Streamlining OAuth 2.0 Token Management in Spring Boot
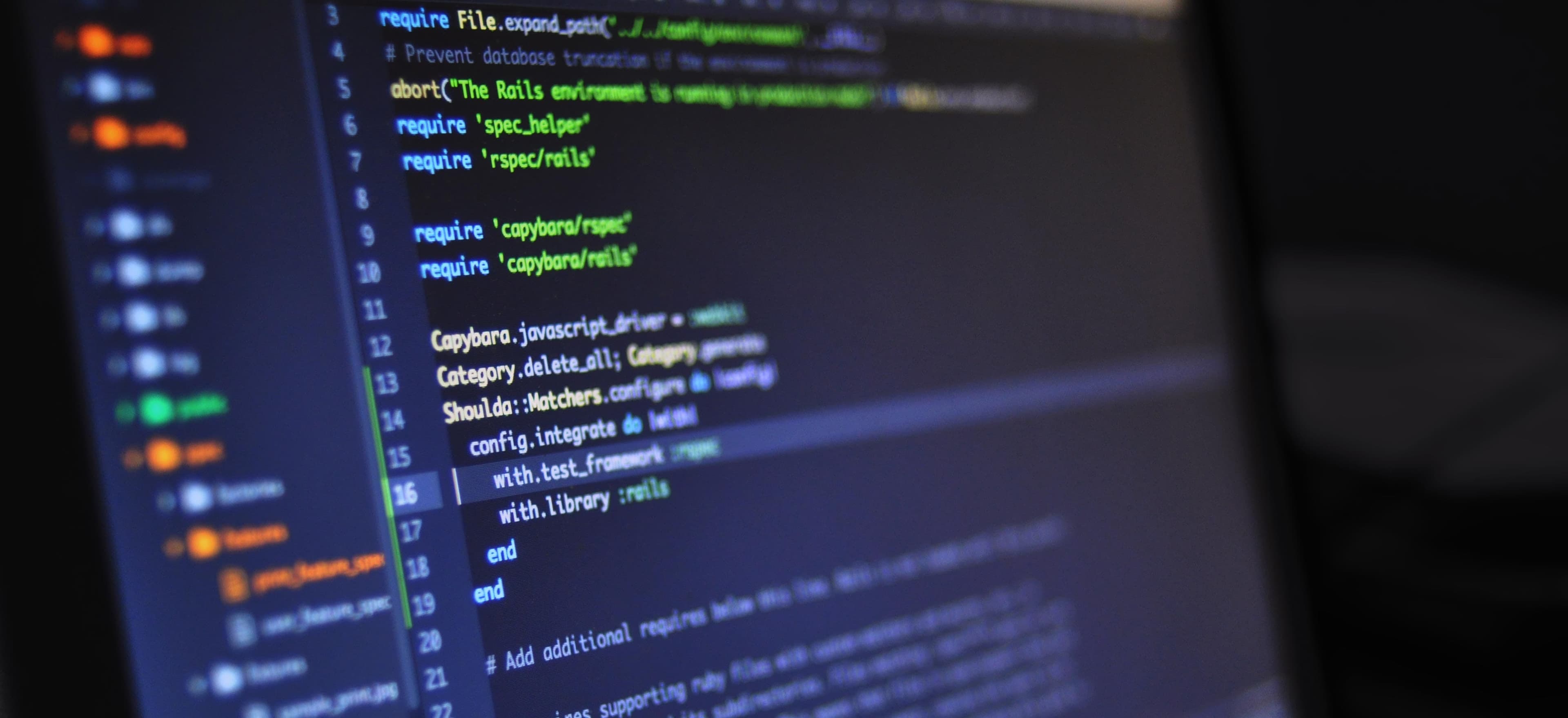
- Published on
Streamlining OAuth 2.0 Token Management in Spring Boot
OAuth 2.0 is the industry-standard protocol for authorization, allowing third-party services to exchange information without exposing user credentials. In a Spring Boot application, implementing OAuth 2.0 can seem daunting, especially when it comes to managing tokens efficiently. In this blog post, we will explore practical ways to streamline OAuth 2.0 token management, providing code snippets, clear explanations, and strategies for a seamless integration.
Table of Contents
- Understanding OAuth 2.0 Tokens
- Setting Up Spring Boot for OAuth 2.0
- Implementing Token Storage
- Refreshing Tokens
- Security Considerations
- Testing Your Implementation
- Conclusion
Understanding OAuth 2.0 Tokens
OAuth 2.0 tokens facilitate secure delegated access. There are two main types of tokens:
- Access Tokens: Short-lived tokens that allow access to resources.
- Refresh Tokens: Long-lived tokens used to request new access tokens without user intervention.
Understanding these tokens is crucial for implementing a resilient token management strategy. For an in-depth look at OAuth 2.0, you can refer to the official OAuth 2.0 specification.
Setting Up Spring Boot for OAuth 2.0
To get started, ensure your Spring Boot application has the necessary dependencies. The key dependency for OAuth 2.0 support is Spring Security.
