Eliminating Common Code Smells in Java Applications
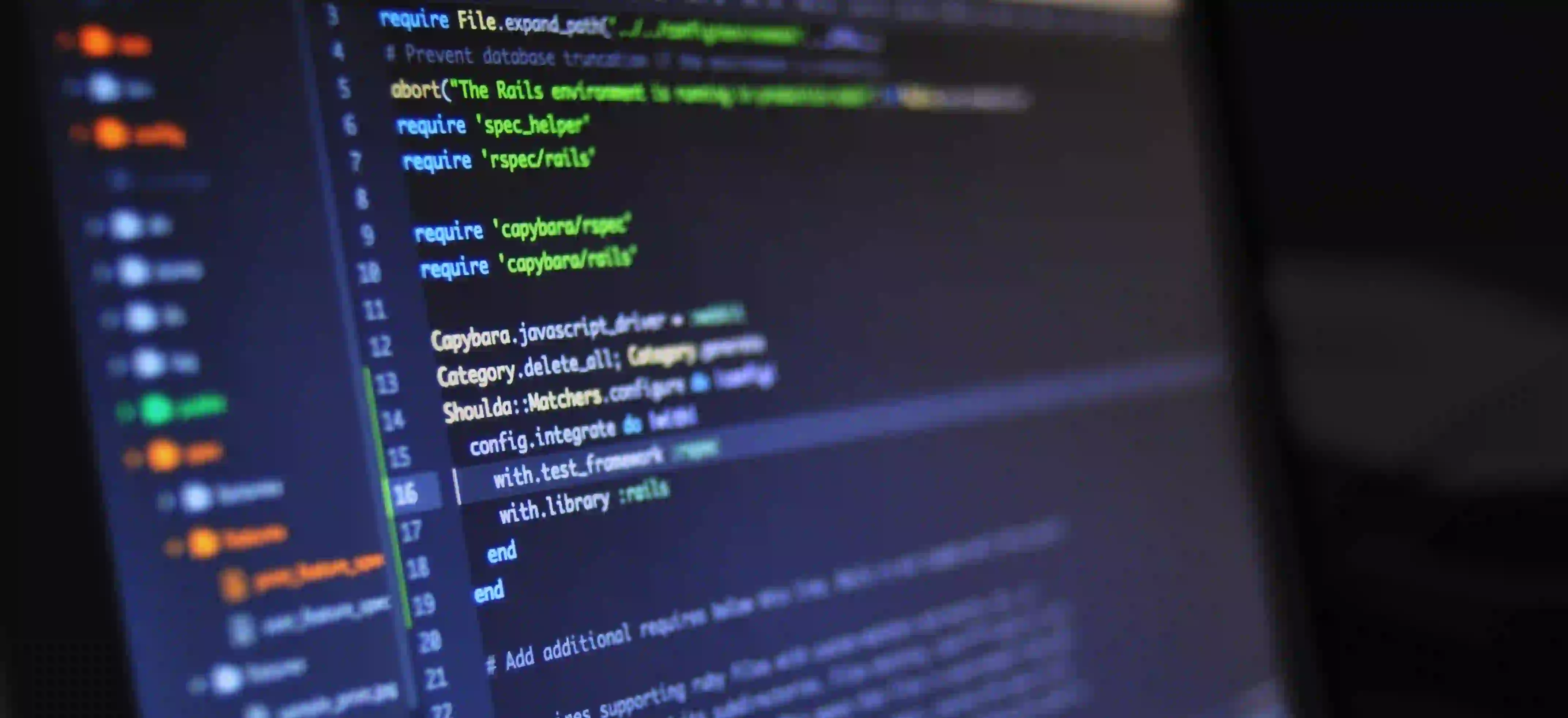
Eliminating Common Code Smells in Java Applications
In the world of software development, particularly in Java application development, the concept of "code smells" is often discussed. Code smells refer to certain patterns in code that may indicate deeper issues. They are not bugs, meaning they won't cause the application to crash or behave incorrectly. However, their presence can lead to maintainability problems, technical debt, and ineffective collaboration in the long run. In this blog post, we'll explore some common code smells in Java and how to eliminate them.
What are Code Smells?
Code smells can be thought of as hints that something might be wrong with the code. They often arise from poor design, lack of understanding of the problem domain, or simply bad habits formed over time. Identifying these smells is the first step toward improvement, and we can utilize principles from Clean Code by Robert C. Martin to aid in this process.
