Troubleshooting MicroProfile Config Source Loading Issues
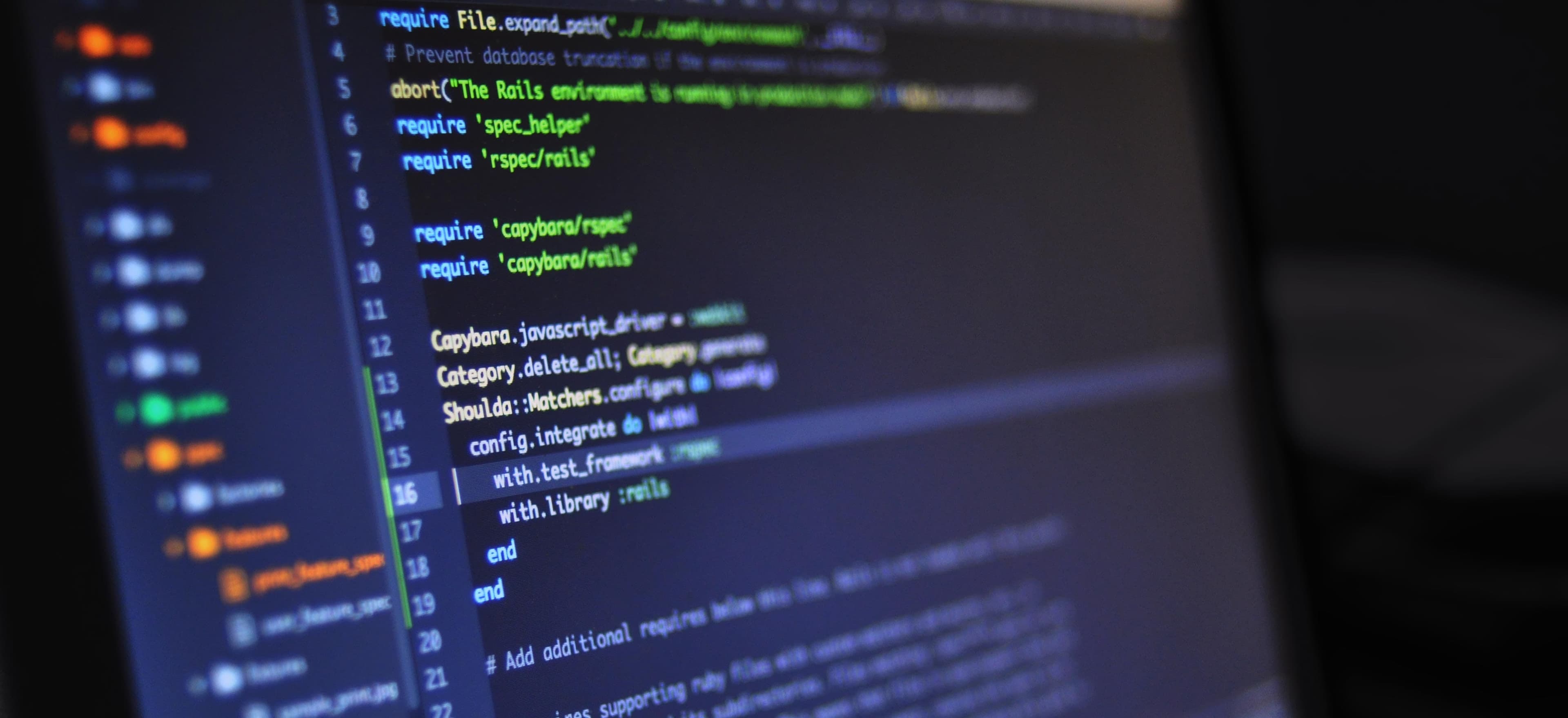
- Published on
Troubleshooting MicroProfile Config Source Loading Issues
MicroProfile offers a powerful framework for cloud-native Java applications by enhancing Java EE technologies with microservices-oriented capabilities. One pivotal feature of MicroProfile is its configuration management, which allows developers to externalize and centralize configuration properties. However, developers often encounter issues while loading these configuration sources. This blog post delves into troubleshooting common MicroProfile Config Source loading issues, provides clarifications, and details some solutions.
Understanding MicroProfile Config
Before we delve into troubleshooting, it’s important to understand how MicroProfile Config works. MicroProfile Config provides a way to read configuration from various sources (like environment variables, system properties, or even custom sources) in a standardized manner. The configuration is accessible through key-value pairs.
