Overcoming Deadlocks in Concurrent Applications with Guava
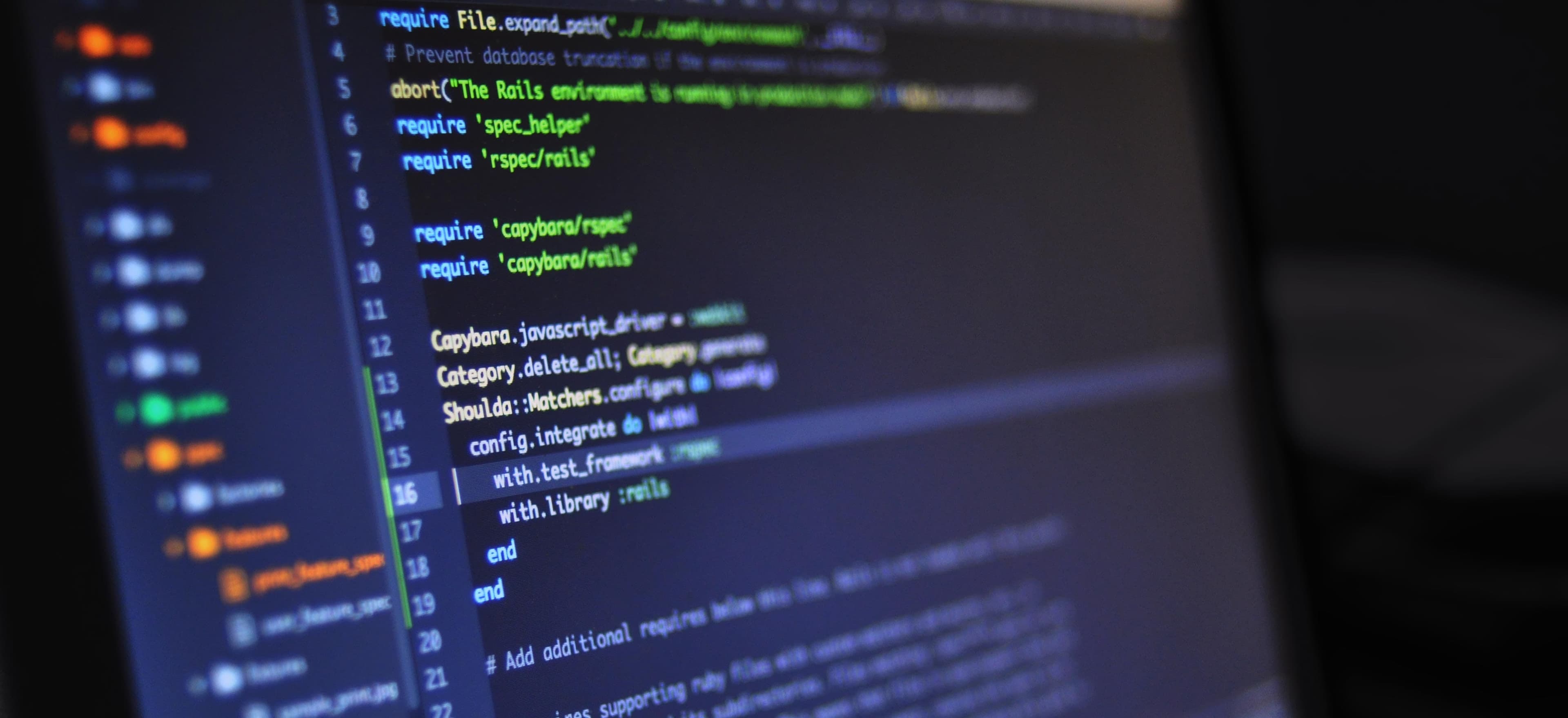
- Published on
Overcoming Deadlocks in Concurrent Applications with Guava
Deadlocks can be among the most challenging issues to address in concurrent programming. When multiple threads are blocked and waiting for resources held by each other, the application can come to a complete halt. Fortunately, libraries like Guava provide tools to mitigate and, ideally, prevent deadlocks from occurring in your Java applications. In this blog post, we'll delve into the problem of deadlocks and how you can leverage Guava's features to reduce the chances of encountering them in concurrent applications.
Understanding Deadlocks
A deadlock occurs in a multi-threaded environment when two or more threads are waiting indefinitely for each other to release resources.
Example of a Deadlock Scenario
Consider the following situation with two threads (T1 and T2) and two resources (R1 and R2).
- Thread T1 locks resource R1.
- Thread T2 locks resource R2.
- T1 attempts to lock R2 while holding R1.
- T2 attempts to lock R1 while holding R2.
