Mastering the Template Method Pattern for Cleaner Code
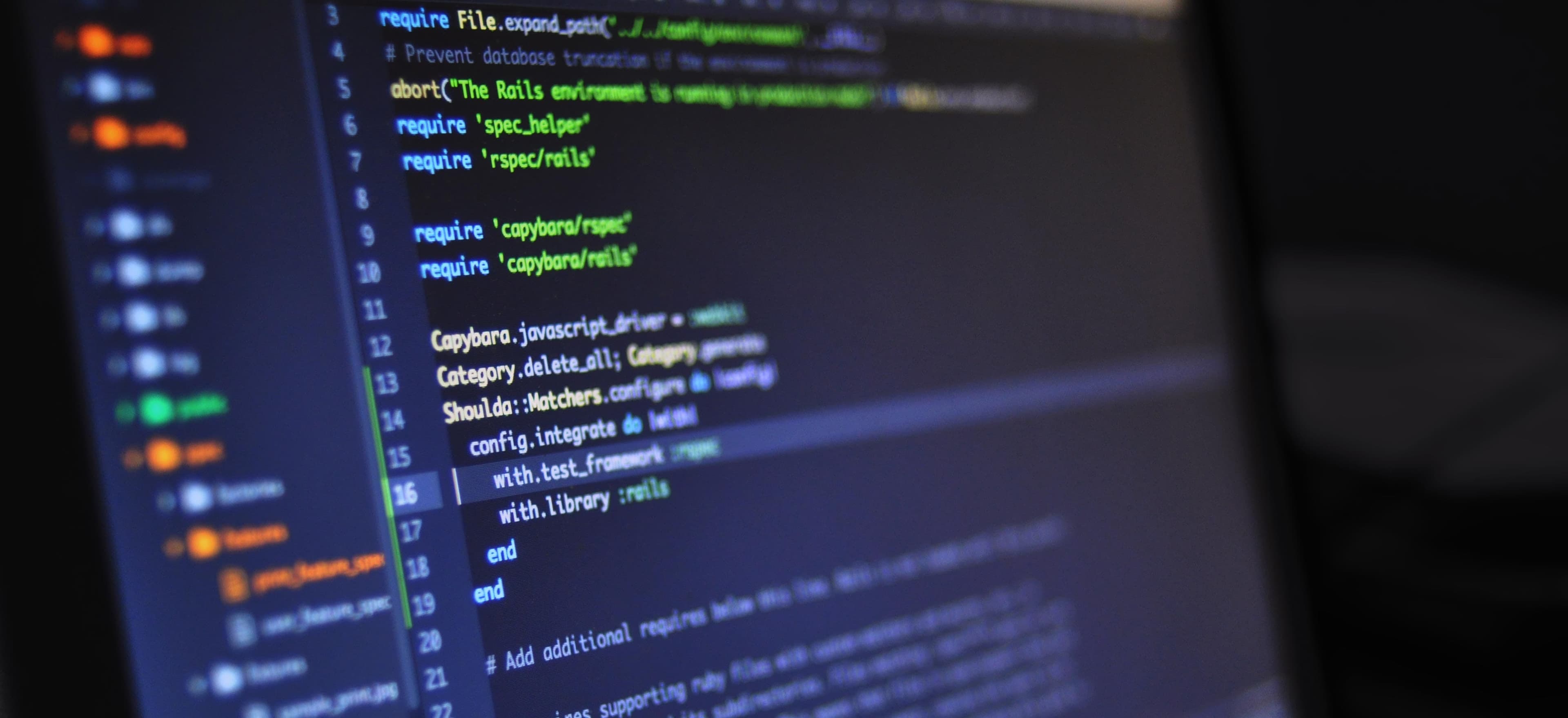
- Published on
Mastering the Template Method Pattern for Cleaner Code
In the world of software engineering, clean and maintainable code is paramount. One effective design pattern for achieving this is the Template Method Pattern. This pattern allows base classes to define the skeleton of an algorithm while deferring some steps to subclasses. By mastering this pattern, you can improve code readability and reuse, reduce duplication, and enhance flexibility.
In this blog post, we'll delve into the Template Method Pattern, explore its components, and provide practical examples to solidify your understanding. Let’s embark on this journey into cleaner code!
What is the Template Method Pattern?
The Template Method Pattern is a behavioral design pattern. It allows you to define the structure of an algorithm in a base class, while letting subclasses implement specific details. This promotes code reuse and enforces a common structure across different implementations.
