Navigating Compatibility Issues with JDK 14's New Features
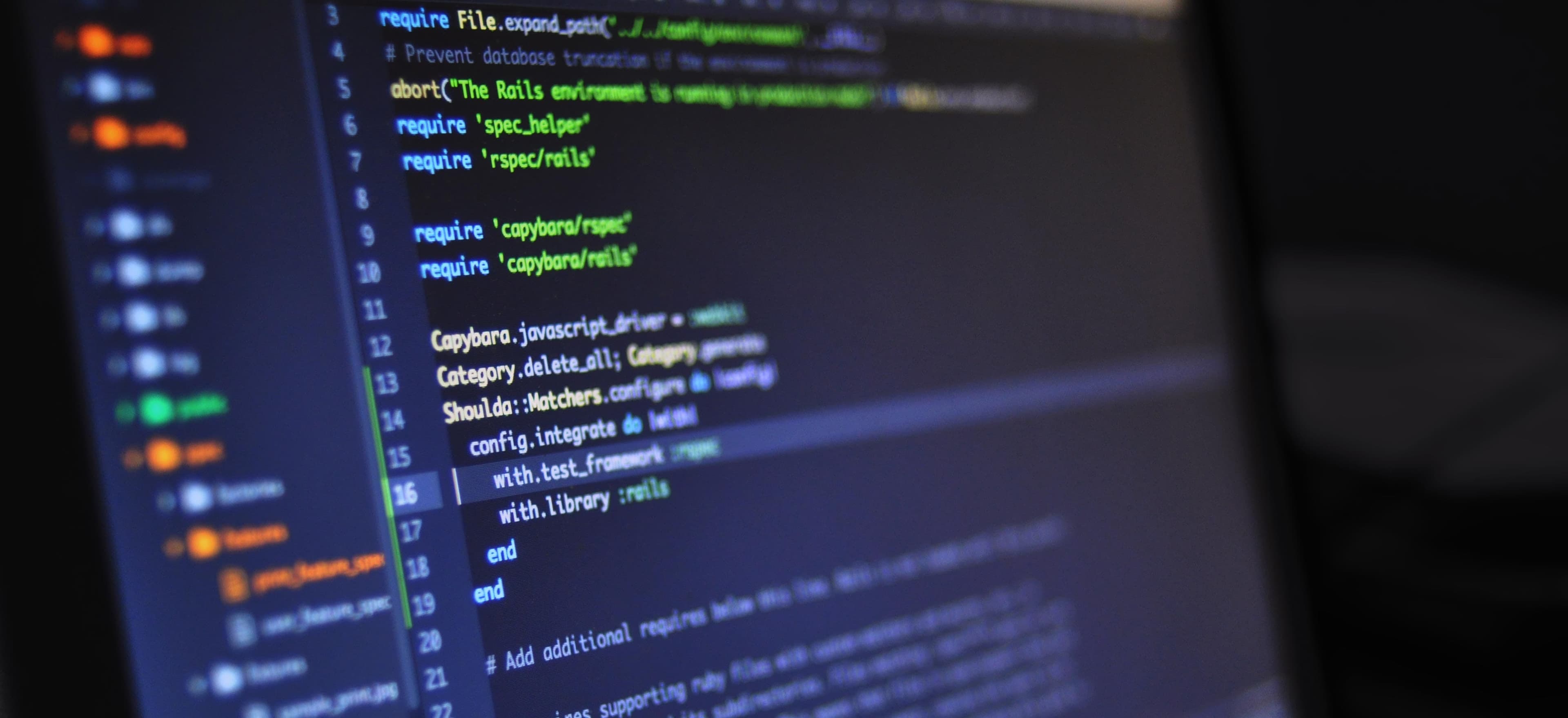
- Published on
Navigating Compatibility Issues with JDK 14's New Features
Java Development Kit (JDK) 14 introduced a slew of features that are aimed at enhancing developer productivity and improving program performance. However, these new features can sometimes introduce compatibility issues. In this blog post, we will explore some significant features in JDK 14, highlight potential compatibility challenges, and provide best practices to navigate through these challenges effectively.
