Understanding the Use of 'final' in Java Methods
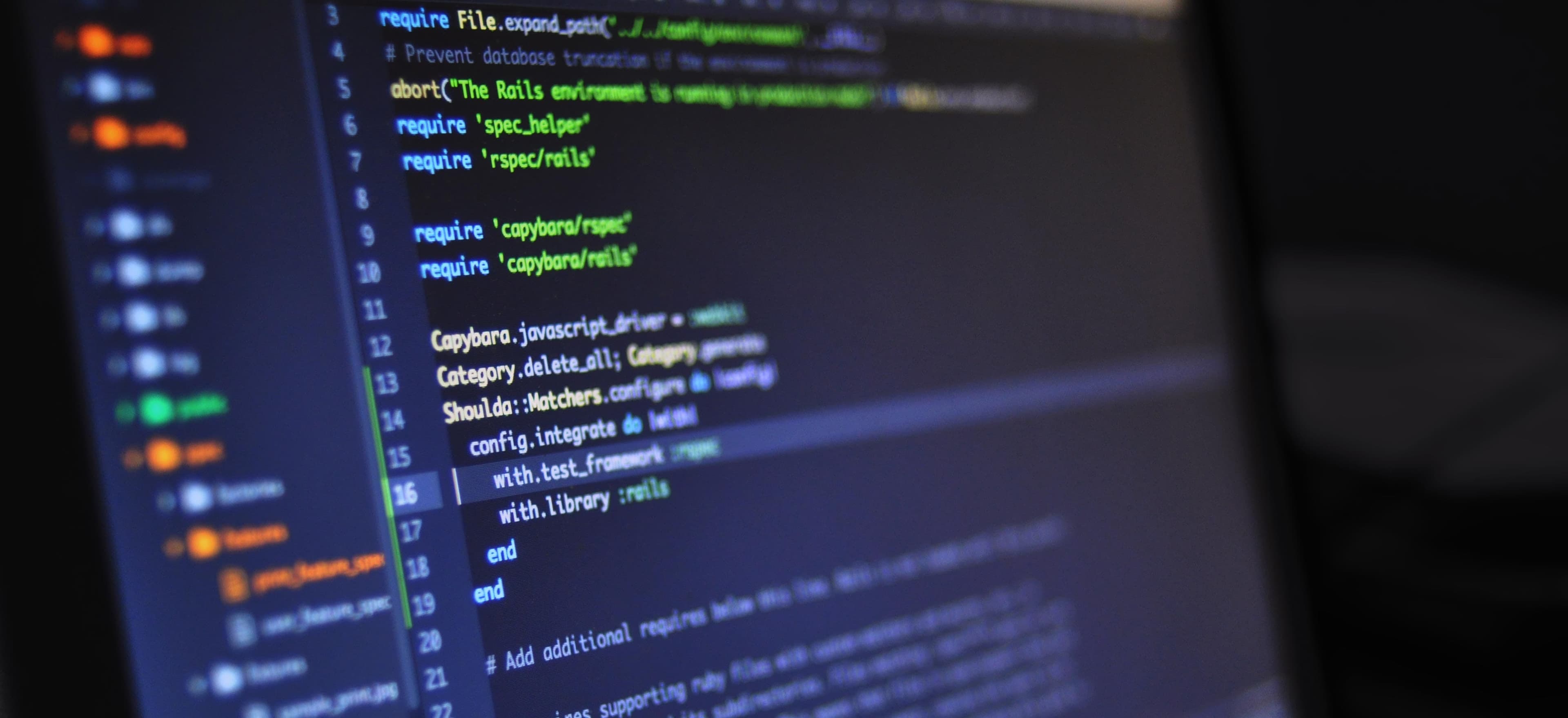
- Published on
Understanding the Use of 'final' in Java Methods
Java, as a powerful object-oriented programming language, is full of nuanced concepts that can sometimes create confusion for developers. One such concept is the final
keyword. Used in a variety of contexts, its implications can vary. In this post, we'll dive deep into the use of the final
keyword specifically relating to methods, shedding light on its importance, how it functions, and best practices.
What Does 'final' Mean in Java?
