Common Pitfalls in JAX-RS and Jetty Web Service Setup
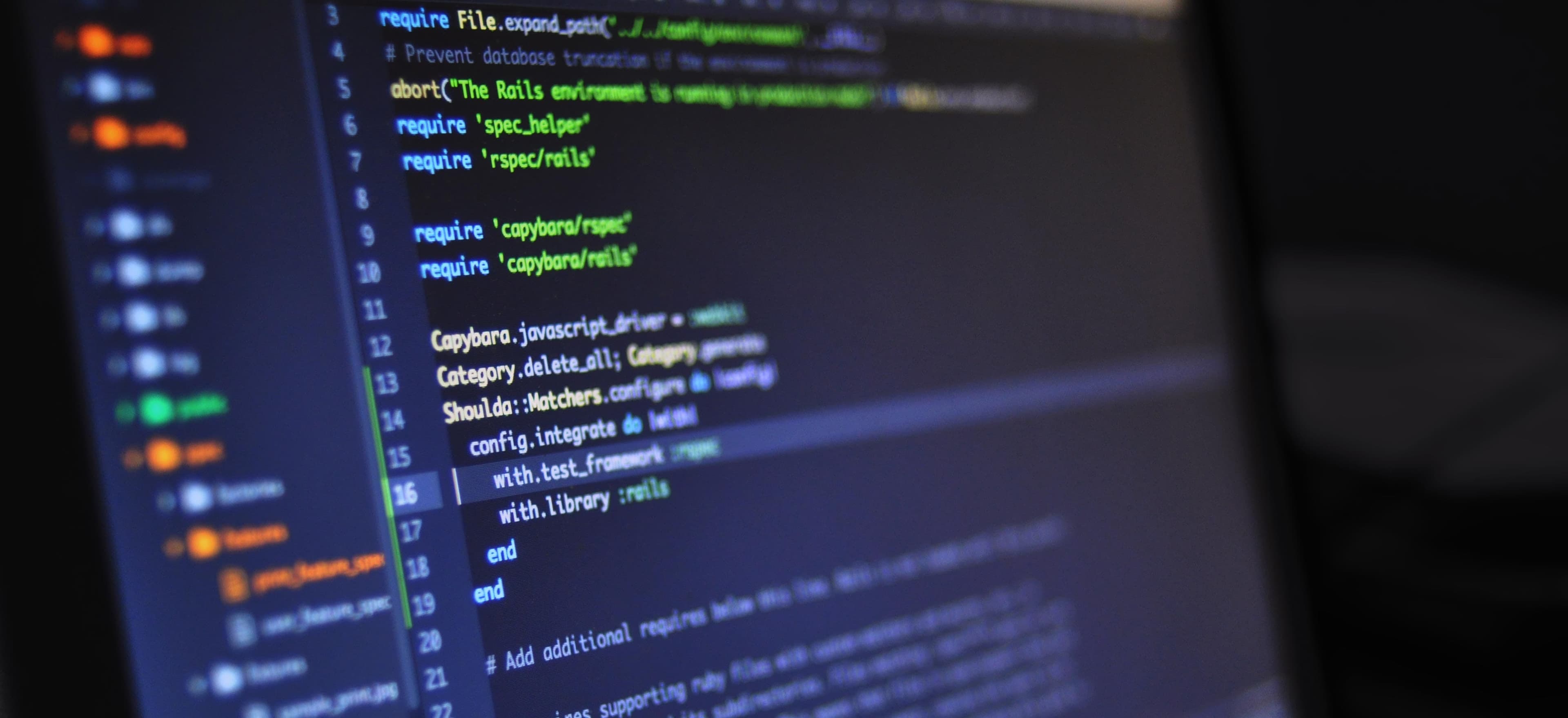
- Published on
Common Pitfalls in JAX-RS and Jetty Web Service Setup
When building web services in Java using JAX-RS (Java API for RESTful Web Services) alongside Jetty, developers often experience various challenges. While both technologies offer power and flexibility, there are common pitfalls to be aware of. In this blog post, we’ll discuss these pitfalls and provide you with best practices and code snippets to help you successfully set up JAX-RS with Jetty.
What is JAX-RS?
JAX-RS is a set of APIs to create RESTful web services in Java. It provides annotations that simplify interaction with HTTP requests, making it easier to build scalable and flexible services.
What is Jetty?
Jetty is a lightweight, highly scalable Java-based HTTP server and servlet container. It is particularly known for its embedded web server capabilities, making it suitable for microservices.
Common Pitfalls in JAX-RS and Jetty Setup
1. Incorrect Dependency Configuration
One of the most frequently encountered issues involves misconfigured dependencies. JAX-RS and Jetty require specific libraries that, if not included correctly, can lead to runtime errors.
