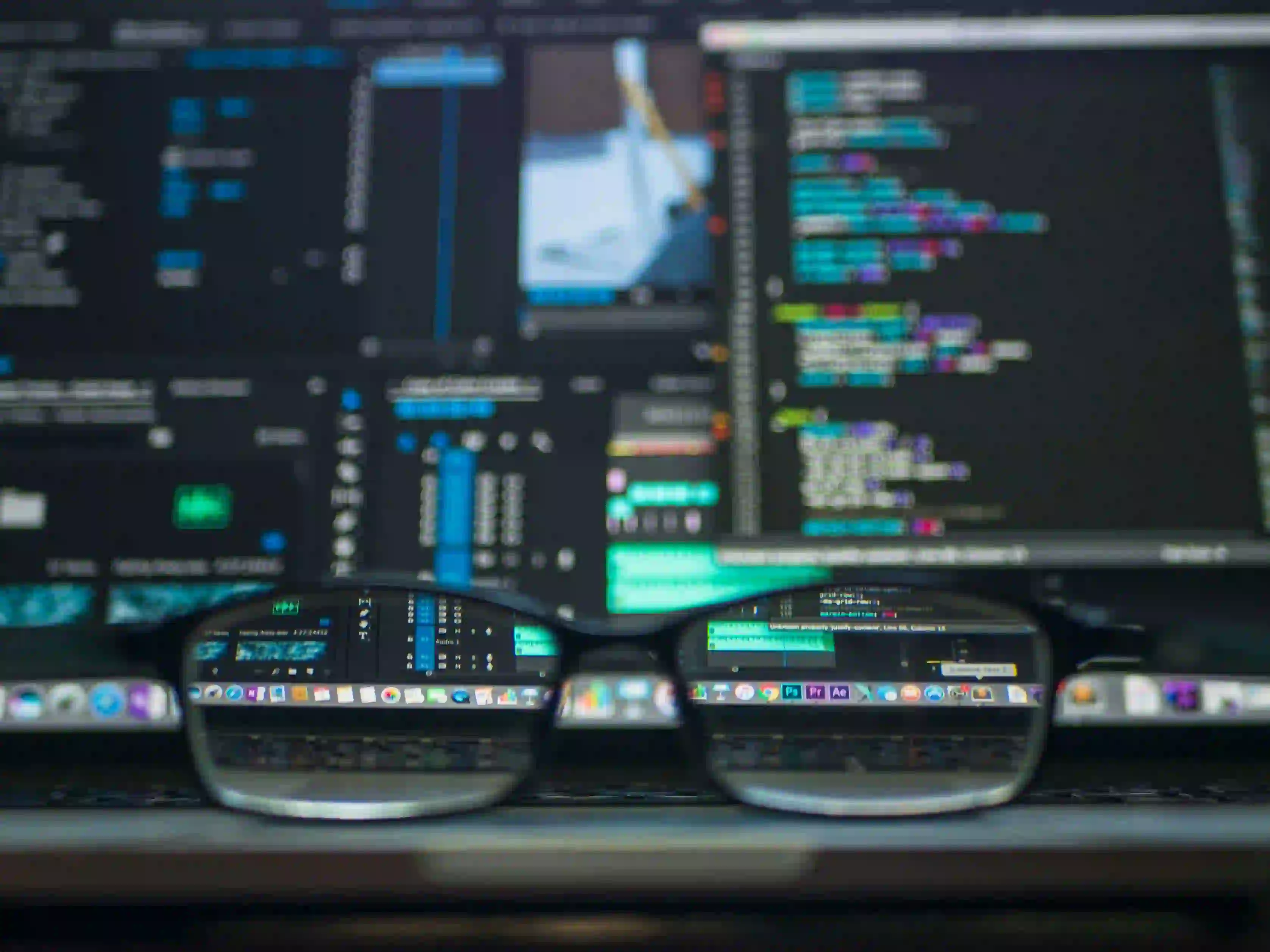
Struggling with On-Heap vs Off-Heap Memory? Here’s Why!
Java memory management can sometimes feel like an intricate puzzle. Two significant players in this sphere are On-Heap and Off-Heap memory. Understanding how they function can greatly improve your application performance and resource management.
In this blog post, we will explore the key differences between On-Heap and Off-Heap memory, their use cases, advantages, and disadvantages, along with relevant code examples. Let’s dive in!
