How to Fix Common Issues with Android Image Filters
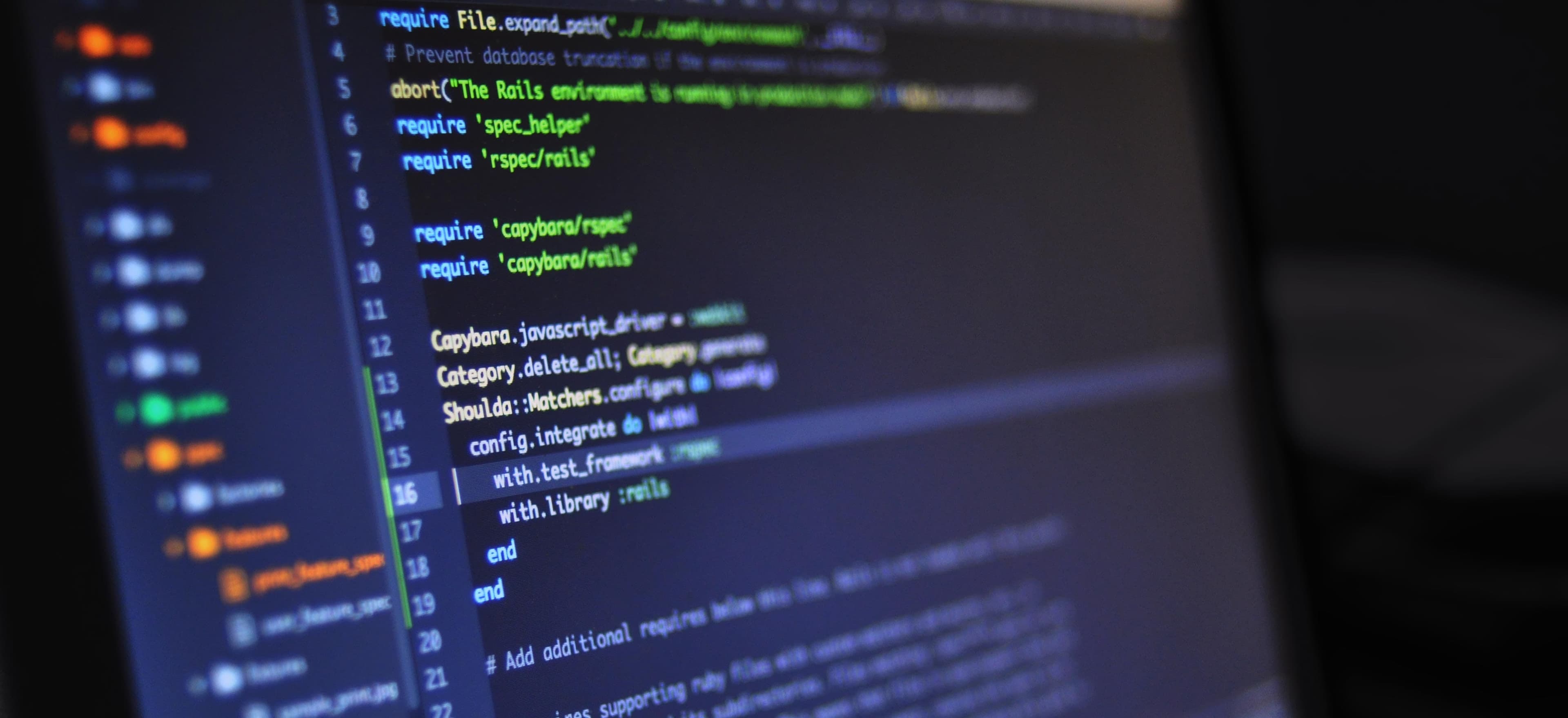
- Published on
How to Fix Common Issues with Android Image Filters
Image filters are a standout feature in Android development, enabling developers to enhance visuals and provide users with an engaging experience. However, working with image filters can sometimes present challenges ranging from performance issues to unexpected results. In this blog post, we will walk through common issues encountered when implementing image filters in Android, along with practical solutions that will keep your applications performing optimally.
Understanding Image Filters
Before delving into the issues, it's essential to understand what image filters are. They are graphical filters applied to image data that modify or enhance the input image. Filters can change brightness, contrast, saturation, and apply various effects like blurring or sharpening.
For more details on image processing in Android, check out the official Android documentation.
