Is System.getProperty() Slowing Down Your Java Application?
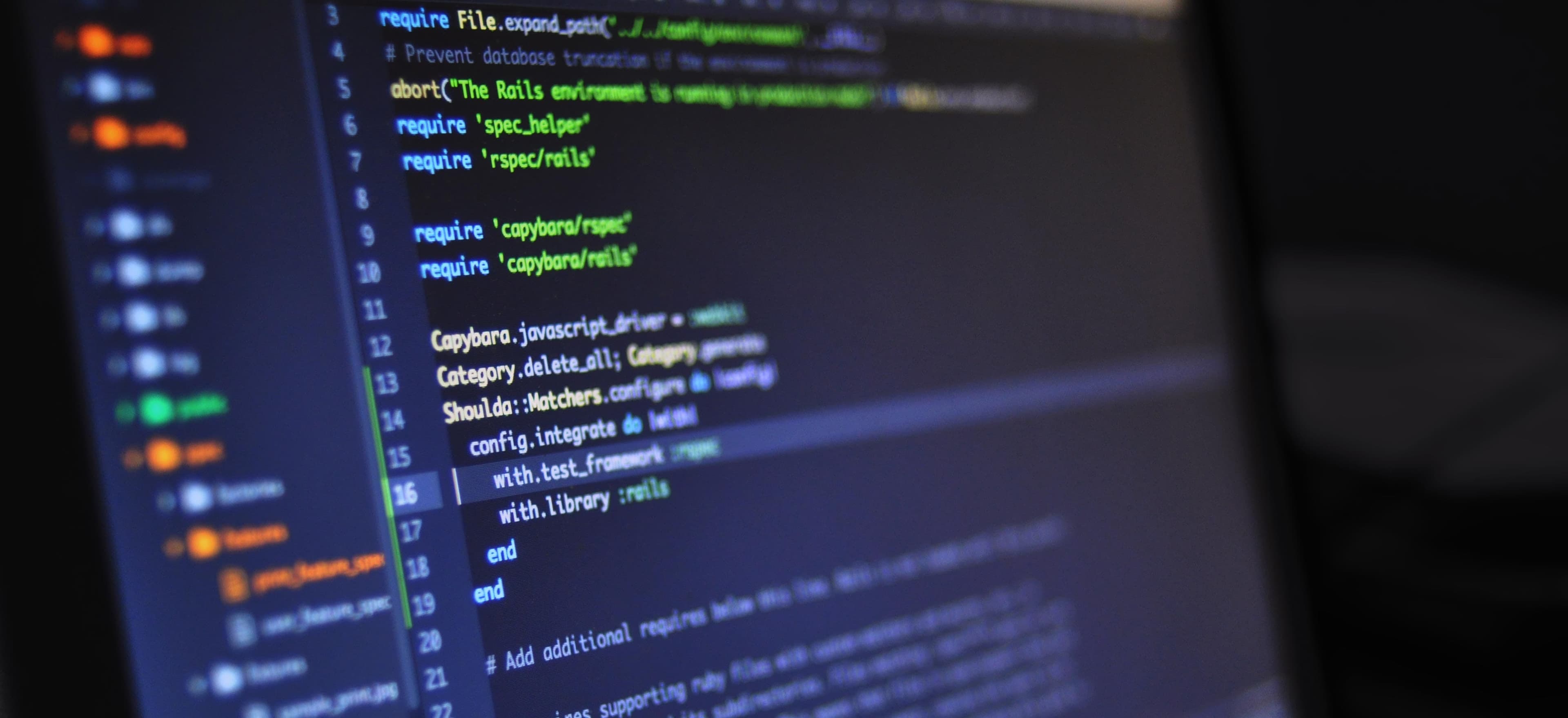
- Published on
Is System.getProperty() Slowing Down Your Java Application?
The performance of a Java application is crucial, especially when it operates in a high-load environment. One often overlooked aspect that can affect performance is the use of System.getProperty()
. In this blog post, we will dive deep into this method, explore potential performance impacts, and provide some strategies for optimizing its use.
What is System.getProperty()?
In Java, System.getProperty(String key)
is a method used to retrieve the value of a system property. System properties can provide information about the environment your application runs in, such as the Java version, user home directory, or operating system type.
String osName = System.getProperty("os.name");
System.out.println("Operating System: " + osName);
In the code snippet above, we retrieve the name of the operating system using the system property os.name
.
Why Use System.getProperty()?
Before we dive into performance, let's talk about the why of using System.getProperty()
. It provides a straightforward way to access system configurations without hardcoding values, making your application more flexible and portable.
Common Use Cases
- Configuration: Dynamically retrieve settings based on the system environment.
- Logging: Capture information for troubleshooting.
- Feature Flags: Control the availability of certain features based on the environment.
How System.getProperty() Can Impact Performance
While System.getProperty()
has its uses, frequent calls to it can lead to performance bottlenecks, particularly in tight loops or time-sensitive code.
The Performance Cost
- Synchronization Overhead: The method retrieves properties from a synchronized map. This synchronization may introduce a slight delay, especially when called many times.
- Repeated Access: Each call to
System.getProperty()
involves a lookup in a Hashtable-like structure. If you are calling this method multiple times, the cumulative cost becomes significant.
Example of Inefficiency
Consider the following example that repeatedly accesses system properties in a loop:
for (int i = 0; i < 1000; i++) {
String userHome = System.getProperty("user.home");
// Perform some operation using userHome
}
In this case, the System.getProperty()
method is called 1000 times unnecessarily. A better approach would involve retrieving the property once and storing it in a variable:
String userHome = System.getProperty("user.home");
for (int i = 0; i < 1000; i++) {
// Use userHome for operations
}
Optimization Strategies
-
Cache Properties: Retrieve the property once and store it in a local variable.
String userHome = System.getProperty("user.home"); // Use userHome throughout your method
-
Utilize Static Final Constants: If a property is static and known at compile time, define it as a constant.
public static final String USER_HOME = System.getProperty("user.home");
-
Profile Your Application: Use tools like VisualVM or Java Flight Recorder to analyze and identify performance bottlenecks.
-
Limit the Scope: Ensure that properties are fetched only when necessary. Don't retrieve them more than once if the value doesn't change during runtime.
When to Use System.getProperty() Responsibly
Despite its downsides, there are scenarios where using System.getProperty()
is justified:
- Initial Configuration: When your application first initializes, a small number of properties can be fetched.
- Non-Performance Critical Sections: If the method is called infrequently and does not affect core functionality, it may be acceptable to use it.
Final Thoughts
While System.getProperty()
is a powerful tool for accessing system information, it can also lead to performance issues if not used wisely. Caching its values and consciously minimizing calls can lead to noticeable improvements in application performance. By following the best practices outlined in this post, you can harness the benefits of System.getProperty()
without falling into performance pitfalls.
For further insights into Java performance optimization, consider checking out articles on Java Performance Tuning and Java Memory Management.
Happy coding!
Checkout our other articles