Starting Off to JDK 14 Features
JDK 14 brought several noteworthy enhancements, including:
- JEP 305: Pattern Matching for instanceof
- JEP 343: Packaging Tool (Incubator)
- JEP 345: NUMA-aware memory allocation
- JEP 338: Vector API (Incubator)
Let's delve into each feature, discussing its compatibility implications in detail.
Pattern Matching for instanceof
What is it?
Pattern matching allows developers to simplify the common practice of casting an object after an instanceof
check. Here is a simple code example:
if (obj instanceof String s) {
System.out.println(s.toUpperCase());
}
Compatibility Issues
While this feature seems to streamline code, it can lead to compatibility issues with older Java versions. For instance, developers working in mixed environments may face difficulties if:
- Some modules are still using legacy Java versions.
- Existing codebases rely on older casting techniques.
Solutions
To mitigate these issues, adhere to best practices:
- Use version control to ensure proper code testing across different JDK versions.
- Code refactoring to maintain compatibility while leveraging JDK 14 features.
- Educate teams about the new features to avoid confusion in collaborative projects.
Resources
For a more in-depth discussion of pattern matching, have a look at the official JDK 14 Release Notes.
Packaging Tool (Incubator)
What is it?
The Packaging Tool introduced in JDK 14 allows developers to package Java applications as native installers. For instance:
jpackage --input input-dir --name MyApp --main-jar myapp.jar
Compatibility Issues
The tool is packaged as an incubator, meaning it's still a work in progress. Compatibility issues here might arise because:
- The tool is not available in all distributions of Java.
- Existing build systems may not support this new workflow yet.
Solutions
- Evaluate your project needs before integrating the Packaging Tool.
- Test thoroughly on local environments, as well as in deployment configurations.
If you want more information about using the Packaging Tool effectively, you might find Oracle's JPackage Guide useful.
NUMA-aware Memory Allocation
What is it?
NUMA (Non-Uniform Memory Access) allows applications to exploit multi-threading across processors and allocate memory efficiently. This can vastly improve performance for specific workloads.
Compatibility Issues
The introduction of NUMA-aware memory allocation may conflict with applications that rely on Java's default memory allocation behavior. For instance, if a legacy application was optimized for a single-node system, it might misbehave under a NUMA configuration due to improper memory distribution:
System.setProperty("java.nio.channels.spi.SelectorProvider", "sun.nio.ch.PipeSelectorProvider");
Solutions
To ensure compatibility:
- Profile your application by monitoring memory usage under different loads.
- Use appropriate flags when running your Java application to enable or disable NUMA awareness.
Vector API (Incubator)
What is it?
The Vector API introduces a way to express operations on multiple data points in a single operation. This is particularly useful for high-performance computing. Here’s an example:
import jdk.incubator.vector.*;
Vector<Integer> vector = IntVector.fromArray(VectorSpecies.ofInt(), new int[]{1, 2, 3}, 0);
Compatibility Issues
Being an incubator module, it might have incomplete functionality or API changes leading to compatibility challenges. Also, not all environments may support it.
Solutions
- Regularly review updates to the API as it evolves.
- Restrict its use to specific modules while retaining traditional looping methods for others to maintain compatibility.
Best Practices for Navigating Compatibility Issues
-
Gradual Adoption: Introduce new features gradually into existing projects. This can help identify issues without overwhelming developers.
-
Use Compatibility Libraries: Consider leveraging compatibility libraries that help facilitate transitions between different Java versions.
-
Automated Testing: Implement automated unit and integration testing to catch compatibility issues early in the development cycle.
-
Stay Updated: Always keep an eye out for the latest Java enhancements and community feedback.
Closing the Chapter
The features introduced in JDK 14 can significantly enhance Java applications, but navigating compatibility challenges requires a thoughtful approach. It is essential to fully understand the implications of using new features like pattern matching, the packaging tool, NUMA-aware memory allocation, and the experimental Vector API. Adopting best practices can ensure a smooth transition, allowing developers to leverage new innovations without sacrificing performance or stability.
By staying informed and proactive, developers can harness the power of JDK 14 while minimizing compatibility headaches. Always consider engaging with the broader Java community through forums and Q&A sites when navigating complexities, as collective knowledge is invaluable.
By focusing on understanding and mitigating compatibility issues, developers can look forward to a more streamlined and efficient experience with their Java applications in a JDK 14 environment. Happy coding!