Troubleshooting TestNG Integration Issues in NetBeans 7.2
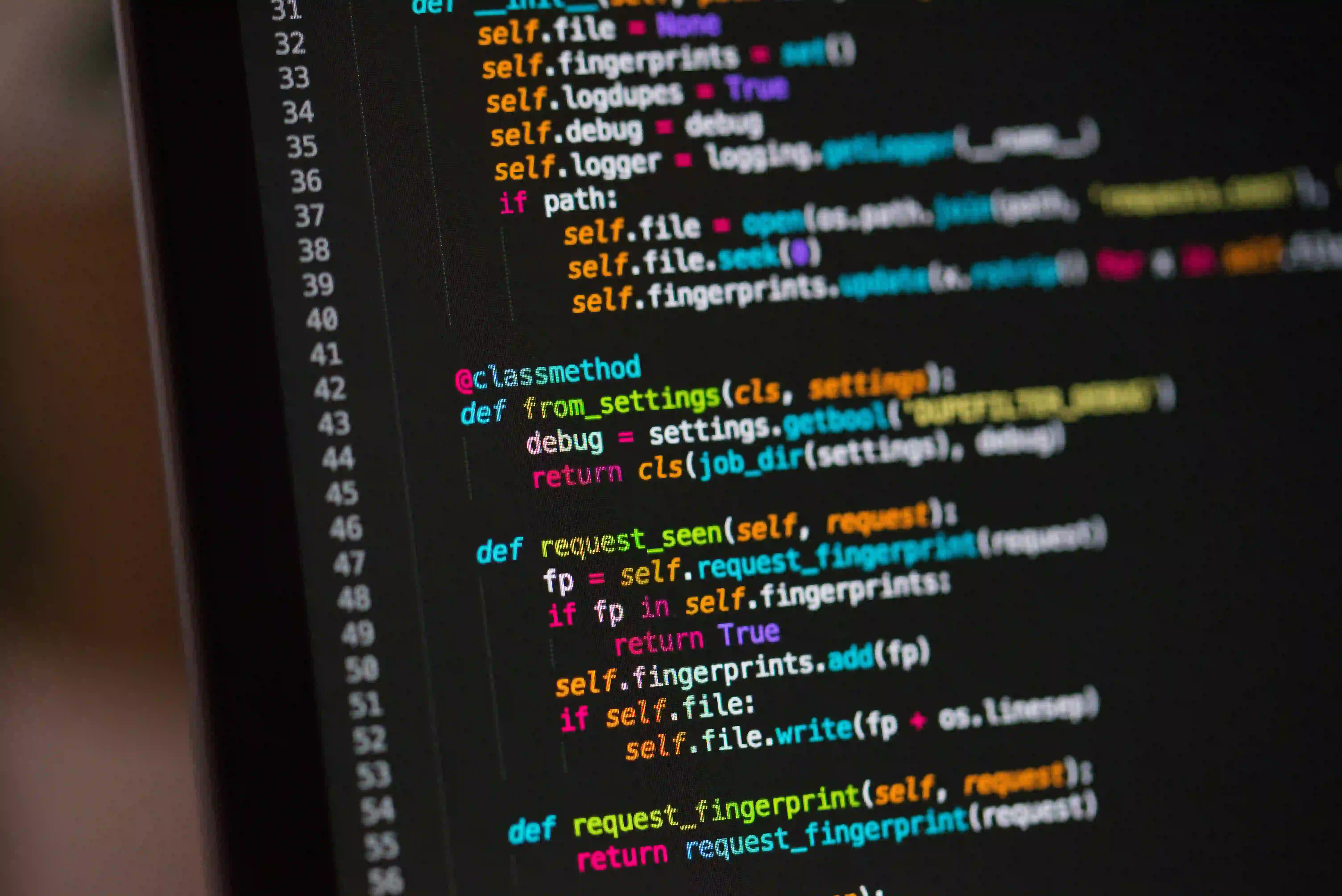
Troubleshooting TestNG Integration Issues in NetBeans 7.2
As Java developers, we often rely on frameworks to streamline our testing processes. TestNG is one such powerful testing framework that offers a plethora of features for a better testing experience. However, integrating TestNG into the NetBeans IDE, particularly in version 7.2, can sometimes pose challenges. This blog post will discuss common integration issues and provide solutions to troubleshoot them effectively.
Understanding TestNG
TestNG, which stands for "Test Next Generation," is a testing framework inspired by JUnit and NUnit, but introduces new functionalities that make it more powerful and flexible. Features such as annotations, flexible test configurations, and data-driven testing are integral to TestNG, making it a preferred choice for many developers.
