Customizing Simple Gradle Java Plugins: Common Pitfalls
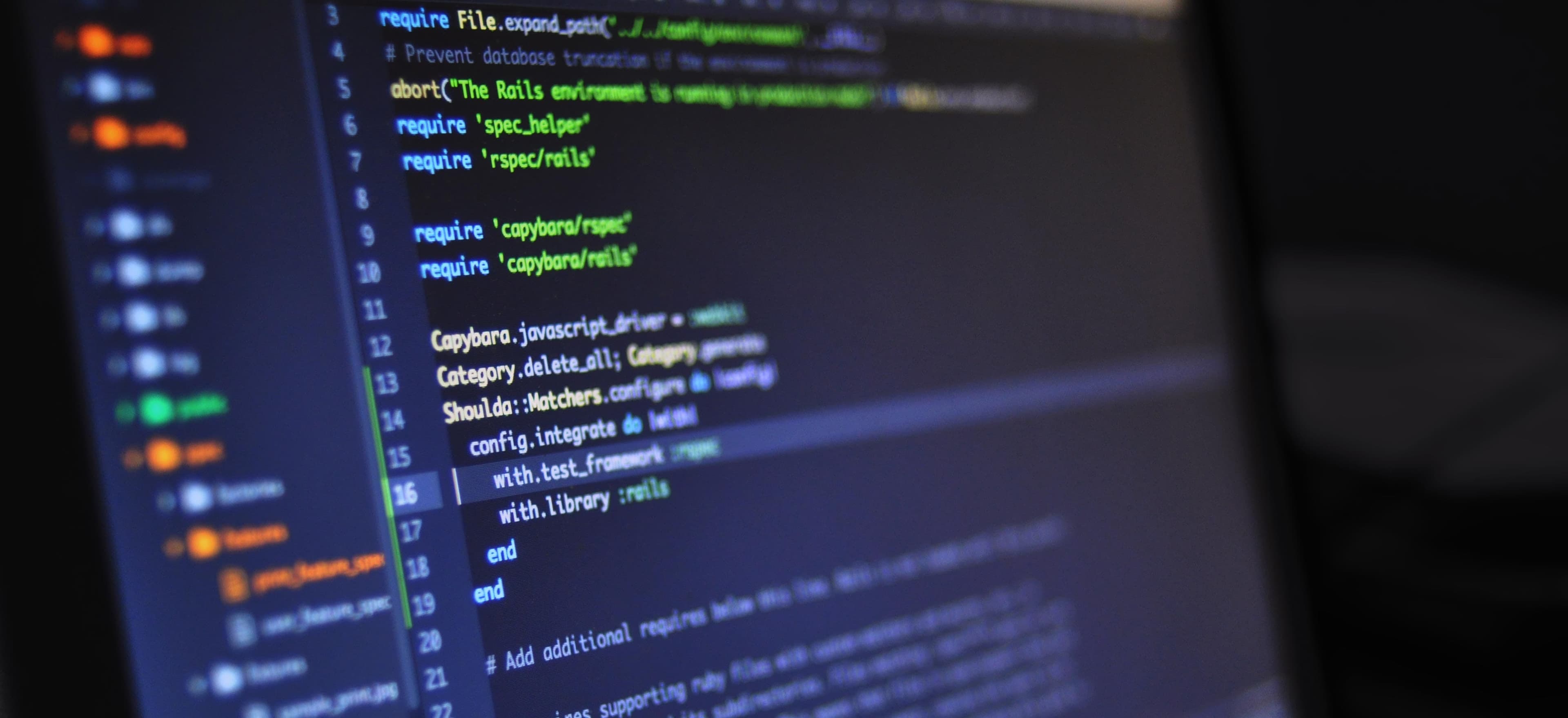
- Published on
Customizing Simple Gradle Java Plugins: Common Pitfalls
Gradle is one of the most popular build tools in the Java development ecosystem. Its flexibility and extensibility allow developers to create custom build solutions catering to their precise needs. However, when customizing Gradle Java plugins, developers often encounter pitfalls that can create hurdles in their project. In this blog post, we'll explore common mistakes and how to avoid them while enhancing your Gradle experience.
The Beauty of Gradle Customization
Gradle plugins simplify tasks and integrate third-party libraries effortlessly. When you begin customizing Gradle plugins, you can:
- Automate repetitive tasks.
- Enhance build scripts with new features.
- Tailor existing plugins to better suit your project's requirements.
Understanding how to do this correctly is essential for a seamless and productive experience.
