Common Pitfalls in Using JUnit 5 Extensions
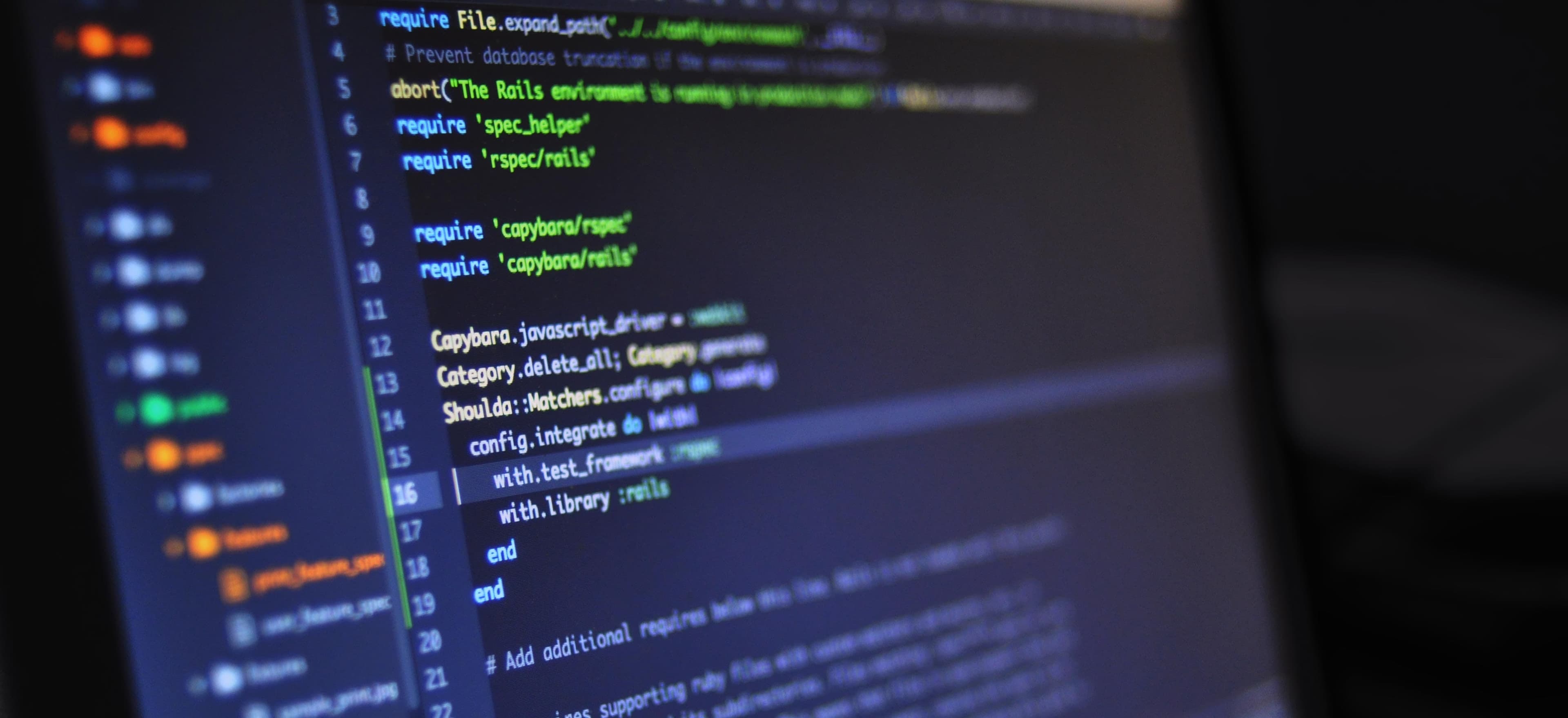
- Published on
Common Pitfalls in Using JUnit 5 Extensions
JUnit 5, the newest version of the popular Java testing framework, provides powerful capabilities that enable developers to write cleaner and more efficient test code. One of the standout features is its extension model, which allows developers to augment the behavior of tests, test instances, and test lifecycle.
However, as with any feature, pitfalls abound. In this blog post, we’ll explore common mistakes developers make while using JUnit 5 extensions, how to avoid them, and best practices for integrating extensions into your testing strategy.
What are JUnit 5 Extensions?
JUnit 5 extensions allow you to intercept and modify the way your tests are executed. This feature is made possible through the use of interfaces such as Extension
, which enables you to customize various parts of the testing lifecycle.
Why Use Extensions?
- Code Reusability: You can share test logic across multiple test classes without repeating code.
- Improved Readability: Cleaner and more organized test code promotes maintainability.