Key Components of the Template Method Pattern
-
Abstract Class: This class contains the template method, which defines the series of steps of the algorithm. It also has abstract methods that subclasses must implement.
-
Concrete Class: These classes implement the details of the algorithm defined in the abstract class.
-
Template Method: This is the key aspect of the pattern. It defines the general process flow, calling the abstract methods at appropriate steps.
When to Use the Template Method Pattern
- Common Algorithm Structure: When multiple classes share a similar algorithm but differ in specific steps.
- Code Reuse: To avoid duplication and centralize the algorithm in a base class.
- Ease of Maintenance: When modifications are needed in the algorithm, you can do so in one place rather than in multiple subclasses.
Example: A Cooking Process
Let’s consider an example of a cooking process. We have different types of dishes (like soup and salad), but each dish follows a general cooking structure.
Step 1: Define the Abstract Class
public abstract class CookingTemplate {
// The template method defines the process
public final void cook() {
gatherIngredients();
prepare();
cook();
serve();
}
protected abstract void gatherIngredients();
protected abstract void prepare();
protected abstract void cook();
private void serve() {
System.out.println("Serving the dish.");
}
}
Commentary
- The
cook()
method is the template method. It outlines the general steps for cooking. - The methods
gatherIngredients
,prepare
, andcook
are abstract and must be implemented by subclasses. - The
serve()
method is a concrete method called at the end. This establishes a common serving procedure across all dishes.
Step 2: Implement Concrete Classes
Concrete Class for Soup
public class Soup extends CookingTemplate {
@Override
protected void gatherIngredients() {
System.out.println("Gathering ingredients for soup: vegetables, water, spices.");
}
@Override
protected void prepare() {
System.out.println("Chopping vegetables for the soup.");
}
@Override
protected void cook() {
System.out.println("Cooking the soup on the stove for 30 minutes.");
}
}
Concrete Class for Salad
public class Salad extends CookingTemplate {
@Override
protected void gatherIngredients() {
System.out.println("Gathering ingredients for salad: lettuce, tomatoes, cucumber.");
}
@Override
protected void prepare() {
System.out.println("Chopping vegetables for the salad.");
}
@Override
protected void cook() {
// No cooking required for salad
System.out.println("No cooking needed for the salad.");
}
}
Step 3: Utilizing the Pattern
Now that we have our abstract class and concrete implementations, we can use them as follows:
public class TemplateMethodExample {
public static void main(String[] args) {
CookingTemplate soup = new Soup();
soup.cook();
System.out.println();
CookingTemplate salad = new Salad();
salad.cook();
}
}
Commentary
- In the
main
method, we create instances ofSoup
andSalad
and call thecook()
method. - Each dish follows its own unique preparations while adhering to the general structure defined in the abstract class.
Advantages of the Template Method Pattern
- Code Reuse: Common functionality is centralized in one place, reducing redundancy.
- Flexibility: Subclasses can define their specific behavior while adhering to a consistent structure.
- Ease of Maintenance: Updates to the core algorithm can be made without altering subclasses.
Limitations of the Template Method Pattern
While the Template Method Pattern has numerous benefits, it's important to recognize its limitations:
- Rigid Structure: The algorithm's structure is fixed. Adding new steps without modifying the base class can be challenging.
- Subclass Complexity: Subclasses may become too complex if they need to implement many abstract methods, leading to increased maintenance costs.
- Tight Coupling: The pattern may result in tight coupling between a base class and its subclasses, which can make the system less flexible.
When Not to Use the Template Method Pattern
- If your algorithms frequently change or if there is no shared algorithm structure.
- If the algorithm has too many variations, it might be more prudent to consider other design patterns, such as the Strategy Pattern.
Final Thoughts
The Template Method Pattern is an invaluable tool for creating cleaner and more maintainable code. By clearly defining a skeleton of an algorithm in a base class while allowing subclasses to implement specific steps, developers can greatly enhance the flexibility and reuse of their codebase.
As you incorporate this pattern into your development practices, remember to balance its use with the potential for complexity it introduces. Use it thoughtfully, and you will experience the benefits of a well-structured codebase that adheres to the principles of clean code design.
For further reading on design patterns in Java, consider checking out Refactoring Guru's Design Patterns or the book “Design Patterns: Elements of Reusable Object-Oriented Software” by Gamma et al. to deepen your understanding.
Embrace the Template Method Pattern and transform your coding practices into a masterpiece of efficiency and elegance! Happy coding!
Checkout our other articles