Common Pitfalls When Virtualizing Java Applications
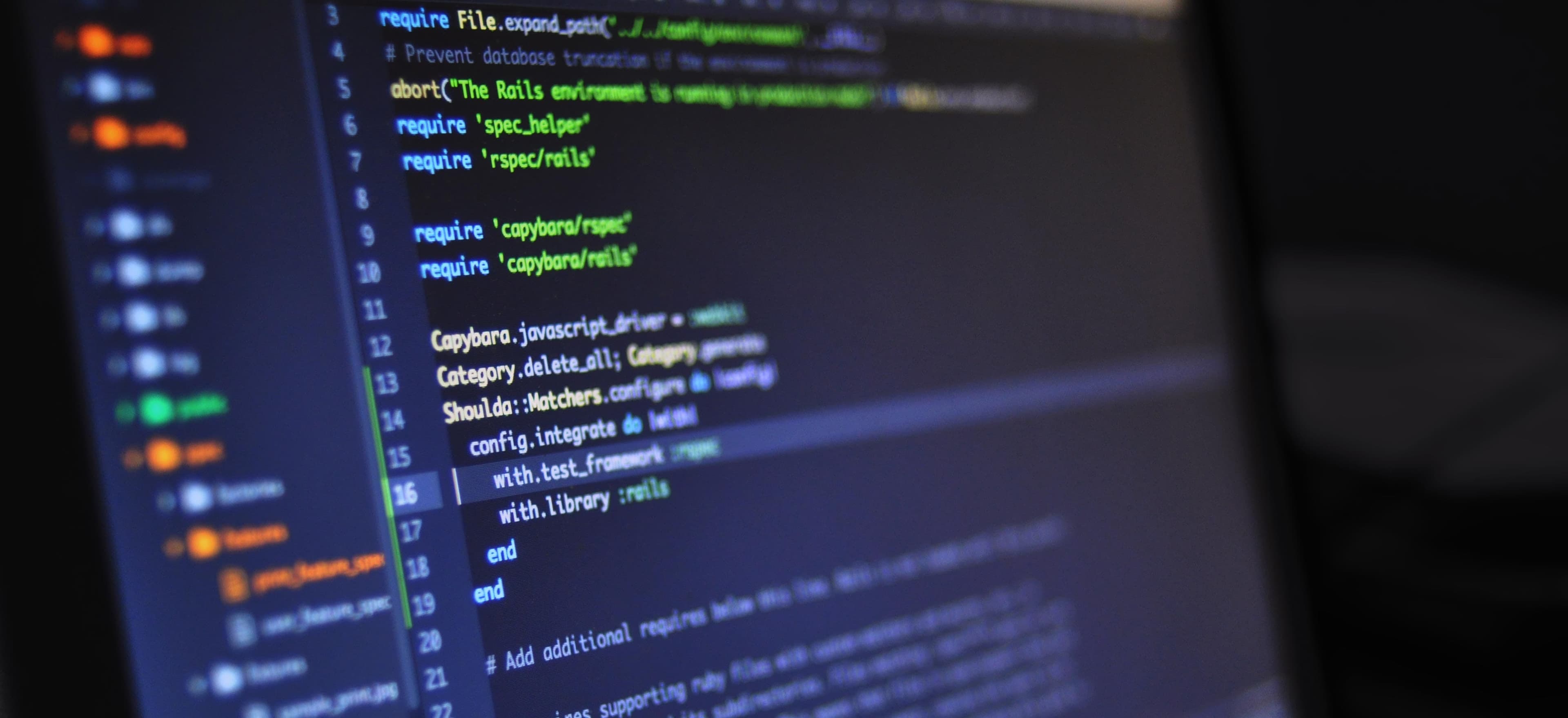
- Published on
Common Pitfalls When Virtualizing Java Applications
In recent years, virtualization has emerged as a cornerstone for deploying applications in cloud environments and managing resources effectively. When it comes to Java applications, virtualization can lead to significant performance improvements and facilitate easier management. However, developers often encounter various pitfalls that can hamper these benefits. In this blog post, we'll explore common challenges faced when virtualizing Java applications and how to avoid them effectively.
The Advantages of Virtualizing Java Applications
Before diving into the pitfalls, it's essential to understand why virtualization is beneficial:
- Scalability: Easily scale applications based on demand without overhauling infrastructure.
