Managing Concurrency Issues in Spring Thread Transactions
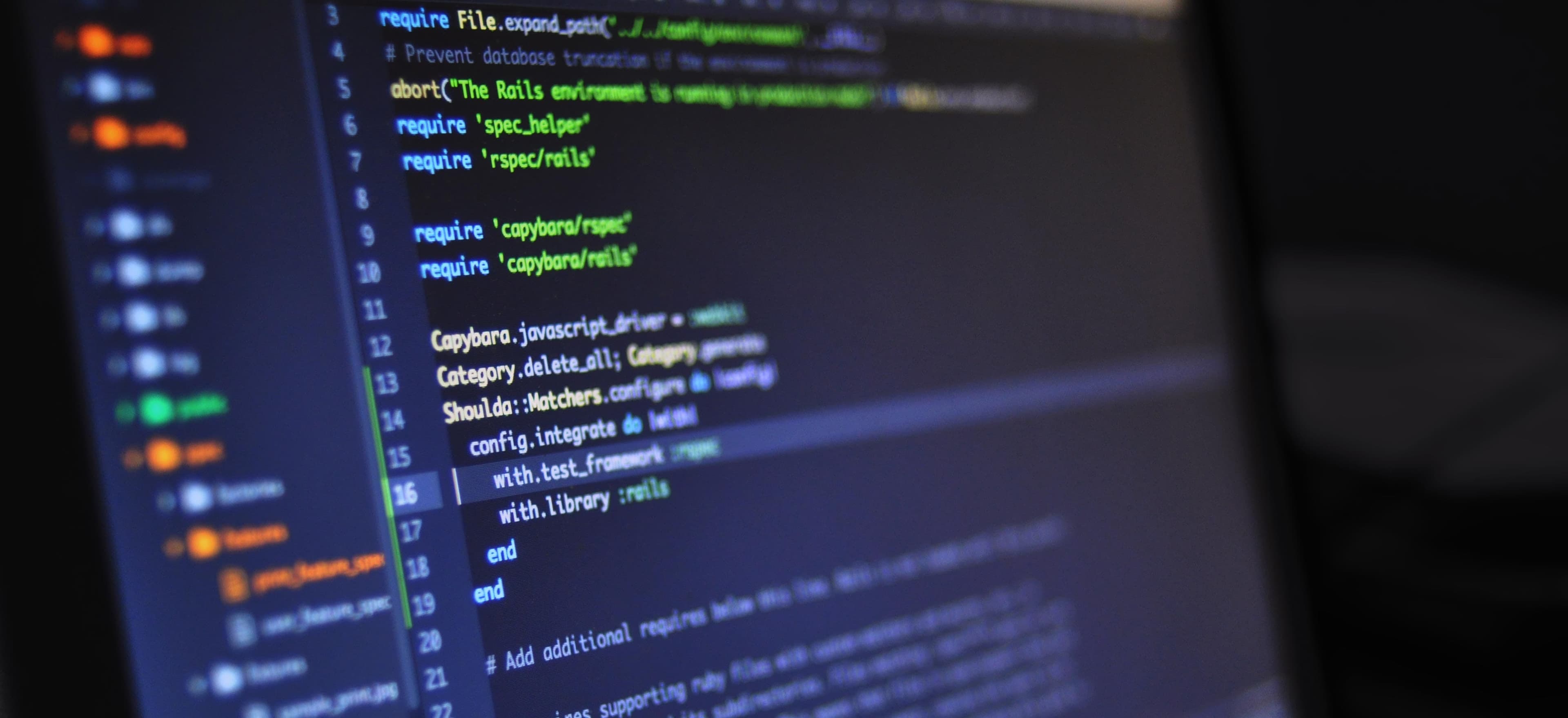
- Published on
Managing Concurrency Issues in Spring Thread Transactions
Concurrency is an inherent part of modern application design, especially in multi-threaded environments where multiple transactions or threads may attempt to read or write to shared resources concurrently. In the Spring Framework, handling transactions becomes even more complex when coupled with multiple threads. In this blog post, we will dive into managing concurrency issues in Spring thread transactions, covering various strategies and best practices.
Understanding Concurrency in Spring
Concurrency refers to multiple threads executing simultaneously. When multiple transactions are involved, issues like dirty reads, lost updates, and phantom reads can occur. To mitigate these issues, Spring provides a robust transaction management framework that operates on a higher level of abstraction.
Types of Concurrency Issues
- Dirty Read: Occurs when one transaction reads uncommitted changes made by another transaction.
- Non-Repeatable Read: Happens when a transaction reads the same row twice, and another transaction modifies it in between the reads.
- Phantom Read: Refers to a situation where new rows are added or removed by another transaction before the current transaction completes.
