Optimize Web App Speed: Tackling Data URI Pitfalls
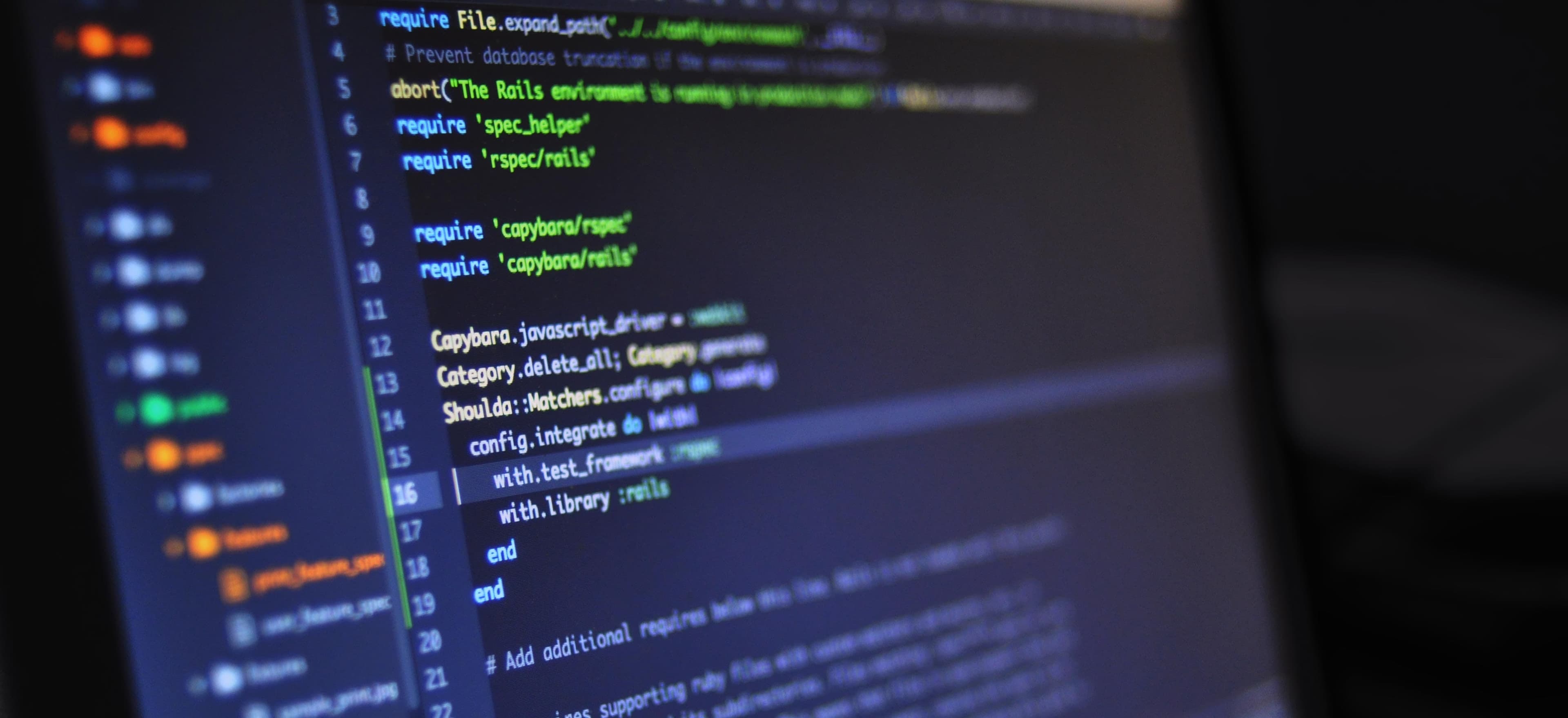
- Published on
Optimize Web App Speed: Tackling Data URI Pitfalls
In the modern digital landscape, web applications must deliver seamless experience to users. One of the key performance metrics is speed. A crucial aspect that can affect your web app's speed is how you manage resources—particularly images and other media. One strategy often employed is the use of Data URIs. While they can offer benefits, misusing Data URIs can also lead to significant pitfalls. In this post, we will explore data URIs, their benefits, potential pitfalls, and best practices to optimize your web application speed.
