Mastering JSON String Escapes in Java: Eclipse Tips
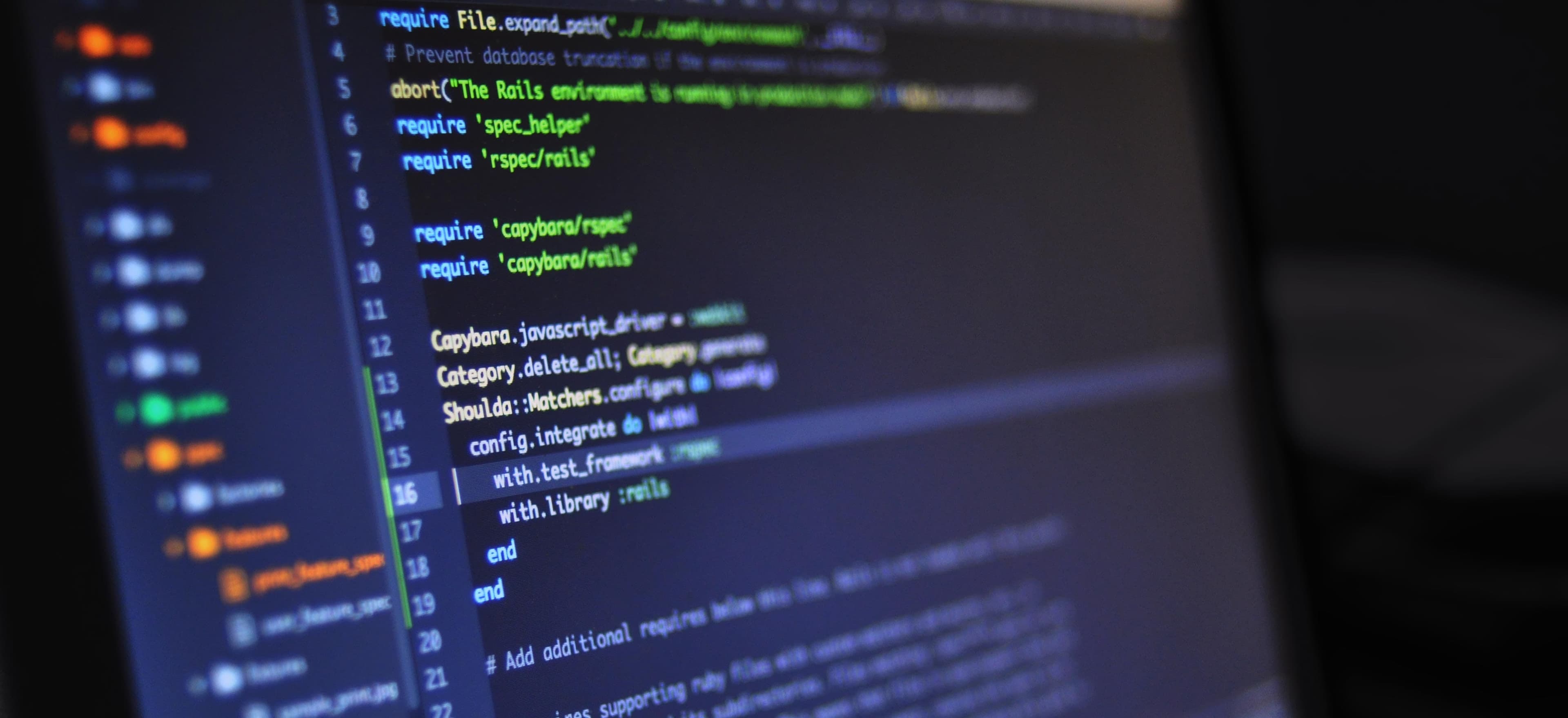
- Published on
Mastering JSON String Escapes in Java: Eclipse Tips
Java developers often work with JSON (JavaScript Object Notation) due to its lightweight data interchange format. JSON's popularity stems from its simplicity and ease of use, but handling JSON string escapes can sometimes pose challenges. In this blog post, we will delve into the intricacies of JSON string escapes in Java, while also incorporating useful tips for using Eclipse, a popular Java Integrated Development Environment (IDE). By the end, you'll be equipped with the knowledge to deftly manage JSON strings in your Java applications.