Common Issues with Android Image Filters
1. Performance Issues
Problem: Applying image filters can be resource-intensive. This may slow down the app, especially on low-end devices.
Solution: Use Bitmap
caching and offload processing to a background thread.
Code Example:
// This method runs image processing in a background thread
private void applyFilterAsync(final Bitmap originalBitmap) {
new Thread(new Runnable() {
@Override
public void run() {
Bitmap filteredBitmap = applyFilter(originalBitmap);
// Update the UI on the main thread
runOnUiThread(new Runnable() {
@Override
public void run() {
imageView.setImageBitmap(filteredBitmap);
}
});
}
}).start();
}
// The actual filter application
private Bitmap applyFilter(Bitmap src) {
// Apply your filter logic here
// Example: changing saturation
// ...
return filteredBitmap;
}
Why: By using background threading with AsyncTask
or Runnable
, you ensure that the UI remains responsive while resolving intensive tasks like image filtering.
2. Memory Leaks
Problem: High-resolution images consumed by filters can lead to OutOfMemoryError
.
Solution: Use bitmap options for inSampleSize.
Code Example:
private Bitmap decodeSampledBitmapFromResource(String pathName, int reqWidth, int reqHeight) {
final BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true;
BitmapFactory.decodeFile(pathName, options);
// Calculate inSampleSize
options.inSampleSize = calculateInSampleSize(options, reqWidth, reqHeight);
options.inJustDecodeBounds = false;
return BitmapFactory.decodeFile(pathName, options);
}
private int calculateInSampleSize(BitmapFactory.Options options, int reqWidth, int reqHeight) {
final int height = options.outHeight;
final int width = options.outWidth;
int inSampleSize = 1;
if (height > reqHeight || width > reqWidth) {
final int halfHeight = height / 2;
final int halfWidth = width / 2;
// Calculate the largest inSampleSize value that is a power of 2 and keeps both
// height and width larger than the requested height and width.
while ((halfHeight / inSampleSize) >= reqHeight && (halfWidth / inSampleSize) >= reqWidth) {
inSampleSize *= 2;
}
}
return inSampleSize;
}
Why: By leveraging inSampleSize
, you can downsample images which conserves memory while maintaining acceptable quality for user interface displays.
3. Incorrect Color Levels
Problem: Filters may produce unexpected results if the color levels are not handled correctly.
Solution: Ensure you're managing color formats effectively throughout your processing.
Code Example:
private int colorToGrayscale(int color) {
int alpha = Color.alpha(color);
int red = Color.red(color);
int green = Color.green(color);
int blue = Color.blue(color);
// Calculate luminance value
int gray = (int) (0.299 * red + 0.587 * green + 0.114 * blue);
return Color.argb(alpha, gray, gray, gray);
}
Why: In the grayscale conversion, controlling the color channels properly results in a more visually appealing filter output. This helps to avoid washed-out or improperly tinted images.
4. Filter Stack Order
Problem: The order in which filters are applied can significantly alter the final output, sometimes detracting from the intended effect.
Solution: Carefully plan and document your filter order based on desired visual outcomes.
Example Filter Order:
- Base Filter: Apply any foundational changes first (e.g., resizing, cropping).
- Adjustment Filters: Then modify brightness, contrast, saturation—fundamental tweaks to the image.
- Effect Filters: Finally, apply any stylistic effects like vignetting or texture overlays.
// Example method applying filters in a predetermined order
private Bitmap applyFiltersInOrder(Bitmap originalBitmap) {
Bitmap result = resizeBitmap(originalBitmap);
result = adjustBrightness(result, 30);
result = applyVignette(result);
return result;
}
Why: By strategizing the order in which filters are applied, you can achieve more desirable results without having to reverse engineer unwanted alterations later.
5. Inadequate Testing on Different Devices
Problem: Filters that work on one device may perform poorly on another due to variations in hardware.
Solution: Always test your filters on a variety of devices, particularly on those with lower specifications.
Why: Testing across several devices ensures that any performance issues are identified and addressed early in the development process, enhancing overall user experience.
The Last Word
Implementing image filters in Android can prove beneficial but requires a thoughtful approach to address common issues. By following best practices related to performance, memory management, color handling, filter stacking, and testing, you can deliver high-quality image processing features that delight your users.
Don't hesitate to explore more about image processing on Android through resources like Android Image Processing and Android's Graphics API. With the appropriate strategies, you can enhance your Android applications and guarantee an enjoyable user experience.