Why Focus on Code Smells?
Addressing code smells can lead to several benefits:
- Improved readability: Cleaner code is easier to understand.
- Enhanced maintainability: Code that’s easy to navigate is far easier to modify.
- Greater cohesion and reduced coupling: Well-designed code promotes better organization.
Common Code Smells and How to Fix Them
1. Long Methods
Description: A method that is excessively long often indicates that it's trying to perform too many tasks. This violates the Single Responsibility Principle.
Solution: Break down long methods into smaller, more focused methods.
Code Example:
public void processCustomerOrder(Order order) {
// Validate order
if (order == null || order.getItems().isEmpty()) {
throw new IllegalArgumentException("Invalid order");
}
// Process payment
processPayment(order);
// Ship order
shipOrder(order);
}
// Breaking down the processPayment method
private void processPayment(Order order) {
// Execute payment logic here
}
private void shipOrder(Order order) {
// Execute shipping logic here
}
Why: The processCustomerOrder
method is broken down into specific parts, making it easier for others (and future you) to understand and maintain.
2. Duplicated Code
Description: Duplicated code is a clear sign of code smell. When the same code is repeated, it increases the risk of bugs and makes modifications significantly harder.
Solution: Extract duplicate code into a single method.
Code Example:
// Before refactoring
public double calculateOrderTotal(List<Item> items) {
double total = 0.0;
for (Item item : items) {
total += item.getPrice();
}
return total;
}
public double calculateInvoiceTotal(List<Invoice> invoices) {
double total = 0.0;
for (Invoice invoice : invoices) {
total += invoice.getTotal();
}
return total;
}
// After refactoring to eliminate duplication
public double calculateTotal(Collection<? extends Payable> payables) {
double total = 0.0;
for (Payable payable : payables) {
total += payable.getPrice();
}
return total;
}
Why: Extracting duplicate logic into a single method reduces redundancy and enhances maintainability. Now, if a change is needed, it only has to be done in one place.
3. Large Classes
Description: Classes that contain too many responsibilities can be cumbersome. They become challenging to understand and maintain.
Solution: Adopt class decomposition, separating concerns into distinct classes.
Code Example:
public class UserProfile {
private String username;
private String email;
private String password;
public void updateProfile(String email, String password) {
// Update logic
}
public void sendEmailVerification() {
// Email logic
}
}
// After refactoring into multiple classes
public class UserDetails {
private String username;
private String email;
public void updateEmail(String email) {
this.email = email;
}
}
public class UserAuthentication {
private String password;
public void updatePassword(String password) {
this.password = password;
}
}
Why: The new structure adheres to the Single Responsibility Principle, making each class easier to manage and change individually.
4. Inappropriate Intimacy
Description: This code smell occurs when classes are too tightly coupled, which means one class is overly dependent on the internals of another.
Solution: Reduce dependency via interfaces or refactor based on responsibilities.
Code Example:
public class Customer {
private Order order;
public void displayOrderDetails() {
System.out.println(order.getDetails());
}
}
// After introducing an interface
public interface OrderDetailsProvider {
String getDetails();
}
public class Customer {
private OrderDetailsProvider orderProvider;
public void displayOrderDetails() {
System.out.println(orderProvider.getDetails());
}
}
Why: By introducing an interface, you decouple the classes, making it easier to change the behavior of either without affecting the other.
5. Magic Numbers and Strings
Description: The use of unexplained numbers or string values in code can lead to confusion and code that’s not self-explanatory.
Solution: Replace magic values with named constants.
Code Example:
public static final double DISCOUNT_RATE = 0.15;
// Applying a discount
double finalPrice = price - (price * 0.15);
// After refactoring
public static final double DISCOUNT_RATE = 0.15;
double finalPrice = price - (price * DISCOUNT_RATE);
Why: Named constants provide context, improving code readability while reducing the risk of errors.
To Wrap Things Up
Eliminating code smells requires a shift in mindset. Regular code reviews, adherence to well-defined principles, and the practice of refactoring can greatly enhance the long-term sustainability of your Java applications. For more details on design principles that encourage clean coding, consider checking out Martin's Clean Code workshops and Robert C. Martin's books.
Remember, the goal is not perfection but continuous improvement. The more you practice identifying and resolving code smells, the more intuitive it will become. Embrace the journey towards cleaner, more maintainable code!