For example, ensure you have the right Maven dependencies. Here is an example of a pom.xml
file:
<dependencies>
<dependency>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-server</artifactId>
<version>11.0.0</version>
</dependency>
<dependency>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-servlet</artifactId>
<version>11.0.0</version>
</dependency>
<dependency>
<groupId>javax.ws.rs</groupId>
<artifactId>javax.ws.rs-api</artifactId>
<version>2.1</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<version>2.34</version>
</dependency>
</dependencies>
Why is this important?
Using incompatible or outdated versions can lead to issues like NoClassDefFoundError, which indicates that certain classes cannot be found at runtime. Always check for version compatibility and updates.
2. Failure to Set Up Resource Scanning
Another common issue is not properly configuring your resource classes for JAX-RS discovery. By default, JAX-RS scans packages for resources, but it's essential to clarify which packages should be scanned.
A common configuration for Jetty can look like this:
import org.eclipse.jetty.server.Handler;
import org.eclipse.jetty.server.Server;
import org.eclipse.jetty.servlet.ServletContextHandler;
import javax.ws.rs.core.Application;
public class Main {
public static void main(String[] args) throws Exception {
Server server = new Server(8080);
ServletContextHandler context = new ServletContextHandler(ServletContextHandler.SESSIONS);
context.setContextPath("/");
server.setHandler(context);
context.addServlet(org.glassfish.jersey.servlet.ServletContainer.class, "/*")
.setInitParameter("javax.ws.rs.Application", MyApplication.class.getCanonicalName());
server.start();
server.join();
}
public static class MyApplication extends Application {
@Override
public Set<Class<?>> getClasses() {
Set<Class<?>> resources = new HashSet<>();
resources.add(MyResource.class); // Add your resource classes here
return resources;
}
}
}
Why is this crucial?
If the resources are not correctly scanned, your services will be unreachable, leading to 404 errors. Explicitly defining your resource classes helps ensure that your application can properly locate and register them.
3. Ignoring Cross-Origin Resource Sharing (CORS)
CORS is vital for web applications, especially when your front-end and back-end are hosted on different origins. Ignoring CORS can lead to security errors that inhibit client-side API calls.
To enable CORS in JAX-RS, you can create a filter like this:
import javax.ws.rs.container.ContainerResponseFilter;
import javax.ws.rs.container.ContainerRequestContext;
import javax.ws.rs.container.ContainerResponseContext;
import javax.ws.rs.ext.Provider;
import java.io.IOException;
@Provider
public class CORSFilter implements ContainerResponseFilter {
@Override
public void filter(ContainerRequestContext requestContext, ContainerResponseContext responseContext) throws IOException {
responseContext.getHeaders().add("Access-Control-Allow-Origin", "*"); // Set to specific domains in production
responseContext.getHeaders().add("Access-Control-Allow-Headers", "origin, content-type, accept, authorization");
responseContext.getHeaders().add("Access-Control-Allow-Credentials", "true");
responseContext.getHeaders().add("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE, OPTIONS, HEAD");
}
}
Why is this important?
CORS avoids scenarios where your application could be misused via cross-origin requests. Proper configuration enhances security and user experience.
4. Not Handling Exceptions Gracefully
Unhandled exceptions can lead to your application crashing or returning meaningless error messages. Utilizing exception mappers in JAX-RS is essential for handling and logging errors gracefully.
Here’s an example:
import javax.ws.rs.WebApplicationException;
import javax.ws.rs.core.Response;
import javax.ws.rs.ext.ExceptionMapper;
import javax.ws.rs.ext.Provider;
@Provider
public class MyExceptionMapper implements ExceptionMapper<Throwable> {
@Override
public Response toResponse(Throwable exception) {
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity("Something went wrong: " + exception.getMessage())
.type("text/plain")
.build();
}
}
Why is this necessary?
A good API should provide meaningful error messages. Properly handled exceptions inform developers and clients about what went wrong, making maintenance easier.
5. Configuration and Server Lifecycle Management
Finally, managing the lifecycle of your Jetty server and its configurations is crucial. Often developers overlook starting, stopping, or restarting the server correctly, leading to resource leaks or hanging processes.
Runtime.getRuntime().addShutdownHook(new Thread(() -> {
try {
server.stop();
} catch (Exception e) {
e.printStackTrace();
}
}));
Why does this matter?
Proper lifecycle management ensures that resources are released appropriately, thus maintaining the stability of your application.
Closing the Chapter
Setting up a JAX-RS web service with Jetty can be a rewarding experience, but it's important to avoid common pitfalls. By ensuring proper dependency management, resource scanning, CORS configuration, exception handling, and server lifecycle management, you can build robust, maintainable RESTful services.
If you're interested in diving deeper into JAX-RS, consider checking out the official JAX-RS documentation or Jetty's documentation.
Taking these points into consideration, you'll be well-equipped to tackle web service setups using JAX-RS and Jetty effectively! Happy coding!
Checkout our other articles