- Custom Behaviors: Easily implement cross-cutting concerns such as logging, timeout management, and more.
To learn more about sponsoring your own extensions, you might want to check out the official JUnit 5 documentation.
Common Pitfalls
1. Misunderstanding the Lifecycle
Understanding the lifecycle of a JUnit 5 test is vital before diving into extensions. Failing to recognize when tests are executed can lead to unexpected behavior.
Example:
As an example, using the @BeforeEach
annotation is common for initialization. A pitfall occurs when developers use an extension to mimic this behavior incorrectly.
import org.junit.jupiter.api.extension.BeforeEachCallback;
import org.junit.jupiter.api.extension.ExtensionContext;
public class MyExtension implements BeforeEachCallback {
@Override
public void beforeEach(ExtensionContext context) {
// This should happen before every test method
System.out.println("Setting up before each test");
}
}
In this extension, you are correctly setting up for each test. However, if you accidentally put limited or incorrect logic in this method, it may affect test outcomes unexpectedly.
Avoiding the Pitfall
Make sure to:
- Thoroughly understand the test lifecycle.
- Utilize debug logging at different lifecycle stages to ensure the order of operations is as expected.
2. Overusing Extensions
Another common mistake is over-relying on extensions. While they can provide great functionality, too many extensions can confuse your code and hide underlying behavior.
Example:
A developer might decide to implement extensions for logging, timing, and reporting, all in a single class:
import org.junit.jupiter.api.extension.ExtendWith;
// Too many responsibilities in one extension
@ExtendWith({LoggingExtension.class, TimingExtension.class, ReportingExtension.class})
public class MyTest {
}
This approach complicates your tests and can lead to maintenance nightmares.
Avoiding the Pitfall
Instead, keep extensions focused and single-purpose. Here’s an ideal example:
@ExtendWith(LoggingExtension.class)
class MyTest {
// Test methods
}
3. Improper Exception Handling
JUnit 5 allows you to expect exceptions in your tests, but your extensions need to adequately handle these scenarios. Failing to do so can lead to false positives or negatives in your tests.
Example:
An extension that catches exceptions for logging purposes might misinterpret failures:
import org.junit.jupiter.api.extension.AfterEachCallback;
import org.junit.jupiter.api.extension.ExtensionContext;
public class ExceptionHandlingExtension implements AfterEachCallback {
@Override
public void afterEach(ExtensionContext context) {
try {
// Simulate potential failure
} catch (Exception e) {
// Log the exception but mistakenly ignore it
System.out.println("Exception occurred");
}
}
}
In this case, the extension logs an exception but fails to fail the test, leading developers to miss crucial test failures.
Avoiding the Pitfall
Always rethrow exceptions if you're not handling them completely. Modify the above method to ensure it fails when an exception occurs.
@Override
public void afterEach(ExtensionContext context) throws Exception {
try {
// Simulate potential failure
} catch (Exception e) {
System.out.println("Exception occurred");
throw e; // Rethrow the exception
}
}
4. Mixing Test and Production Code
Another pitfall developers often fall into is mixing testing concerns with production concerns. Extensions should be isolated to avoid this mix-up.
Example:
Including business logic directly in your extension can lead to hard-to-maintain code:
public class MyExtension implements BeforeEachCallback {
@Override
public void beforeEach(ExtensionContext context) {
// Business logic here, which doesn't belong in a test extension
ConfigService.getConfig(); // Mixing concerns
}
}
Avoiding the Pitfall
Keep business logic out of your testing code. Use the extension solely for setup or control flows related to testing.
5. Not Utilizing Parameterized Tests
JUnit 5 supports parameterized tests, and extensions can work alongside them. Not taking advantage of this feature can lead to repetitive boilerplate code in tests.
Example:
Rather than writing separate tests for different inputs:
@Test
void testMethodA() {
// Test case A
}
@Test
void testMethodB() {
// Test case B
}
You can use an extension to parameterize test execution.
Implementing Parameterized Tests
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
class MyTests {
@ParameterizedTest
@ValueSource(strings = { "Hello", "JUnit" })
void testWithDifferentValues(String input) {
System.out.println(input);
}
}
Key Takeaways
JUnit 5 extensions offer immense potential to enhance your testing practices, but they come with pitfalls that can lead to confusion and bugs if not carefully navigated. Understanding the lifecycle, avoiding the overuse of functionalities, handling exceptions properly, separating your testing code from business logic, and utilizing parameterized tests effectively can maximize the benefit of these extensions.
By following these guidelines, you will write cleaner, more maintainable, and more effective tests. For additional reading, don’t forget to check out the JUnit 5 GitHub repository. Happy testing!
Checkout our other articles