In Java, the final
keyword can be applied to variables, methods, and classes. When it comes to methods, declaring a method as final
indicates that it cannot be overridden by subclasses.
This keyword plays a critical role in inheritance, ensuring that certain desired behaviors are preserved. Using final
effectively can enhance code security and design predictability, making it an essential part of a Java developer's toolkit.
Benefits of Using 'final' with Methods
-
Prevention of Overriding: When a method is marked as
final
, any subclass that extends the parent class cannot modify its behavior. This ensures that the original implementation of the method stays intact. -
Enhanced Performance: The Java compiler can optimize calls to
final
methods. Knowing that a method is not going to change in subclasses can allow the compiler to perform optimizations that reduce method call overhead. -
Improved Code Clarity: Marking methods as
final
communicates to other developers (and your future self) that this method is not designed to be altered. This clarity can prevent unintended consequences and improve maintainability. -
Designing Immutable Classes: Final methods are often used in the context of immutable classes. If the methods that modify class properties are
final
, it ensures that instances of the class remain unchanged.
Example of 'final' in a Method
To illustrate how the final
keyword functions with methods, let's look at an example.
class Animal {
final void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
// This will cause a compile-time error
void sound() {
System.out.println("Dog barks");
}
}
Explanation of the Code
- The
Animal
class has a method,sound()
, which is marked asfinal
. - The
Dog
class extendsAnimal
. However, trying to override thesound()
method inDog
will result in a compile-time error. - This enforces that all
Animal
instances will make the same sound, highlighting the importance of code stability and clear design.
Best Practices for Using 'final' Methods
While it may be tempting to declare many methods as final
, you should use discretion. Here are some best practices:
-
Use When Necessary: Only declare methods as
final
when you have a clear reason to prevent overriding. If there's a possibility of extension or modification, consider whetherfinal
is appropriate. -
Document Your Intentions: If you declare a method as
final
, put comments in the code that explain your reasoning. This will benefit your team and clarify future maintenance. -
Apply in Immutable Classes: Use
final
methods when designing immutable objects to preserve their state. -
Consistent Style: If you're following a design pattern that favors the use of
final
, apply it consistently across your methods to prevent confusion.
Common Misconceptions
A few common misconceptions about final
methods include:
-
Final is Always Better: While the
final
keyword serves many benefits, its use is not universally applicable. Each case should be evaluated individually. -
Final Methods Can't Be Private: Private methods can also be marked as
final
. While they cannot be overridden (internally), marking them asfinal
can emphasize that they are not intended for subclass use outside of their declaring class. -
Inheritance Dynamics: Some developers believe that marking a method as
final
is a sign of poor design. However, this isn't always true. Sometimes, enforcing certain behaviors via thefinal
keyword can be a powerful design choice.
Example of 'final' with an Immutable Class
Consider an immutable class example supported by final
methods.
public final class ImmutablePoint {
private final int x;
private final int y;
public ImmutablePoint(int x, int y) {
this.x = x;
this.y = y;
}
public final int getX() {
return x;
}
public final int getY() {
return y;
}
public final ImmutablePoint move(int deltaX, int deltaY) {
return new ImmutablePoint(this.x + deltaX, this.y + deltaY);
}
}
Code Explanation
- Immutable Class: The class
ImmutablePoint
is declared asfinal
to prevent subclassing, protecting its immutability. - Final Attributes: Both attributes
x
andy
are marked asfinal
, meaning they cannot be modified after the constructor executes. - Final Methods: The getter methods
getX
andgetY
are marked asfinal
. This not only prevents overriding but also assures that any future use of these getters returns the original values accurately.
Final Thoughts
In a Java ecosystem that thrives on flexibility and extensibility, understanding the final
keyword is pivotal. When applied to methods, it can enforce design constraints that ensure stability, better performance, and clearer communication about the intended use of class functionalities.
While final
methods can limit extensibility, they create predictable and controlled behavior, which is invaluable in many scenarios. Always consider the broader implications of marking methods as final
, evaluate the design intentions, and apply this keyword judiciously to produce cleaner and more maintainable code.
For more information about Java keywords and concepts, check out Oracle's Java Documentation.
Happy coding!
Checkout our other articles