How to Effectively Stop and Destroy Threads in Java
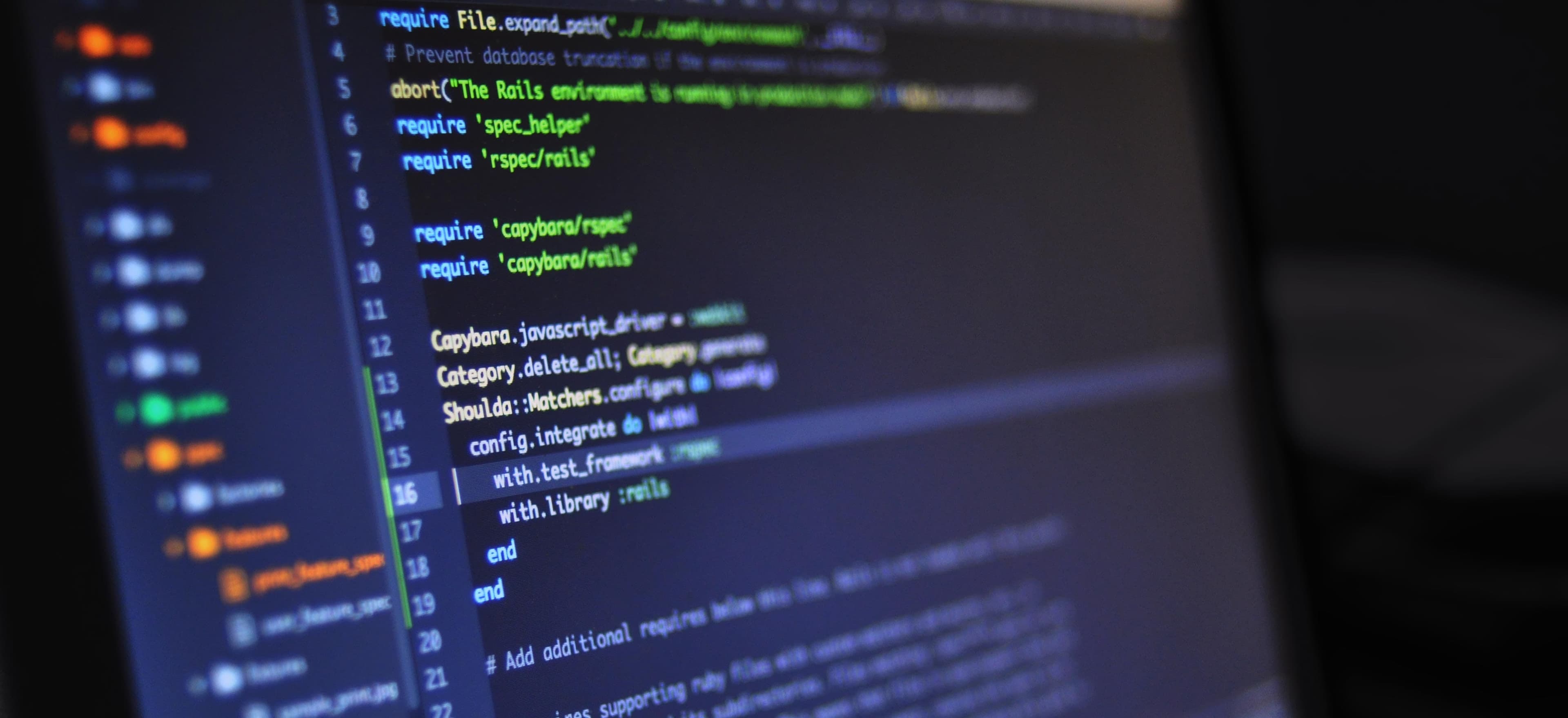
- Published on
How to Effectively Stop and Destroy Threads in Java
Multi-threading is one of the core features of Java, allowing developers to achieve significant performance enhancements and responsive applications. However, managing thread life cycles can be challenging, especially when it comes to stopping or destroying threads. This blog post will delve into the elegant and effective ways to stop and destroy threads in Java.
Understanding Threads in Java
Before we dive into the mechanisms for stopping threads, it's essential to understand what a thread is. A thread in Java is a lightweight sub-process, the smallest unit of processing. Java utilizes an abstraction known as a "thread" to perform concurrent execution of two or more parts of a program.
Why You Need to Stop Threads
There are various scenarios where you may need to stop threads:
- Resource Management: To avoid memory leaks and ensure that resources like file handles and network connections are released.
