Unlocking the Secrets of Java Flight Recorder (JFR) Performance
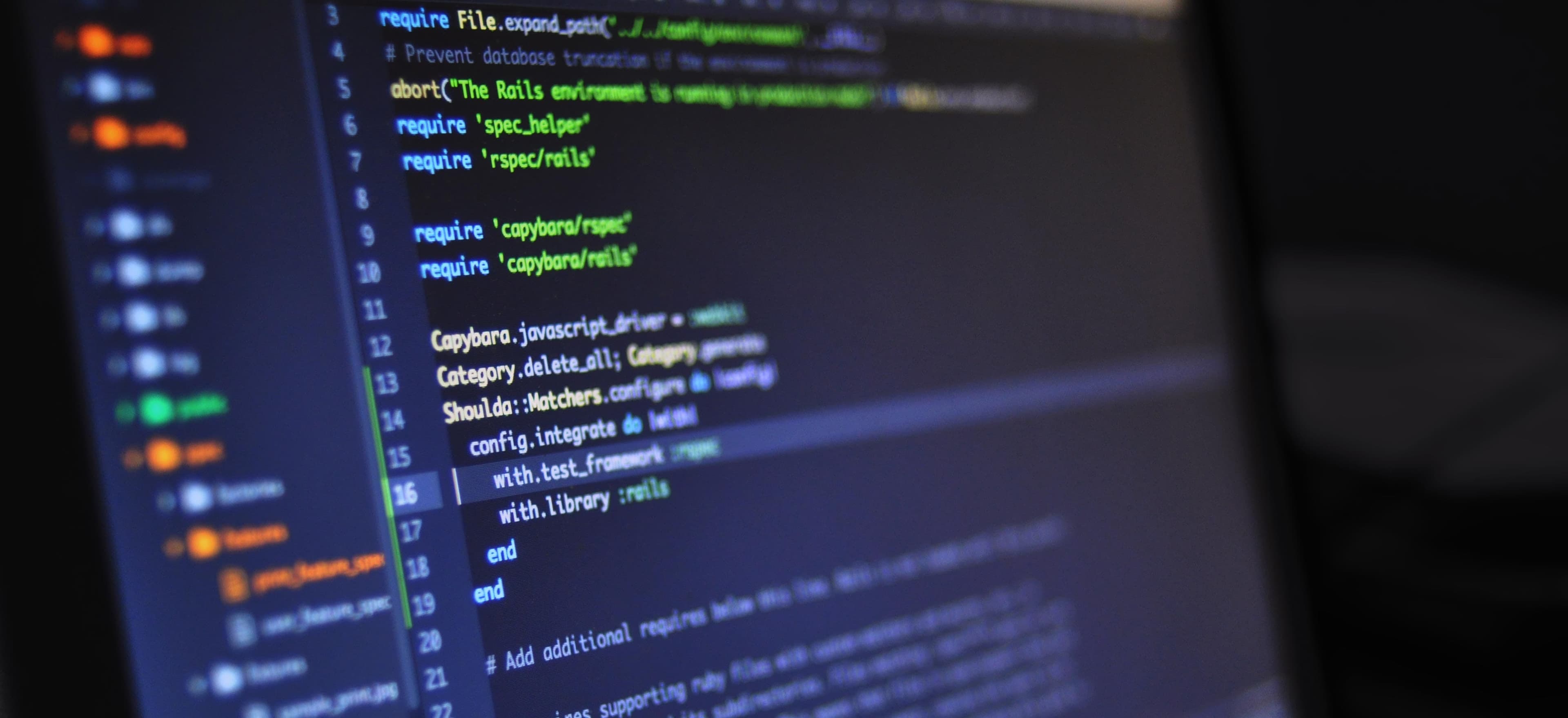
- Published on
Unlocking the Secrets of Java Flight Recorder (JFR) Performance
In the world of Java application development, performance optimization is a concern as critical as writing clean, maintainable code. As applications grow in complexity, identifying performance bottlenecks and analyzing application behavior becomes essential. One powerful tool that can help developers achieve these objectives is Java Flight Recorder (JFR).
JFR is a profiling tool built into the Java Virtual Machine (JVM) that enables developers to monitor and gather detailed information about the application's performance. It provides insights into CPU usage, memory allocation, garbage collection, and thread activity. This blog post will delve into the features of JFR, how to use it effectively, and tips for optimizing your applications.
What is Java Flight Recorder?
