Mastering Java's Math.pow: Recursive vs Iterative Pitfalls
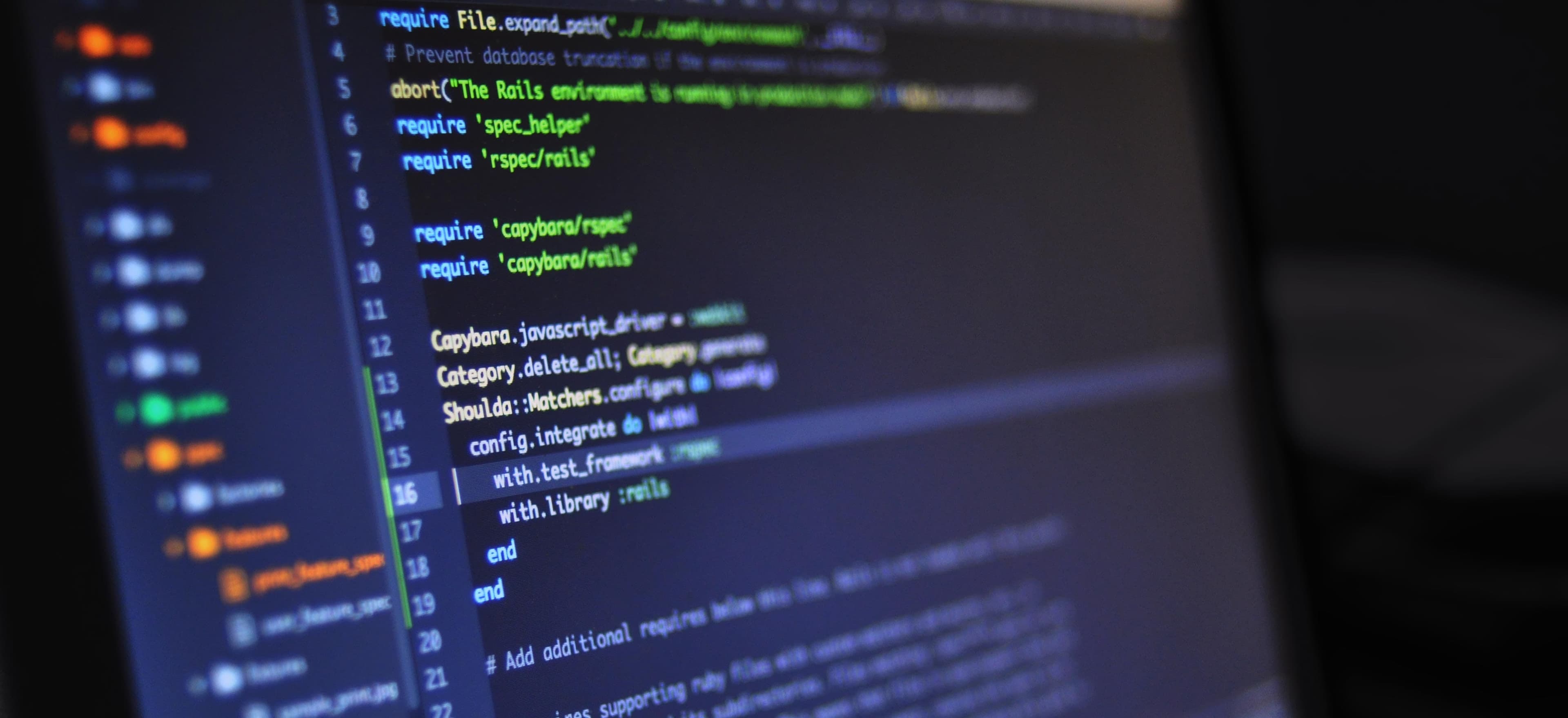
- Published on
Mastering Java's Math.pow: Recursive vs Iterative Pitfalls
When it comes to performing mathematical calculations in Java, one of the go-to functions is Math.pow()
. It allows developers to raise a number to a specific power, which will come in handy in various applications, from mathematical computations to algorithm implementations. However, as with many programming constructs, you might encounter pitfalls—especially when you delve into alternative ways of implementing this functionality. In this blog post, we will explore the usage of Math.pow
, compare recursive and iterative approaches, and identify common pitfalls.
