Boost Your Java Code: Top Libraries You’re Missing!
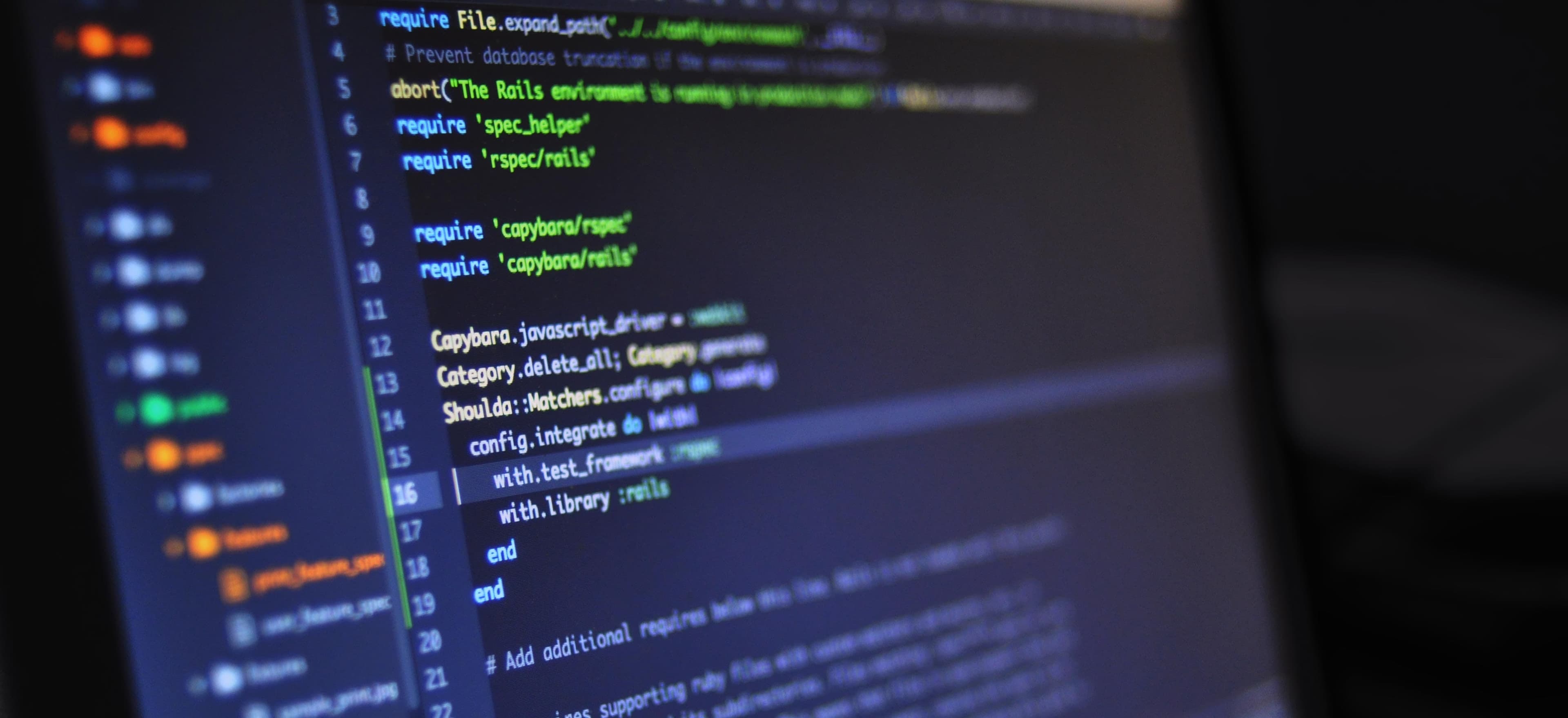
- Published on
Boost Your Java Code: Top Libraries You’re Missing!
Java is an incredibly powerful language, renowned for its versatility and reliability. However, to truly unlock its full potential, it's essential to capitalize on the vast ecosystem of libraries available. Many developers often stick to the standard libraries, but by exploring additional libraries, you can expedite your development process, enhance code quality, and introduce new functionalities seamlessly.
In this blog post, we’ll explore some of the top libraries that can boost your Java applications, along with code snippets demonstrating their practical application. You'll discover why integrating these libraries can take your projects to the next level.
1. Apache Commons
Overview
Apache Commons is a collection of reusable Java components. It’s a staple in the Java ecosystem, providing a rich set of utilities for various programming tasks.
Why Use It?