For more information about TestNG and its features, you can visit the official TestNG documentation.
Setting Up TestNG in NetBeans 7.2
Before diving into troubleshooting integration issues, it's essential to understand how to set up TestNG in NetBeans 7.2 properly:
-
Install NetBeans 7.2 - Ensure you have the IDE installed on your machine.
-
Add the TestNG Library - Download the TestNG JAR file from the TestNG download page. You can also add it via Maven if you are using a Maven project.
-
Configure Libraries:
- Navigate to the project properties (right-click on your project > Properties).
- Go to the "Libraries" menu, click "Add JAR/Folder," and select the downloaded TestNG JAR.
-
Create a Test Class: Here’s a sample TestNG test class:
☕snippet.javaimport org.testng.annotations.Test; public class SampleTest { @Test public void testMethod() { System.out.println("Hello, TestNG!"); assert true; } }
-
Run the Test - Right-click on the class and choose "Test File" to see the results.
Common Integration Problems
Despite following the setup instructions, developers occasionally run into issues. Here are some of the most common problems and how to resolve them.
1. TestNG Not Recognizing Annotations
Problem: TestNG annotations like @Test are not recognized by the IDE.
Solution: This typically occurs due to:
- Missing TestNG library in the project.
- Incorrectly configured project.
Make sure you have added the TestNG JAR file to the project libraries correctly. Review the libraries configured in your project properties and add the TestNG JAR if necessary.
import org.testng.annotations.Test;
public class MyTest {
@Test
public void verifyCalculation() {
int result = 2 + 2;
assert result == 4;
}
}
After verifying that the libraries are included, try refreshing or restarting NetBeans to ensure the IDE picks up the changes.
2. Class Not Found Errors
Problem: When running the tests, you encounter "class not found" errors.
Solution:
- Ensure that your test class is in the correct directory. In a typical Maven project structure, test classes should reside in the
src/test/java
folder. - Check the classpath configuration to make sure the test classes are appropriately compiled.
To fix this issue, you can compile using the following command from the terminal within your project directory:
mvn clean test
3. Configuration XML Errors
Problem: Issues arise from a poorly configured testng.xml
file.
Solution: If you are using a testng.xml
file to define your test suite, ensure that it is correctly structured. Below is a simple example:
<!DOCTYPE suite SYSTEM "https://testng.org/testng-1.0.dtd">
<suite name="Suite1">
<test name="Test1">
<classes>
<class name="com.example.MyTest"/>
</classes>
</test>
</suite>
Key Points:
- Ensure the class names are fully qualified with the appropriate package names.
- Any typo in the XML can lead to configuration issues.
To validate if your suite file is working correctly, run it via the IDE or directly from the command line:
java -cp "lib/*" org.testng.TestNG testng.xml
4. Build Path Issues
Problem: The IDE throws an error stating that TestNG cannot be found within the build path.
Solution: This can often happen due to an incorrect build configuration. Check the following:
- Navigate to Project Properties > Build > Compile.
- Ensure that the TestNG library is listed under the Compile Classpath.
If issues persist, consider cleaning the project:
- Right-click on the project.
- Select "Clean and Build."
Cleaning the build can often resolve path-related issues.
Best Practices for Using TestNG
To enhance your testing productivity with TestNG, consider applying the following best practices:
Use Annotations Wisely
Annotations drive the lifecycle of your tests in TestNG. Familiarize yourself with annotations like @BeforeMethod
, @AfterMethod
, @BeforeClass
, and @AfterClass
to set up and tear down tests effectively.
Group Your Tests
Organizing tests into groups can make managing large test suites easier. Use the groups
attribute in your test methods to categorize tests.
@Test(groups = {"smoke"})
public void mySmokeTest() {
// test code
}
Data-Driven Testing
Take advantage of TestNG's data provider feature for data-driven testing. This allows you to run the same test with different input values by defining a data provider method.
@DataProvider(name = "dataProvider")
public Object[][] dataProviderMethod() {
return new Object[][]{ {1, 2}, {3, 4} };
}
@Test(dataProvider = "dataProvider")
public void additionTest(int a, int b) {
assertEquals(a + b, a + b);
}
Refer to the Documentation
For extensive features and functionalities, always keep TestNG’s documentation open. It is a comprehensive resource for all things related to TestNG.
A Final Look
Integrating TestNG into NetBeans 7.2 can be straightforward if approached with a systematic mindset. However, problems can arise at any step of the way. With the guidelines and troubleshooting tips provided in this article, you should be able to resolve common issues seamlessly.
Remember that a structured testing approach, combined with a solid understanding of TestNG features, lays the foundation for a robust testing suite that enhances code quality and reliability. Happy testing!