What is a Data URI?
A Data URI is a base64 encoded representation of a file (like an image or video) that can be embedded directly into your HTML or CSS. Instead of linking to an external file, you include the file's content inline.
For example, here's how an image might be represented as a Data URI in HTML:
<img src="data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAJYAAAB...">
Advantages of Using Data URIs
-
Reduction in HTTP Requests: By embedding files directly, you can lower the number of HTTP requests a browser has to make. This is especially beneficial if your web app uses a large number of small images.
-
Faster Load Times: Since the resources are included within the HTML/CSS, the browser does not have to wait for additional network requests, leading to improved load times.
-
Self-containment: Having resources embedded makes certain assets self-contained with your documents, which can simplify deployments and reduce dependency on external files.
Potential Pitfalls
While Data URIs can contribute to speeding up your web app, they can also lead to performance issues if not managed properly. Let’s discuss some of these pitfalls.
1. Increased File Size
Data URIs can bloat your HTML or CSS files significantly. This happens because base64 encoding increases the size of the original file by about 33%. If you use Data URIs for large images or a significant number of files, the overall size of the HTML or CSS would increase drastically.
Consider this example of embedding an image directly in CSS:
.background {
background-image: url(data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAA...);
}
While this approach reduces the number of HTTP requests, it can result in a larger CSS file.
2. Cache Inefficiency
When using Data URIs, you lose the benefit of browser caching. Typically, when an image is loaded, it is cached so that subsequent requests for the same image can be served from the cache rather than having to load the file again. With Data URIs, forcing repeated data transmission for every request means the same image is re-processed every time it is called. This can lead to unnecessary data transfer and slower performance in certain scenarios.
3. Compilation Overhead
When large Data URIs are embedded in style sheets, the initial loading time increases because the browser has to compile these styles. It can also complicate Minification processes, leading to longer build times.
Best Practices for Using Data URIs
-
Limit the Size of Embedded Resources: Use Data URIs for small images or icons, typically those less than 10 KB in size. Larger images should remain as external resources to prevent file size increases and caching inefficiencies.
-
Utilize Sprite Images: For icons or small graphics, consider using image sprites. This involves combining multiple images into a single one and applying CSS background positions to display the required segment. This approach reduces the number of on-the-fly requests while keeping file sizes manageable.
-
Leverage Efficient Encoding: Use tools to optimize images before converting them to Data URIs. Websites like TinyPNG can help you compress images significantly, thus keeping the overall file size minimal.
-
Hybrid Approach: Use a mixture of Data URIs for small items and traditional file links for larger assets. This approach maximizes the benefits of both methods.
-
Monitor Performance: Use tools such as Google PageSpeed Insights to analyze your web app's speed and assess whether your use of Data URIs is helpful or harmful.
How to Convert Files to Data URIs
To use a Data URI, you can easily convert files using online converters or command-line tools. Here's an example of how you might do this using Node.js:
const fs = require('fs');
function convertToDataURI(filePath) {
const image = fs.readFileSync(filePath);
const base64 = image.toString('base64');
const mime = 'image/png' // Change it according to the file type
return `data:${mime};base64,${base64}`;
}
console.log(convertToDataURI('path/to/image.png'));
Closing the Chapter
Optimizing web app speed is an ongoing challenge. Data URIs can be both a boon and a bane. When used appropriately, they can reduce the number of HTTP requests and improve load times. However, the pitfalls associated with their misuse, such as increased file sizes and inefficiencies in caching, can degrade your application's performance.
For best results, consider a well-balanced approach: leverage Data URIs for small assets while keeping larger files external. Continuously monitor performance and incorporate optimization techniques to ensure that your web application remains fast and user-friendly.
For more detailed tips on web optimization, feel free to explore the following resources:
- Web Performance Optimization: The Ultimate Guide
- Understanding Data URIs in Web Applications
With a careful strategy, your web application can achieve peak performance, ensuring a faster and more efficient user experience.