- User Input: In GUI applications, threads may need to be stopped based on user actions.
- Timeouts: If a particular thread takes too long to complete its task, control may need to be handed back to the user.
The Thread Lifecycle
In Java, a thread can exist in several states:
- NEW: A thread is in this state just after it has been created, but not started.
- RUNNABLE: A thread is executing in this state or waiting for CPU scheduling.
- BLOCKED: A thread is blocked waiting for a monitor lock.
- WAITING: A thread is waiting indefinitely for another thread to perform a particular action.
- TIMED_WAITING: A thread that is waiting for another thread for up to a specified waiting time.
- TERMINATED: A thread that has exited.
Understanding these states will help you better manage your threads.
Thread Life Management
1. Using an Interrupted Flag
The most graceful way to stop a running thread is to use a flag to signify that the thread should stop its execution. This allows the thread to finish its current task properly and clean up resources.
public class StoppableThread extends Thread {
private volatile boolean running = true;
public void run() {
while (running) {
try {
// Simulate some work
Thread.sleep(1000);
System.out.println("Thread is running...");
} catch (InterruptedException e) {
Thread.currentThread().interrupt(); // Restore the interrupted status
System.out.println("Thread was interrupted");
break; // Exit loop if an interruption occurs
}
}
System.out.println("Thread has stopped.");
}
public void stopThread() {
running = false; // Signal the thread to stop
}
}
Commentary
- volatile: We declare
running
asvolatile
to ensure that changes to it are visible across all threads immediately. - InterruptedException: Catch this exception to handle cases where the thread is interrupted while sleeping or waiting.
- Graceful Exit: This method allows the thread to finish its current execution before stopping.
2. Using interrupt()
Java's threading model provides the interrupt()
method, which can be utilized to signal a thread to stop what it is doing. However, the thread must be programmed to respond to this interruption properly.
public class InterruptibleThread extends Thread {
public void run() {
try {
for (int i = 0; i < 10; i++) {
// Simulate some work
System.out.println("Working: " + i);
Thread.sleep(500); // Sleep for half a second
}
} catch (InterruptedException e) {
System.out.println("Thread was interrupted during sleep.");
}
System.out.println("Thread has exited.");
}
}
Commentary
- Handling InterruptedException: Always handle the
InterruptedException
to know when a thread has been interrupted during sleep or wait. - Typical Use Case: This is particularly useful when threads are executing long-running tasks that can be safely interrupted.
3. Thread Pools and ExecutorService
Using a thread pool can simplify thread management. The ExecutorService
interface allows executing tasks asynchronously and managing life cycles more effectively.
import java.util.concurrent.Executors;
import java.util.concurrent.ExecutorService;
public class ExecutorServiceExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(2);
Runnable task1 = () -> {
try {
for (int i = 0; i < 5; i++) {
System.out.println("Task 1 - " + i);
Thread.sleep(500);
}
} catch (InterruptedException e) {
System.out.println("Task 1 interrupted.");
}
};
Runnable task2 = () -> {
try {
for (int i = 0; i < 5; i++) {
System.out.println("Task 2 - " + i);
Thread.sleep(500);
}
} catch (InterruptedException e) {
System.out.println("Task 2 interrupted.");
}
};
executor.submit(task1);
executor.submit(task2);
executor.shutdownNow(); // It will stop all active tasks
}
}
Commentary
- ExecutorService: It is a higher-level replacement for managing threads compared to the raw
Thread
class. - shutdownNow(): This call attempts to stop all actively executing tasks and halts the processing of waiting tasks.
Best Practices for Stopping Threads
- Avoid using
Thread.stop()
: Thestop()
method is deprecated because it can leave shared resources in inconsistent states. - Use Volatile Boolean Flags: They allow for soft stopping of threads.
- Handle
InterruptedException
properly: Always check for interruptions during long-running tasks. - Utilize Executor Framework: Thread pools manage resources effectively and abstract thread management complexity.
Wrapping Up
Stopping and destroying threads in Java is a critical aspect that requires careful consideration. Whether you decide to use a simple boolean flag, the interrupt()
method, or the more robust ExecutorService
, the key takeaway is to always allow your threads to terminate gracefully.
By adhering to these practices, you not only preserve the integrity of shared resources but also improve the readability and maintainability of your code.
For further reading on Java threads and concurrency, check out the Java Concurrency Tutorial provided by Oracle.
Understanding the nuances of thread management will contribute to building resilient and responsive Java applications. Happy coding!
Checkout our other articles