Java Flight Recorder is a profiling feature available in the Oracle JDK starting from Version 11. Though previously it was proprietary, it is now open-source and part of the OpenJDK project. The core functionality of JFR is to record and analyze events happening within the JVM while the application is running.
By gathering low-overhead profiling data, JFR allows developers to create detailed recordings of their applications without significantly affecting performance. This data helps identify performance issues and optimizations, making it a critical tool for any Java developer.
Key Features of JFR
-
Low Overhead: JFR is designed to record data with minimal impact on application performance. Unlike traditional profilers, which may cause the application to slow down, JFR records events efficiently.
-
Detailed Event Tracking: JFR allows you to track a wide variety of JVM events, such as method invocations, garbage collection events, thread state changes, and exceptions.
-
Ease of Use: JFR is easy to use, with built-in commands and integrations for monitoring. It can be enabled at startup or dynamically during the application's runtime.
-
Integration with Java Mission Control (JMC): JFR integrates seamlessly with JMC, an interactive tool for viewing and analyzing JFR recordings. JMC provides a graphical interface to explore the data, making it easier to identify patterns and issues.
Getting Started with JFR
To start using JFR, you need to ensure you are using JDK 11 or later. Here’s how to enable and record a JFR session:
Step 1: Enable JFR
You can enable JFR by adding the -XX:StartFlightRecording
option to your JVM command line when you start your Java application.
For example, in the command line, you can use:
java -XX:StartFlightRecording=duration=60s,filename=myrecording.jfr -jar myapp.jar
This command will start a flight recording that runs for 60 seconds and saves the output to a file named myrecording.jfr
.
Step 2: Analyzing JFR Recording
Once you've collected your recording, you can analyze it using Java Mission Control.
-
Download JMC: If you haven’t already, download the Java Mission Control (JMC) from Oracle's website.
-
Open JFR file: Launch JMC and open the
.jfr
file by selecting File > Open. -
Explore the Data: JMC offers various views to analyze the recorded data, including Events, Java Layout, Memory, and Thread performance.
Example Code Snippet
Here's an example of enabling JFR in a codebase directly from the Java API:
import jdk.jfr.Recording;
import jdk.jfr.consumer.RecordingFile;
public class ExampleApp {
public static void main(String[] args) {
// Start a new JFR recording
Recording recording = new Recording();
recording.start();
// Application logic here
performHeavyComputation();
// Stop and save the recording
recording.stop();
recording.dump("myrecording.jfr");
}
public static void performHeavyComputation() {
// Simulating some heavy computation
for (int i = 0; i < 1_000_000; i++) {
double value = Math.sqrt(i);
}
}
}
In this example:
- We create a new
Recording
object, which allows us to control our flight recording programmatically. - Once we've performed our desired computations, we stop the recording and dump it to a file for later analysis.
Optimizing Performance with JFR
1. Monitor CPU Usage
By tracking CPU events, you can identify methods consuming excessive CPU time. You can visualize this via the JMC’s CPU view.
2. Analyze Garbage Collection
Understanding garbage collection behavior is vital to optimizing memory performance. JFR collects data on GC pauses, allowing you to identify frequent full GCs or long GC pauses.
3. Thread Utilization
Analyzing thread usage helps you understand the state of all threads in your application, allowing you to identify thread contention and bottlenecks.
4. Custom Events
JFR allows you to create custom events if you need to observe specific application behavior or performance metrics.
Here’s an example of a custom event:
import jdk.jfr.Description;
import jdk.jfr.Event;
@Description("My Custom Event")
public class MyCustomEvent extends Event {
public void log(String message) {
this.setMessage(message);
this.commit();
}
}
// In your application:
MyCustomEvent event = new MyCustomEvent();
event.log("This event has been logged");
5. Combine with JMH
For more fine-grained performance analysis, consider using Java Microbenchmark Harness (JMH). JFR can record the execution of JMH benchmarks, enabling in-depth analysis of performance metrics.
In Conclusion, Here is What Matters
Java Flight Recorder is an invaluable tool for developers looking to optimize their Java applications. By enabling low-overhead event tracking and analysis, JFR empowers you to uncover hidden performance bottlenecks, improve application responsiveness, and ultimately deliver better software.
By following the practices outlined in this post, you'll advance your proficiency in using JFR. Remember, performance tuning is an iterative process—analyze, adjust, and analyze again.
For further reading, you may explore the official documentation on JFR in OpenJDK and the capabilities of Java Mission Control.
By embedding JFR into your development practices, you're not just fixing problems—you're gaining insight into your application's behavior and boosting its overall performance. Happy profiling!