Transaction Isolation Levels
Spring uses the concept of transaction isolation levels to handle concurrency issues. These isolation levels define how transaction integrity is visible to other transactions. The four primary transaction isolation levels are:
- READ_UNCOMMITTED
- READ_COMMITTED
- REPEATABLE_READ
- SERIALIZABLE
For most applications, READ_COMMITTED is the recommended isolation level, as it prevents dirty reads while maintaining a good balance between performance and integrity.
Setting Up Spring Transactions
Before we can address concurrency issues, we need to set up transactional support in a Spring application. Here’s a basic example using Spring's @Transactional
annotation.
Example Configuration
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.transaction.annotation.EnableTransactionManagement;
import org.springframework.jdbc.datasource.DriverManagerDataSource;
import javax.sql.DataSource;
@Configuration
@EnableTransactionManagement
public class AppConfig {
@Bean
public DataSource dataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/mydb");
dataSource.setUsername("user");
dataSource.setPassword("password");
return dataSource;
}
}
Why Use @EnableTransactionManagement
?
This annotation enables Spring's annotation-driven transaction management capability, allowing us to use @Transactional
easily within our services.
Handling Concurrency in Spring
Optimistic Locking
Optimistic locking is a concurrency control mechanism that allows multiple transactions to complete without locking the database rows. It relies on versioning and is particularly useful when conflicts are rare, thus reducing lock contention.
Example of Optimistic Locking
Let’s see how we can implement optimistic locking using JPA.
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Version;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
@Version
private Long version; // This field enables optimistic locking
// getters and setters
}
Why Use @Version
?
Adding a @Version
field to our entity allows JPA to automatically handle version checks. When an update occurs, if the version in the database does not match the version of the instance being updated, an OptimisticLockException
will be thrown, indicating a conflict.
Transaction Example
Here's an example of a service method that updates a user’s username:
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
@Transactional
public void updateUsername(Long userId, String newUsername) {
User user = userRepository.findById(userId)
.orElseThrow(() -> new ResourceNotFoundException("User not found"));
user.setUsername(newUsername);
userRepository.save(user);
}
}
Benefits of Optimistic Locking
- Minimizes lock contention.
- Encourages collaboration and can yield better performance under low-conflict scenarios.
Pessimistic Locking
Pessimistic locking is a more straightforward approach and is typically used when conflicts are anticipated. With this strategy, we lock the data before modifying it to ensure that no other transactions can affect it.
Example of Pessimistic Locking
Using the @Lock
annotation can define pessimistic locking in Spring Data JPA.
import org.springframework.data.jpa.repository.Lock;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.Query;
import javax.persistence.LockModeType;
public interface UserRepository extends JpaRepository<User, Long> {
@Lock(LockModeType.PESSIMISTIC_WRITE)
@Query("SELECT u FROM User u WHERE u.id = ?1")
User findUserForUpdate(Long id);
}
Why Use PESSIMISTIC_WRITE
?
This mode will lock the row when it is fetched, ensuring that no other transaction can modify it until the current transaction is complete.
Handling Pessimistic Locking in Service
Here’s how you would use the repository method in your service.
@Transactional
public void safeUpdateUsername(Long userId, String newUsername) {
User user = userRepository.findUserForUpdate(userId); // This will lock the user row
user.setUsername(newUsername);
userRepository.save(user);
}
Performance Implications
Pessimistic locking can introduce performance bottlenecks, especially in high-throughput scenarios, as it significantly increases the likelihood of deadlocks and rollbacks.
Using Spring's @Transactional
for Concurrency Control
Utilizing the @Transactional
annotation, you can fine-tune your transaction management. For example, you can specify the isolation level directly in the annotation:
@Transactional(isolation = Isolation.READ_COMMITTED)
public void someTransactionalMethod() {
// your code here
}
Choosing the Right Isolation Level
Selecting an appropriate isolation level depends on your application's requirements. You need to consider the trade-off between consistency and performance. For most scenarios, READ_COMMITTED
provides adequate protection against dirty reads without the performance hit that SERIALIZABLE
brings.
In Conclusion, Here is What Matters
Managing concurrency in Spring thread transactions requires a balanced approach. By leveraging optimistic and pessimistic locking, along with suitable isolation levels, you can effectively handle various concurrency issues. The choice of which mechanism to implement should be based on your application's specific needs and anticipated load characteristics.
For further reading on Spring Transaction Management and concurrency control techniques, consider checking the official Spring documentation. This document provides in-depth insights and additional examples that can enhance your understanding.
Remember, concurrency control is not just about avoiding issues; it’s about optimizing your application for both scalability and performance. Happy coding!
Checkout our other articles