Step 1: Add Dependencies
In your pom.xml
, include the following dependencies:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-client</artifactId>
</dependency>
Step 2: Configure Security Properties
In your application.yml
or application.properties
, configure your OAuth 2.0 client settings:
spring:
security:
oauth2:
client:
registration:
my-client:
client-id: YOUR_CLIENT_ID
client-secret: YOUR_CLIENT_SECRET
scope: read,write
authorization-grant-type: authorization_code
redirect-uri: "{baseUrl}/login/oauth2/code/{registrationId}"
provider:
my-provider:
authorization-uri: https://provider.com/oauth/authorize
token-uri: https://provider.com/oauth/token
user-info-uri: https://provider.com/oauth/userinfo
Why This Matters
By setting up these properties, Spring Security will handle many parts of token management automatically. This hands-off approach allows you to focus on implementing custom logic where necessary.
Implementing Token Storage
A common requirement for OAuth 2.0 configurations is effective token storage. You may consider storing tokens in a database or an in-memory repository, depending on your application's architecture and performance needs.
Example: In-Memory Token Management
We can create a custom token service to manage access and refresh tokens in memory:
import org.springframework.security.oauth2.core.OAuth2AccessToken;
import org.springframework.security.oauth2.core.OAuth2RefreshToken;
import org.springframework.stereotype.Service;
import java.util.HashMap;
import java.util.Map;
@Service
public class TokenStorageService {
private final Map<String, OAuth2AccessToken> accessTokenStore = new HashMap<>();
private final Map<String, OAuth2RefreshToken> refreshTokenStore = new HashMap<>();
public void storeAccessToken(String key, OAuth2AccessToken token) {
accessTokenStore.put(key, token);
}
public void storeRefreshToken(String key, OAuth2RefreshToken token) {
refreshTokenStore.put(key, token);
}
public OAuth2AccessToken retrieveAccessToken(String key) {
return accessTokenStore.get(key);
}
public OAuth2RefreshToken retrieveRefreshToken(String key) {
return refreshTokenStore.get(key);
}
}
Commentary
This service provides a simple interface for storing and retrieving both access and refresh tokens. The in-memory nature makes it suitable for testing and light-load applications, but consider a more persistent solution for production environments.
Refreshing Tokens
Tokens typically expire after a short period. To maintain user sessions without frequent logins, a refresh token mechanism is crucial. Below, we will create a method to refresh tokens when they expire:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.oauth2.client.OAuth2AuthorizedClient;
import org.springframework.security.oauth2.client.OAuth2AuthorizedClientManager;
import org.springframework.security.oauth2.client.OAuth2AuthorizedClientProvider;
import org.springframework.security.oauth2.core.OAuth2AccessToken;
@Service
public class TokenRefreshService {
@Autowired
private OAuth2AuthorizedClientManager authorizedClientManager;
public OAuth2AccessToken refreshAccessToken(OAuth2AuthorizedClient authorizedClient) {
OAuth2AuthorizedClientProvider provider = OAuth2AuthorizedClientProviderBuilder.builder()
.authorizationCode()
.refreshToken()
.build();
OAuth2AuthorizedClient newAuthorizedClient = authorizedClientManager.authorize(
OAuth2AuthorizeRequest.withClientRegistrationId(authorizedClient.getClientRegistrationId())
.principal(authorizedClient.getPrincipalName())
.build()
);
return newAuthorizedClient.getAccessToken();
}
}
Why Refresh Tokens?
By implementing a refresh token mechanism, we ensure a smoother user experience, preventing constant re-authentication. This is critical in applications with lengthy user sessions.
Security Considerations
With great power comes great responsibility. Storing OAuth tokens raises some security concerns, and here are a few strategies for mitigating risks:
- Use HTTPS: Always implement secure connections to prevent token interception.
- Secure Storage: For production, consider using a secure database or a vault service to store tokens.
- Token Expiry: Always set appropriate expiration times for access tokens and properly handle refresh tokens.
Additional tips can be found on the OWASP website, which offers guidelines on securing applications using OAuth 2.0.
Testing Your Implementation
Testing is essential to ensure your OAuth 2.0 setup functions correctly.
Unit Tests
You can write unit tests using JUnit to assert that tokens are stored and retrieved properly. Here’s a simple test for our TokenStorageService
:
import org.junit.jupiter.api.Test;
import org.springframework.security.oauth2.core.OAuth2AccessToken;
import static org.junit.jupiter.api.Assertions.assertEquals;
class TokenStorageServiceTest {
@Test
void testStoreAccessToken() {
TokenStorageService service = new TokenStorageService();
OAuth2AccessToken token = new OAuth2AccessToken(/* parameters */);
service.storeAccessToken("testKey", token);
assertEquals(token, service.retrieveAccessToken("testKey"));
}
}
Integration Tests
For a comprehensive verification, consider writing integration tests with a real authorization server using tools like Mockito or Testcontainers.
Lessons Learned
By following the strategies outlined in this post, you can implement efficient OAuth 2.0 token management in your Spring Boot applications. Understanding token dynamics and employing secure practices will enhance user experience while maintaining security. Dive deeper into Spring Security OAuth 2.0 documentation for more customization options and features.
With these insights, you're now equipped to build a solid foundation for OAuth 2.0 in Spring Boot. Happy coding!