Overcoming Common Pitfalls in Software Transactional Memory
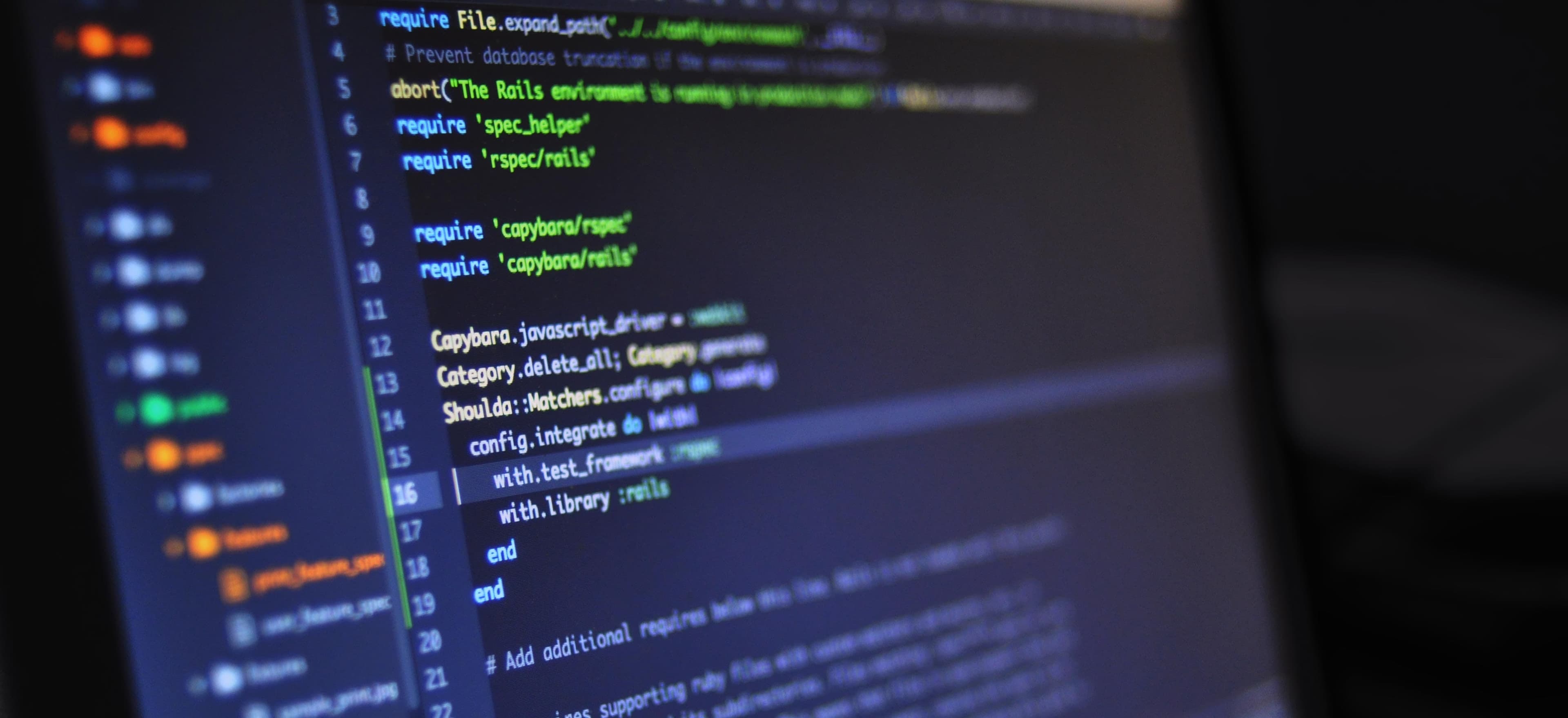
- Published on
Overcoming Common Pitfalls in Software Transactional Memory
Software Transactional Memory (STM) is an exciting paradigm that helps developers manage shared memory in concurrent applications. It allows blocks of code to execute in an atomic way, making it easier to write safe and correct multithreaded programs. However, like any technology, it comes with its challenges. In this post, we will discuss common pitfalls associated with STM and strategies to overcome them.
What is Software Transactional Memory?
Software Transactional Memory is a programming model that simplifies concurrent programming. It consists of blocks of code called transactions, which can run in isolation from each other. If a transaction completes successfully, all changes it made are visible. If something goes wrong, the system rolls back, ensuring a consistent state.