- Isolation: Run multiple Java applications in separate virtual instances to eliminate conflicts.
- Resource Efficiency: Optimize the use of CPU, memory, and storage efficiently.
- Disaster Recovery: Streamline backup and restore processes.
In short, virtualization provides many opportunities, but it's vital to navigate its challenges effectively.
Common Pitfalls
1. Improper JVM Configuration
When deploying Java applications in virtualized environments, developers often overlook JVM settings. Using default settings can lead to suboptimal performance. Key configurations to consider include:
-
Heap Size: Adjust the heap size based on the needs of your application. A larger heap may reduce garbage collection frequency but can adversely affect performance.
public class MyApplication { public static void main(String[] args) { // Memory allocation long maxHeapSize = 1024 * 1024 * 1024; // 1 GB System.setProperty("java.vm.maxHeapSize", String.valueOf(maxHeapSize)); } }
Why: This code sets the maximum heap size, which can help prevent memory-related issues.
-
Garbage Collection: Choose an appropriate garbage collection strategy based on application characteristics. Options include G1, CMS, and Parallel GC.
java -XX:+UseG1GC -jar MyApplication.jar
Why: G1GC is often beneficial for applications with large heaps and low pause time requirements.
2. Neglecting Resource Allocation
Another common pitfall is allocating insufficient or excessive resources to virtual machines (VMs). VMs need to be scaled according to workload. Under-provisioning can lead to performance bottlenecks, while over-provisioning can lead to unnecessary costs.
- CPU and Memory Monitoring: Regularly monitor usage patterns to adjust allocations efficiently.
3. File System Latency
Virtual machines often rely on shared file systems, which can introduce noticeable latency. This issue is especially prevalent for Java applications that frequently access disk I/O.
Recommended Approach:
Utilize in-memory databases or cache mechanisms:
public class CacheExample {
// Simple local cache using HashMap
private static final Map<String, String> cache = new HashMap<>();
public static void putInCache(String key, String value) {
cache.put(key, value);
}
public static String getFromCache(String key) {
return cache.get(key);
}
}
Why: By caching frequently accessed data, you minimize disk I/O operations significantly.
4. Networking Issues
Virtualizing Java applications can introduce networking-related performance issues due to increased latency and bandwidth limitations. When applications need to communicate with other services, these issues can greatly affect performance.
Solutions:
- Use HTTP/2 or gRPC for efficient communication.
- Set up Load Balancers to distribute incoming traffic effectively.
5. Inadequate Monitoring and Logging
In a virtualized environment, a lack of monitoring and logging can lead to difficulties in diagnosing performance issues or anomalies.
Recommended Tools:
- Prometheus: For metrics collection and monitoring.
- ELK Stack: For logging and searching through log data.
Example Setup Code for Prometheus:
# prometheus.yml configuration snippet
scrape_configs:
- job_name: 'java_application'
static_configs:
- targets: ['localhost:9090'] # Java application endpoint
Why: This configuration ensures that your Java application is monitored in real-time, enabling faster debugging.
6. Overlooking Security Considerations
Security is often neglected in virtualized Java applications. Virtual machines can be susceptible to attacks if not properly secured.
Best Practices:
- Regularly patch security vulnerabilities.
- Use Virtual Private Networks (VPNs) to secure data in transit.
7. Failure to Test in a Virtualized Environment
One of the most significant missteps is not testing the Java application in the environment it will run in. Local development setups seldom mirror production environments which may lead to unexpected issues.
Recommended Strategy:
- Create a staging environment that mimics your production setup. Run performance benchmarks and behavioral tests unique to your Java application.
Closing the Chapter
Virtualizing Java applications comes with its own set of challenges. By being aware of these common pitfalls - including improper JVM configuration, resource allocation, file system latency, networking issues, inadequate monitoring, security flaws, and neglecting proper testing - developers can effectively mitigate risks and maximize the benefits of virtualization.
By understanding the underlying causes and implementing appropriate strategies, you can take full advantage of what virtualization offers. For those interested in diving deeper into this topic, consider these resources:
- The Java™ Language Specification
- Java Performance: The Definitive Guide
Continuously learn and adapt your practices, and you’ll pave the way for successful deployments in virtualized environments!