Common Pitfalls When Customizing Gradle Java Plugins
Let's dive into the common pitfalls that developers face when customizing Java plugins in Gradle.
1. Not Understanding the Plugin Lifecycle
Why This Matters: One of the biggest mistakes developers make is jumping into scripting tasks without grasping the plugin lifecycle.
Gradle plugins are executed in specific phases:
- Initialization: Basic setup of the project model.
- Configuration: Configuration of projects, where the build script, dependencies, and tasks are evaluated.
- Execution: The build execution phase starts, running the configured tasks.
What to Do: Familiarize yourself with Gradle’s project lifecycle. This knowledge will help you correctly position your logic within the appropriate phase.
2. Ignoring Dependency Management
Why This Matters: Gradle’s dependency management system is powerful, but mistaking dependency configurations can lead to issues like version conflicts or unexpected behavior.
Common Issues to Watch Out For:
- Using the wrong configuration (e.g., compiling with
implementation
instead ofapi
). - Not understanding the difference between
compileOnly
andruntimeOnly
.
Best Practice: Use the appropriate dependency configuration to ensure that libraries are correctly included and scoped. For example:
dependencies {
implementation 'com.google.guava:guava:30.1.1-jre' // Available to the module
api 'org.apache.commons:commons-lang3:3.12.0' // Available to consumers
compileOnly 'junit:junit:4.13.2' // Only available at compile time
runtimeOnly 'mysql:mysql-connector-java:8.0.26' // Available only at runtime
}
3. Hardcoding Values
Why This Matters: Hardcoded values make your builds fragile and less portable, creating maintenance challenges.
What to Do: Always use project properties or configuration files instead of hardcoded values. For example:
Instead of this:
version = '1.0.0'
group = 'com.mycompany'
Use project properties:
version = project.hasProperty('version') ? project.version : '1.0.0'
group = project.hasProperty('group') ? project.group : 'com.mycompany'
This enables configurability, promoting flexibility across various environments.
4. Forgetting to Apply Plugins Correctly
Why This Matters: Misapplying plugins can result in build failures or unexpected behavior.
Common Mistakes:
- Applying plugins outside of the plugins block.
- Forgetting to apply the necessary Java plugin when creating a custom plugin.
Best Practice:
Always apply the Java plugin at the start of your build.gradle
file. Here is an example:
plugins {
id 'java'
id 'my.custom.plugin' version '1.0.0' // Always apply your custom plugin after the Java plugin
}
5. Overcomplicating the Build Script
Why This Matters: The power of Gradle lies in its simplicity. Overcomplicated scripts can lead to confusion and make maintenance challenging.
What to Do: Keep your build scripts clean and straightforward. Split complex logic into separate gradle files or use build script plugins. For example:
Instead of having lengthy logic in your main build file, create a separate file for specific tasks:
apply from: 'gradle/task.gradle'
6. Inefficient Task Configuration
Why This Matters: Gradle’s lazy configuration can significantly improve build times, but improper setup is a common misstep.
Best Practice: Use the doFirst
and doLast
closures to ensure that tasks are configured efficiently.
Example:
task myTask {
doFirst {
println 'Task is about to run...'
}
doLast {
println 'Task has finished executing!'
}
}
By configuring logic that only runs before or after tasks, you preserve resources and boost performance.
7. Failing to Manage Gradle’s Caching
Why This Matters: Gradle effectively caches outputs to speed up future builds, but not using it efficiently negates this advantage.
What to Do: Use the @CacheableTask
annotation in custom tasks where applicable. This improves build time by caching task outputs.
Example of usage:
import org.gradle.api.tasks.CacheableTask
@CacheableTask
class MyCustomTask extends DefaultTask {
@TaskAction
void performTask() {
println 'This task can be cached'
}
}
8. Bad Communication with External Libraries
Why This Matters: Improper configurations or misunderstandings of how an external library integrates with your project can result in build failures.
What to Do: Always consult the documentation of the libraries you’re using to understand how they expect to be configured and included in your build.
The Bottom Line
Customizing Gradle Java plugins can unleash a new level of productivity in your project. By being aware of common pitfalls, you can create efficient and maintainable build scripts that harness the full potential of Gradle.
For continued learning, consider exploring the Gradle documentation for best practices and fundamental concepts.
By adhering to these guidelines and adopting a mindful approach to customization, you can avoid common missteps and enjoy a smooth and effective Gradle experience in your Java projects.
Happy building!
Checkout our other articles