The library helps reduce the amount of boilerplate code, allowing developers to focus on the core logic of their applications. This can significantly speed up development time and improve code readability.
Example Code
Let’s take a look at the StringUtils class from Apache Commons Lang to perform common string operations.
import org.apache.commons.lang3.StringUtils;
public class StringExample {
public static void main(String[] args) {
String str = " Boost Your Java Code ";
System.out.println("Trimmed String: " + StringUtils.trim(str));
System.out.println("Is Blank: " + StringUtils.isBlank(str));
}
}
Commentary
In the code above, we first import the StringUtils
class. We can then trim whitespace and check if the string is blank, significantly reducing the amount of code you would otherwise have to write.
For more information, check out the Apache Commons documentation.
2. Google Guava
Overview
Guava is a set of core libraries that complement the standard Java libraries and provide enhanced and additional utilities, particularly centered around collections, caching, primitives support, and more.
Why Use It?
Guava enhances the standard Java SDK, making your code more concise and expressive. It also includes caching utilities to improve performance.
Example Code
Here's how you can use Guava's ImmutableSet
.
import com.google.common.collect.ImmutableSet;
public class ImmutableSetExample {
public static void main(String[] args) {
ImmutableSet<String> fruits = ImmutableSet.of("Apple", "Banana", "Cherry");
System.out.println("Fruits: " + fruits);
}
}
Commentary
This code snippet creates an immutable set of fruits. Immutable collections can enhance thread safety and performance since they do not change state after creation.
To dive deeper into Guava, check out the Guava GitHub repository.
3. JUnit
Overview
JUnit is the most popular testing framework for Java. It is widely used for unit testing your code to ensure it performs as expected.
Why Use It?
Testing your code with JUnit allows for quick identification of bugs and validation of your application's logic. This can save hours of debugging later in the development process.
Example Code
Here's a simple test case using JUnit.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
Commentary
In this example, we test the add
method of a Calculator
class to verify that it correctly adds two numbers. Using assertions like assertEquals
, we can ensure accuracy in our methods.
To learn more about JUnit, visit the JUnit 5 user guide.
4. Jackson
Overview
Jackson is a high-performance JSON processor for Java. It is one of the most efficient libraries for converting Java objects to and from JSON.
Why Use It?
Dealing with JSON is a common task in many applications today. Jackson makes it simple and intuitive to handle JSON data, ensuring your application can communicate effectively with various APIs.
Example Code
Let’s serialize and deserialize a simple Java object.
import com.fasterxml.jackson.databind.ObjectMapper;
public class JacksonExample {
public static void main(String[] args) throws Exception {
ObjectMapper objectMapper = new ObjectMapper();
// Convert object to JSON
Person person = new Person("John", 30);
String jsonString = objectMapper.writeValueAsString(person);
System.out.println("JSON: " + jsonString);
// Convert JSON to object
Person personFromJson = objectMapper.readValue(jsonString, Person.class);
System.out.println("Person Name: " + personFromJson.getName());
}
}
class Person {
private String name;
private int age;
// Constructor, getters, and setters
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
}
Commentary
In this example, we create a Person
class and use Jackson to convert it into JSON format and back into a Java object. The ease of serialization and deserialization allows developers to manage data effortlessly.
Check the Jackson homepage for more information.
5. Lombok
Overview
Lombok is a popular Java library that helps reduce boilerplate code, such as getters, setters, and constructors.
Why Use It?
By utilizing annotations, Lombok allows you to write cleaner and more expressive code. This improves maintenance and readability while reducing the risk of common coding errors.
Example Code
Here’s a simple implementation of Lombok.
import lombok.Data;
@Data
public class User {
private String username;
private String password;
public static void main(String[] args) {
User user = new User();
user.setUsername("johnDoe");
user.setPassword("password123");
System.out.println(user.getUsername());
}
}
Commentary
In this code, the @Data
annotation from Lombok generates getter and setter methods for the User
class automatically. It saves you from writing repetitive code.
For more on how Lombok works, visit Project Lombok.
Bringing It All Together
Integrating libraries into your Java projects can significantly enhance your code quality, expedite development time, and introduce new functionalities. From enhancing testing with JUnit to managing JSON data with Jackson, these libraries are invaluable tools in your development toolkit.
As you explore these libraries, consider how each could simplify your workflow or bring added functionality to your projects. Embrace the Java ecosystem, and watch your code soar!
Feel free to share your experiences with these libraries or any others that you find indispensable in the comments below. Happy coding!
Checkout our other articles