What is On-Heap Memory?
On-Heap memory refers to the memory that is managed by the Java Virtual Machine (JVM). When you create an object in Java, it is stored in the heap. The JVM is responsible for allocating and deallocating this memory. Here are some key points about On-Heap memory:
- Managed by JVM: The garbage collector (GC) takes care of memory management.
- Garbage Collection: Objects in On-Heap memory are subject to garbage collection.
- Accessibility: On-Heap objects can be accessed directly by Java code.
Example of On-Heap Memory Usage
public class OnHeapExample {
public static void main(String[] args) {
String[] words = new String[1000000]; // Allocate an array of Strings
for(int i = 0; i < words.length; i++) {
words[i] = "Hello, World!"; // Each string is stored in On-Heap memory
}
// The garbage collector will clean up once words goes out of scope
}
}
Why Use On-Heap Memory?
- Simple Memory Management: The JVM manages memory allocation and deallocation.
- Developer-Friendly: You don’t need to worry about manual memory management.
- Built-in Garbage Collection: Automatically reclaims memory for unreferenced objects.
What is Off-Heap Memory?
Off-Heap memory, on the other hand, refers to memory that's not managed by the JVM. It exists outside the garbage collector’s control and is generally allocated using native code. Here are some essential aspects of Off-Heap memory:
- Not Managed by JVM: Memory management needs to be handled manually.
- Higher Performance: Off-Heap can reduce GC overhead and improve performance.
- Direct Byte Buffers: Java provides
java.nio
package for Off-Heap memory access.
Example of Off-Heap Memory Usage
import java.nio.ByteBuffer;
public class OffHeapExample {
public static void main(String[] args) {
// Allocate 1 MB of Off-Heap memory
ByteBuffer buffer = ByteBuffer.allocateDirect(1024 * 1024);
buffer.put("Hello, Off-Heap!".getBytes());
// Off-Heap memory management needs to be done manually.
}
}
Why Use Off-Heap Memory?
- Reduced GC Pressure: Helps avoid frequent garbage collection cycles.
- Increased Performance: Especially beneficial for applications with large datasets.
- Better Memory Control: You have greater control over memory allocation.
On-Heap vs Off-Heap: Key Differences
| Feature | On-Heap Memory | Off-Heap Memory | |--------------------------------|------------------------------|--------------------------------| | Managed by JVM | Yes | No | | Subject to Garbage Collection | Yes | No | | Access Speed | Slower due to GC | Faster due to direct access | | Memory Management Flexibility | Limited | Greater flexibility | | Use Case | General purpose | High-performance applications |
When to Use On-Heap Memory?
- Standard Applications: If your application does not have stringent memory performance requirements.
- Quick Development: Fast object manipulations without worrying about memory management.
- Small Data Volumes: When the data size is manageable within the heap.
When to Use Off-Heap Memory?
- High-Performance Applications: Ideal for systems dealing with large data sets or high transaction rates.
- Real-time Processing: Low-latency systems that handle quick operations.
- Memory Constraints: When you are close to the heap size limit and need additional storage.
Best Practices for Using Off-Heap Memory
- Monitor Memory Usage: Keep an eye on memory allocation and deallocation patterns.
- Minimize Fragmentation: Allocate and free memory efficiently to prevent fragmentation.
- Use Native Libraries: Make use of native libraries that can better manage Off-Heap memory.
Performance Implications
Using Off-Heap memory can provide significant performance gains, especially in data-intensive applications. For example, caching frameworks like Apache Ignite employ Off-Heap memory techniques to cache large data sets outside the heap, thus improving performance drastically.
However, it comes at the cost of increased complexity in the code due to manual management requirements. Hence, deciding between On-Heap and Off-Heap should always consider the trade-offs.
My Closing Thoughts on the Matter
In conclusion, the choice between On-Heap and Off-Heap memory can significantly impact your Java application's performance and memory management capabilities. Use On-Heap memory for simplicity and rapid development, but don't shy away from Off-Heap memory for high-performance scenarios.
As a developer, it's essential to grasp these concepts and leverage them according to your application needs. Always remember that understanding memory management leads to better resource usage.
By recognizing the strengths and weaknesses of On-Heap and Off-Heap memory, you can make informed decisions that lead to highly efficient Java applications.
For further reading, consider exploring the following resources:
- Java Memory Management
- Understanding JVM Heap & Stack
Happy coding!