Common Challenges in Multi-Tenant Application Design
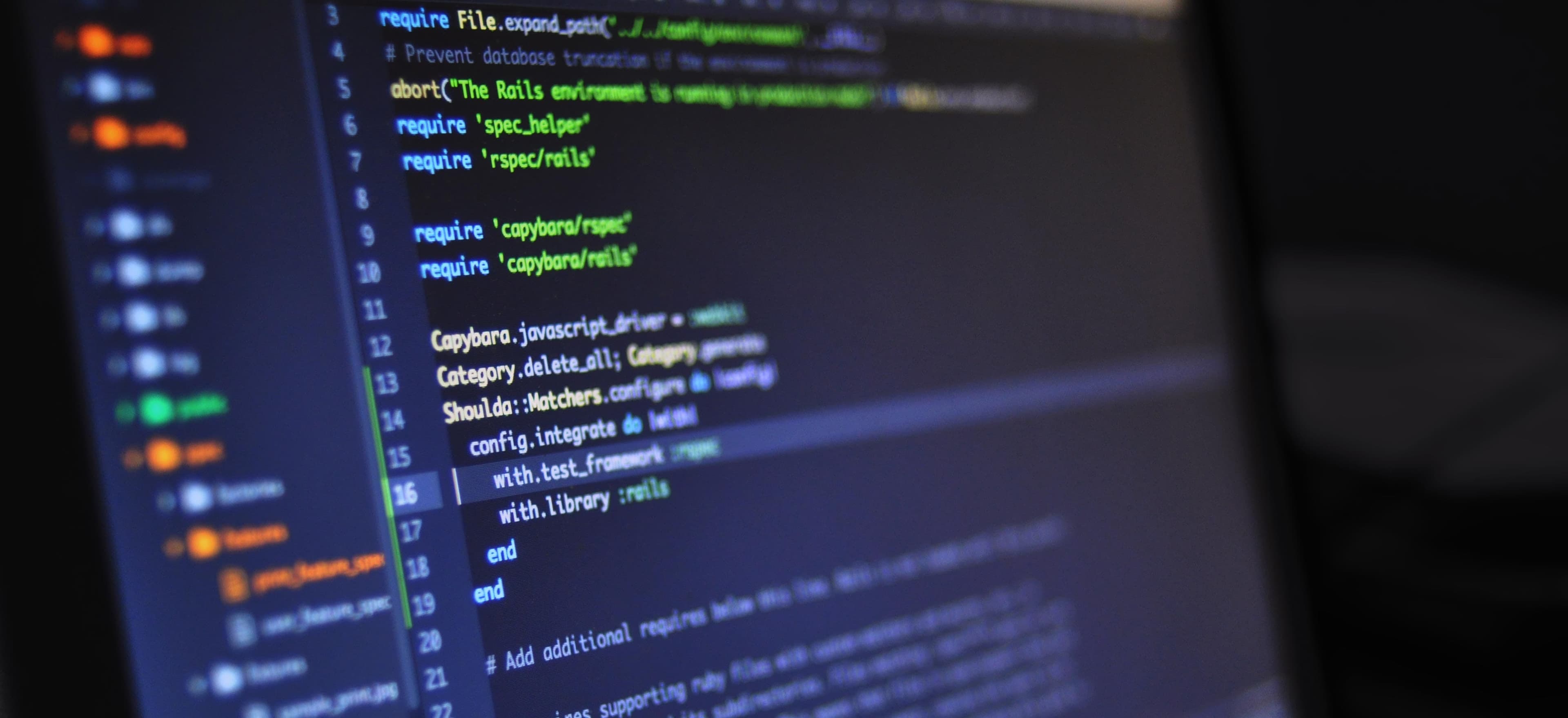
- Published on
Common Challenges in Multi-Tenant Application Design
Designing applications for multi-tenancy is a compelling yet complex endeavor that poses several challenges. Multi-tenancy refers to an architecture where a single instance of an application serves multiple tenants (organizations or user groups). Each tenant's data is isolated and remains invisible to others, enhancing security and data privacy. This architecture can lead to significant cost savings through resource sharing. However, the design process is rife with challenges that developers must consider.
In this blog post, we will delve into common challenges in multi-tenant application design as well as possible solutions, emphasizing best practices to ensure a seamless user experience.
Understanding Multi-Tenant Architecture
Before diving into challenges, it's crucial to grasp the essence of multi-tenant architecture. Developers can employ two primary approaches to multi-tenancy:
- Database-per-Tenant: Each tenant has its database, which can isolate data and enhance performance.