In this scenario, both threads are left waiting indefinitely as neither is able to proceed.
To avoid deadlocks, understanding the common causes is the first step. These include:
- Resource Holding: When threads hold resources while waiting for additional resources.
- No Preemption: Once a resource is allocated to a thread, it cannot be forcibly taken away.
- Circular Wait: A set of threads are all waiting for resources held by each other.
Introducing Guava
Guava is a popular open-source library from Google that enhances Java's collections framework, concurrency utilities, and more. One of the strengths of Guava is its effective concurrency tools that can help minimize the risk of deadlocks.
Key Features of Guava for Concurrency
- Immutable Collections: Immutable collections do not change, which reduces the chances of thread interference.
- ListenableFuture: This abstraction enhances Futures for better handling of asynchronous results.
- RateLimiter: Controls the rate of execution for operations, which can limit resource contention.
- Striped Locks: Allows for a more granular locking mechanism, reducing the chances of deadlocks.
How to Use Striped Locks
Now, let’s focus on Striped Locks as a primary method to help avoid deadlocks. Striped locks allow you to segment locks into groups, reducing contention. This method is particularly useful when you have multiple threads trying to acquire locks on shared resources.
Example Code Snippet
import com.google.common.util.concurrent.Striped;
import java.util.concurrent.locks.Lock;
public class StripedLocksExample {
private final Striped<Lock> locks = Striped.lock(16); // 16 stripes
public void performTask(int taskId) {
Lock lock = locks.get(taskId % 16); // Acquire lock based on hash
lock.lock();
try {
// Critical section logic
System.out.println("Performing task with ID: " + taskId);
doWork(taskId);
} finally {
lock.unlock();
}
}
private void doWork(int taskId) {
// Simulate processing time
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Explanation of the Code
-
Striped Locks: We create a
Striped<Lock>
object that manages multiple locks. This allows segmentation, meaning you can reduce the chances of contention and, thereby, deadlock. -
Acquiring Locks: Each thread gets a lock corresponding to the task's ID modulus the number of stripes.
-
Critical Section: Only one thread can execute the
doWork
method for that specific ID at a time, ensuring thread safety without blocking the bigger lock pool.
This approach effectively reduces the chances of deadlocks by allowing threads to hold different locks rather than requiring exclusive access to a single lock that can block others.
Leveraging Futures to Avoid Deadlocks
Another potent feature of Guava is its ListenableFuture, which adds a callback mechanism. This way, you can define actions upon completion of a task without blocking a thread, thus avoiding deadlocks.
Example Code Snippet for ListenableFuture
import com.google.common.util.concurrent.*;
import java.util.concurrent.Executors;
public class FuturesExample {
private final ListeningExecutorService executorService =
MoreExecutors.listeningDecorator(Executors.newFixedThreadPool(10));
public void executeTask() {
ListenableFuture<Integer> future = executorService.submit(() -> {
// Simulate task execution
Thread.sleep(200);
return 42; // Dummy result
});
future.addListener(() -> {
try {
Integer result = future.get(); // Getting the result
System.out.println("Task completed with result: " + result);
} catch (Exception e) {
System.err.println("Error while executing task: " + e.getMessage());
}
}, executorService);
}
}
Explanation of the Code
-
ListeningExecutorService: This is a special implementation of
ExecutorService
that allows tasks to add callbacks. -
Adding a Callback: Upon the completion of the task, a listener is added to handle the result without blocking the main execution thread.
-
Handling the Result: We retrieve the result only after the task is complete, which avoids holding up any resources unnecessarily.
Best Practices to Prevent Deadlocks
While Guava provides excellent tools, here are additional best practices:
-
Lock Order: Always acquire locks in a consistent global order to avoid circular wait conditions.
-
Timeouts: Implement timeouts when trying to acquire locks. This allows the system to back out and retry if it fails to acquire a lock.
-
Reduce Lock Scope: Keep the scope of locks as small as possible. Only lock resources when absolutely necessary.
-
Use Higher-Level Concurrency Tools: Leverage collections and concurrent data structures provided by Java and libraries like Guava which manage complex concurrency issues internally.
The Last Word
Deadlocks can significantly hinder application performance and reliability. By employing tools like Guava’s striped locks and ListenableFuture, we can effectively reduce the risk of deadlocks in our Java applications.
By understanding the nature of deadlocks and properly utilizing the features of Guava, developers can make informed decisions on how to design concurrent systems that are not only robust but also performant.
For further reading about concurrency in Java, you can check out Java Concurrency in Practice which gives in-depth insights into managing concurrency effectively.
If you're interested in Guava and want to explore its other features, be sure to visit the Guava GitHub Repository. Happy coding!