Understanding Math.pow
First, let's clarify how the Math.pow()
function works. The syntax is straightforward:
double result = Math.pow(base, exponent);
Here is a simple example:
public class MathExample {
public static void main(String[] args) {
double base = 2;
double exponent = 3;
double result = Math.pow(base, exponent);
System.out.println("2 raised to the power of 3 is: " + result); // Output: 8.0
}
}
This method does the heavy lifting and provides a reliable result. However, it's crucial to understand when to use it versus writing your implementations.
Recursive Implementation
Before we present our recursive implementation, let's discuss what recursion is: a programming technique where a method calls itself to solve smaller subproblems until it reaches a base case.
Here’s a sample recursive method for calculating power:
public class PowerCalculator {
// Recursive method to calculate power
public static double recursivePower(double base, int exponent) {
// Base case: when exponent is 0
if (exponent == 0) {
return 1;
}
// Handling negative exponents
if (exponent < 0) {
return 1 / recursivePower(base, -exponent);
}
// Recursive step
return base * recursivePower(base, exponent - 1);
}
public static void main(String[] args) {
System.out.println("2 raised to the power of 3 is: " + recursivePower(2, 3)); // Output: 8.0
}
}
Why Use Recursion?
- Simplicity: The recursive method is easy to understand and aligns with mathematical definitions of exponentiation.
- Natural Decomposition: The problem is naturally divided into smaller problems, which can sometimes lead to more elegant code.
However, recursion is not without its pitfalls:
- Stack Overflow: If the
exponent
value is too large, the recursive calls can quickly consume the stack space, leading to aStackOverflowError
. - Performance: Each recursive call adds overhead. While this may not significantly impact small inputs, for large exponents, an iterative solution may be more efficient.
Iterative Implementation
Now that we’ve explored recursive methods, let’s turn to an iterative approach. This method employs a loop to calculate the power, generally resulting in better performance and no risk of stack overflow.
Here’s how an iterative calculation looks:
public class PowerCalculator {
// Iterative method to calculate power
public static double iterativePower(double base, int exponent) {
double result = 1;
int absExponent = Math.abs(exponent); // Handle negative cases
for (int i = 0; i < absExponent; i++) {
result *= base; // Multiply base absExponent times
}
// If the exponent is negative, invert the result
return (exponent < 0) ? 1 / result : result;
}
public static void main(String[] args) {
System.out.println("2 raised to the power of 3 is: " + iterativePower(2, 3)); // Output: 8.0
}
}
Why Use Iteration?
- Efficiency: The iterative method generally runs in O(n) time, with no additional overhead from recursive calls.
- Space Complexity: It uses O(1) space compared to recursive methods, which can use O(n) space.
Common Pitfalls in Both Approaches
Regardless of the approach you select, there are pitfalls to watch for:
-
Negative Exponents: Both implementations handle negative exponents, but it’s important to remember that with
Math.pow()
, the output is a floating-point number. This could lead to precision issues if you need highly accurate results. -
Non-integer Exponents: The recursive and iterative methods provided assume integer exponents. If you plan on extending functionality to non-integer exponents, you can run into precision issues. For more on floating-point arithmetic in Java, check this Java documentation.
-
Reusing Logic: Writing your methods is valuable for learning but consider using built-in functionality for production-grade code unless performance is a critical issue.
Lessons Learned
Both recursive and iterative methods for calculating powers in Java have their pros and cons. Ultimately, the best choice depends on the specific requirements of your project and the size of the input data.
When you need a quick, reliable solution, Math.pow()
is usually the best bet. However, if you're looking to understand algorithms deeper or need something tailored to specific conditions, you might explore custom implementations.
Feel free to explore further into these concepts with resources such as the Java API Documentation and additional algorithm design patterns. Happy coding!