For instance, loading a configuration value can be done using:
import org.eclipse.microprofile.config.Config;
import org.eclipse.microprofile.config.ConfigProvider;
public class ConfigExample {
public static void main(String[] args) {
Config config = ConfigProvider.getConfig();
String myProperty = config.getValue("my.property", String.class);
System.out.println("Loaded Property: " + myProperty);
}
}
In this example, ConfigProvider.getConfig()
retrieves the configuration values, and getValue()
fetches a specific property.
Common Issues in Config Source Loading
Even with its robust design, you might run into various loading issues. Here are the most common ones:
- Property Not Found
- Incorrect Property Type
- Loading Order Conflicts
- Non-Standard Configuration Sources
- Environment-Specific Issues
Let’s take a deeper look at these issues.
1. Property Not Found
Issue Description: When configuration properties cannot be located, a default value may be returned or an exception may be thrown.
Solution: Ensure your configuration file (like application.properties
) is in the right location. If using an environment variable, confirm its existence.
Code Snippet:
String myProperty = config.getValue("MY_ENV_VAR", String.class);
if (myProperty == null) {
System.out.println("Error: MY_ENV_VAR not set.");
} else {
System.out.println("Loaded Property: " + myProperty);
}
In the above snippet, we are checking if the property is null
to avoid runtime issues.
2. Incorrect Property Type
Issue Description: If you request a property value as a different type than it is defined (e.g., expecting an Integer
but receiving a String
), an error may occur.
Solution: Always ensure the property type matches the expected return type.
Code Snippet:
try {
int myNumber = config.getValue("my.number", Integer.class);
System.out.println("Loaded Number: " + myNumber);
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
This snippet can catch potential exceptions and allow for graceful error handling.
3. Loading Order Conflicts
Issue Description: MicroProfile processes configuration sources in a particular order. If sources overlap, the last one wins, which may lead to unexpected configurations.
Solution: Make sure the order of your configuration sources is clear and intentional.
Loading Order Example
- System properties
- Environment variables
- Application properties files
- Custom config sources
To investigate the configuration loading order, you can check the service configuration in your application server or runtime.
4. Non-Standard Configuration Sources
Issue Description: Custom sources that are incorrectly implemented may cause loading failures.
Solution: If you have written a custom configuration source, ensure it correctly implements the required interfaces.
Code Snippet:
import org.eclipse.microprofile.config.ConfigSource;
import java.util.Map;
import java.util.HashMap;
public class MyCustomConfigSource implements ConfigSource {
private Map<String, String> properties = new HashMap<>();
public MyCustomConfigSource() {
properties.put("my.custom.property", "value");
}
@Override
public Map<String, String> getProperties() {
return properties;
}
// Implement the mandatory methods
@Override
public String getValue(String key) {
return properties.get(key);
}
@Override
public String getName() {
return "MyCustomConfigSource";
}
}
In this example, the custom configuration source is implementing ConfigSource
correctly. Make sure to register the custom source with your MicroProfile runtime.
5. Environment-Specific Issues
Issue Description: Configuration works perfectly in development but fails in production due to different environment setups.
Solution: Be aware of environment-specific variables, and ensure that your production environment mirrors expected configurations.
Perform thorough testing in environments that reflect your production setup as closely as possible. Utilize containerization technologies like Docker to ensure consistent environments.
Debugging Tools and Techniques
Debugging MicroProfile Config loading issues can be enhanced with the help of some tools and techniques:
- Logging: Implement logging in your configuration sources to track the loading process and catch potential issues.
- Environment Configuration Files: Validate that environment-specific configuration files contain the correct properties.
- Debugging in IDE: Utilize breakpoints and debugging in your IDE to inspect the configuration object at runtime.
Key Takeaways
MicroProfile provides developers with a robust way to handle configuration management, but issues will occasionally arise. By understanding common issues and strategies to troubleshoot them, you can effectively maintain your applications.
By following the practices outlined in this blog post, you can reduce the likelihood of running into configuration-related issues and ensure a smoother deployment experience. To learn more about MicroProfile, visit MicroProfile official documentation for additional insights.
By mastering MicroProfile Config, you pave the way towards building resilient cloud-native applications.
For further reading or support, check out community forums like the MicroProfile Community which can provide additional resources and help from experienced professionals. Happy coding!
Checkout our other articles