Understanding JSON
Before we proceed, let’s clarify what JSON is. JSON is primarily used to transmit data between a server and a web application. It consists of key-value pairs and is both human-readable and machine-readable. As it can include various data types, accurately formatting and escaping characters within JSON strings is crucial.
Why Escaping Characters is Important
Certain characters in JSON must be escaped to ensure that the JSON structure remains valid. For instance, characters that have special meanings, such as quotes and backslashes, need to be preceded by a backslash (\
). Here is a simple list of common characters that need escaping in JSON:
- Double quotes:
\"
- Backslash:
\\
- Newline:
\n
- Carriage return:
\r
- Tab:
\t
Missing these escapes can lead to malformed JSON, potentially causing errors in your applications.
Getting Started with JSON in Java
Java provides several libraries to facilitate working with JSON data, including:
- Jackson: A high-performance JSON processing library.
- Gson: A library for converting Java objects to and from JSON.
For this post, we will primarily focus on Jackson. To begin, you will need to add the Jackson dependency to your project. If you are using Maven, add the following to your pom.xml
:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.3</version> <!-- Always check for the latest version -->
</dependency>
Basic Example of JSON Handling with Jackson
Here’s a straightforward example of how to serialize and deserialize JSON data using Jackson.
First, create a Java class that you want to serialize.
public class User {
private String name;
private int age;
public User() {}
public User(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
Next, let's serialize an instance of this class to JSON.
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonExample {
public static void main(String[] args) {
User user = new User("Alice", 30);
ObjectMapper objectMapper = new ObjectMapper();
try {
// Serialize to JSON
String jsonString = objectMapper.writeValueAsString(user);
System.out.println("Serialized JSON: " + jsonString);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Key Takeaways from the Code
- ObjectMapper: This is the main class used for converting Java objects to JSON and vice versa. Its importance cannot be overstated, as it encapsulates all the essential methods.
- writeValueAsString(): This method converts a Java object into its JSON string representation. It handles string escaping automatically.
For the reverse operation—deserializing JSON back into a Java object—you would do the following:
try {
String jsonString = "{\"name\":\"Bob\",\"age\":25}";
// Deserialize from JSON
User userFromJson = objectMapper.readValue(jsonString, User.class);
System.out.println("Deserialized User: " + userFromJson.getName() + ", Age: " + userFromJson.getAge());
} catch (Exception e) {
e.printStackTrace();
}
Handling String Escapes in Java
While Jackson takes care of most escape handling for you, it is necessary to understand how to manage these escapes manually if you work with raw JSON strings.
Using Java's String Escape Sequences
If you need to construct a JSON string manually, remember to escape quotes and backslashes.
String jsonString = "{ \"name\": \"Alice\", \"path\": \"C:\\\\Users\\\\Alice\" }";
Example with Raw String Construction
Here’s how you might construct a JSON string with escaped characters.
String rawJson = "{ \"message\": \"Hello, \\\"world!\\\"\", \"newline\": \"Line1\\nLine2\" }";
try {
User user = objectMapper.readValue(rawJson, User.class);
} catch (Exception e) {
e.printStackTrace();
}
Why String Escapes Matter
Improperly formatted JSON can lead to JsonParseException
. Ensuring that your strings are properly escaped helps maintain the integrity and validity of your JSON data.
Tips for Working in Eclipse
-
Enable Content Assist: Make use of Eclipse's content assist feature (Ctrl + Space) to auto-complete your JSON strings and Java code snippets. This speeds up coding and reduces syntax errors.
-
JSON Editor Plugin: Consider installing a JSON editor plugin for Eclipse. This can help you validate and format JSON data directly within your IDE, simplifying the debugging process.
-
Debugging with Breakpoints: Do not hesitate to use breakpoints to inspect the value of JSON strings in your code. Understanding their structure at runtime can make debugging a lot easier.
Further Reading
For those eager to dive deeper into JSON serialization and parsing in Java, consider exploring the official Jackson documentation and Gson documentation. Both of these resources provide in-depth examples and further nuances about their respective libraries.
Bringing It All Together
Working with JSON in Java doesn't have to be cumbersome. By mastering string escapes and leveraging powerful libraries like Jackson, you can efficiently handle JSON data in your applications. Not only does this ensure that your JSON is valid, but it also allows your applications to remain scalable and maintainable. With Eclipse's helpful features, you can enhance your productivity while writing and debugging your code.
Armed with these insights and tips, you're now better prepared to handle JSON string escapes in Java. Happy coding!
Checkout our other articles