- Shared Database: Tenants share the same database, but each record includes a tenant identifier to differentiate data.
Each approach has its pros and cons. Understanding these will help in addressing the challenges effectively.
1. Data Isolation and Security
Challenge
Data isolation is essential in maintaining the integrity of tenant data. A significant challenge arises in ensuring that one tenant cannot access or manipulate another's data. This is even more crucial in scenarios where sensitive information is involved, such as healthcare or finance.
Solution
Implement role-based access control (RBAC) and encryption. Typically, using robust authentication and authorization mechanisms can mitigate unauthorized access.
Code Snippet: Implementing RBAC in Java
import java.util.HashMap;
import java.util.Map;
public class RoleBasedAccessControl {
private Map<String, String> userRoles = new HashMap<>();
public void assignRole(String user, String role) {
userRoles.put(user, role);
}
public boolean hasAccess(String user, String resource) {
String role = userRoles.get(user);
return role.equals("ADMIN") && resource.equals("SENSITIVE_DATA");
}
}
Why this matters: By establishing roles, we prevent unauthorized access to sensitive data, effectively enforcing data isolation amongst tenants.
2. Performance and Resource Management
Challenge
With multiple tenants sharing resources, performance can become an issue. The demand on shared resources may lead to bottlenecks, thus affecting application performance.
Solution
Load balancing and indexing can help. It is essential to analyze tenant usage patterns and provide resources accordingly. Moreover, caching can significantly enhance performance.
Code Snippet: Implementing Simple Caching
import java.util.Map;
import java.util.HashMap;
public class CachingService {
private Map<String, Object> cache = new HashMap<>();
public void put(String key, Object value) {
cache.put(key, value);
}
public Object get(String key) {
return cache.get(key);
}
}
Why this matters: Caching frequently accessed data improves performance, offering tenants quicker access to necessary resources without degrading the system's overall functionality.
3. Customization Needs
Challenge
Tenants may require different customizations. The challenge lies in balancing customization with maintaining a generic codebase.
Solution
Use feature toggles to manage different functionalities for various tenants without forking your codebase. This approach allows you to decouple features and their implementations.
Code Snippet: Implementing Feature Toggles
import java.util.HashMap;
import java.util.Map;
public class FeatureToggle {
private Map<String, Boolean> features = new HashMap<>();
public void enableFeature(String feature) {
features.put(feature, true);
}
public boolean isFeatureEnabled(String feature) {
return features.getOrDefault(feature, false);
}
}
Why this matters: By clearly managing feature toggles, we can efficiently cater to diverse tenant needs without unnecessary code duplication, which can become a maintenance headache.
4. Scalability
Challenge
As the number of tenants grows, so does the complexity of managing resources. Ensuring scalability while maintaining performance becomes a pressing challenge.
Solution
Utilizing microservices architecture can provide the necessary scalability. Each service can be scaled independently based on different tenant demands.
Why Microservices?
Microservices allow for distributed management of resources. This means one service can handle a spike in requests from one tenant without affecting the performance for others.
5. Compliance and Data Regulations
Challenge
Compliance with data regulations such as GDPR and HIPAA can be daunting. Each tenant may have different compliance requirements that must be addressed in the architecture.
Solution
Implement strict data governance and auditing capabilities, with a clear audit trail of data access and modifications.
Code Snippet: Basic Auditing Implementation
import java.util.ArrayList;
import java.util.List;
public class AuditTrail {
private List<String> auditLogs = new ArrayList<>();
public void logAccess(String tenantId, String resource) {
String log = String.format("Tenant: %s accessed %s", tenantId, resource);
auditLogs.add(log);
}
public void printLogs() {
auditLogs.forEach(System.out::println);
}
}
Why this matters: Keeping an audit trail not only mitigates compliance risks but also provides transparency to tenants regarding data handling.
Closing the Chapter
Designing a multi-tenant application poses a series of intricate challenges. From ensuring data isolation to catering to customization needs, the factors affecting the architecture are vast. By employing best practices like robust access controls, caching mechanisms, feature toggles, microservices architecture, and auditing capabilities, developers can navigate these challenges effectively.
If you are interested in further reading regarding multi-tenancy, consider checking out these resources:
- The Importance of Architecture in Multi-Tenant Applications
- A Guide to Building Multi-Tenant SaaS Applications
Keep iterating and testing your architecture to ensure it meets the current demands of your tenants while also being scalable for future growth. Multi-tenancy offers incredible opportunities but requires diligent design and maintenance strategies. Embrace these challenges, and you'll create a powerful, versatile application.
Checkout our other articles