Key Benefits of STM
- Simplicity: Developers can think sequentially about their code without worrying about locks.
- Scoped Locks: Transactions can expand beyond local scope, which makes code easier to understand.
- Optimistic Concurrency: STM allows for optimistic concurrency control, where transactions assume success.
Common Pitfalls in Software Transactional Memory
While STM simplifies concurrency, several pitfalls can still hinder performance and reliability. Understanding these pitfalls is crucial for effective software design.
1. Overuse of STM
One of the most prevalent issues is using STM for every concurrent operation. Not all problems require the overhead of transactions.
Solution: Use STM selectively. For instance, encapsulate small, critical sections instead of wrapping entire algorithms in transactions. This can greatly reduce overhead.
// Bad practice: using STM for everything
atomic(() -> {
// Long computation here...
});
// Better practice: Only wrap critical sections
atomic(() -> {
// Only the critical section that modifies shared state
});
2. Lack of Fine-Grained Control
STM systems often provide a coarse granularity of locking, which can lead to unnecessary contention.
Solution: Break down large transactions into smaller ones when possible. This minimizes blocking and allows for greater concurrency.
// Larger transaction
atomic(() -> {
// Update account balance
// Update transaction logs
});
// Smaller transactions to reduce contention
atomic(() -> {
// Update account balance
});
atomic(() -> {
// Update transaction logs
});
3. Failure to Handle Rollbacks
Another common pitfall involves not adequately addressing rollbacks when transactions fail. Developers may assume a transaction will always succeed, leading to potential inconsistencies.
Solution: Always plan for failure. Define rollback behavior, and ensure your program can handle inconsistencies gracefully.
try {
// Wrap transaction in a try-catch
atomic(() -> {
// Update shared state
});
} catch (TransactionFailedException e) {
// Handle rollback or recovery logic
}
4. Inefficient Conflict Detection
STM implementations often require expensive checks for conflicts between concurrent transactions. If not managed efficiently, this can lead to significant performance hits.
Solution: Use lightweight conflict detection strategies. Depending on your use case, consider implementing optimistic updates or using versioned objects that can help minimize contention.
// Optimistic updates using versioning
class VersionedData {
private final AtomicInteger version = new AtomicInteger(0);
private int data;
public void updateData(int newData) {
int currentVersion = version.get();
int newVersion = currentVersion + 1;
// Optimistic update
if (version.compareAndSet(currentVersion, newVersion)) {
data = newData; // Perform update only if version is unchanged
}
}
}
5. Poorly Designed Interfaces
STM interfaces often focus on operations but may neglect the design of data structures involved. Improper design can introduce bugs or bottlenecks.
Solution: Design your data structures with concurrent modification in mind. Aim for immutable objects when possible, and provide safe, concurrent access to mutable states.
// Poorly designed mutable structure
class SharedList {
private List<Integer> list = new ArrayList<>();
public void add(int num) {
list.add(num); // Vulnerable to concurrent modification
}
}
// Improved immutable structure
class ImmutableList {
private final List<Integer> list;
public ImmutableList(List<Integer> initialList) {
this.list = new ArrayList<>(initialList);
}
public ImmutableList add(int num) {
List<Integer> newList = new ArrayList<>(list);
newList.add(num);
return new ImmutableList(newList);
}
}
6. Ignoring System and Domain Differences
Different systems and domains have varying concurrency requirements. Failing to adapt STM strategies accordingly can lead to poor performance.
Solution: Analyze your application and its concurrency patterns. Optimize your STM usage based on the specific needs of your domain, whether it's a high-frequency trading application or a web server.
To Wrap Things Up
Software Transactional Memory offers a compelling model for managing concurrency in programming. However, it is not without its challenges. By understanding common pitfalls such as overuse of STM, lack of fine-grained control, failure to handle rollbacks, inefficient conflict detection, badly designed interfaces, and ignoring contextual differences, developers can design better concurrent systems.
Key Takeaway: Selectively use STM, break down complexities, always account for rollbacks, optimize conflict detection, design for concurrency, and tailor strategies to your domain. This mindset will help you create efficient and robust applications.
For further reading, check out:
- The Transactional Memory Book
- Programming Concurrency on the JVM
By mastering these principles, you can overcome pitfalls and unlock the full potential of Software Transactional Memory in your applications. Happy coding!
Checkout our other articles