Balancing Fun and Scalability in Team Projects
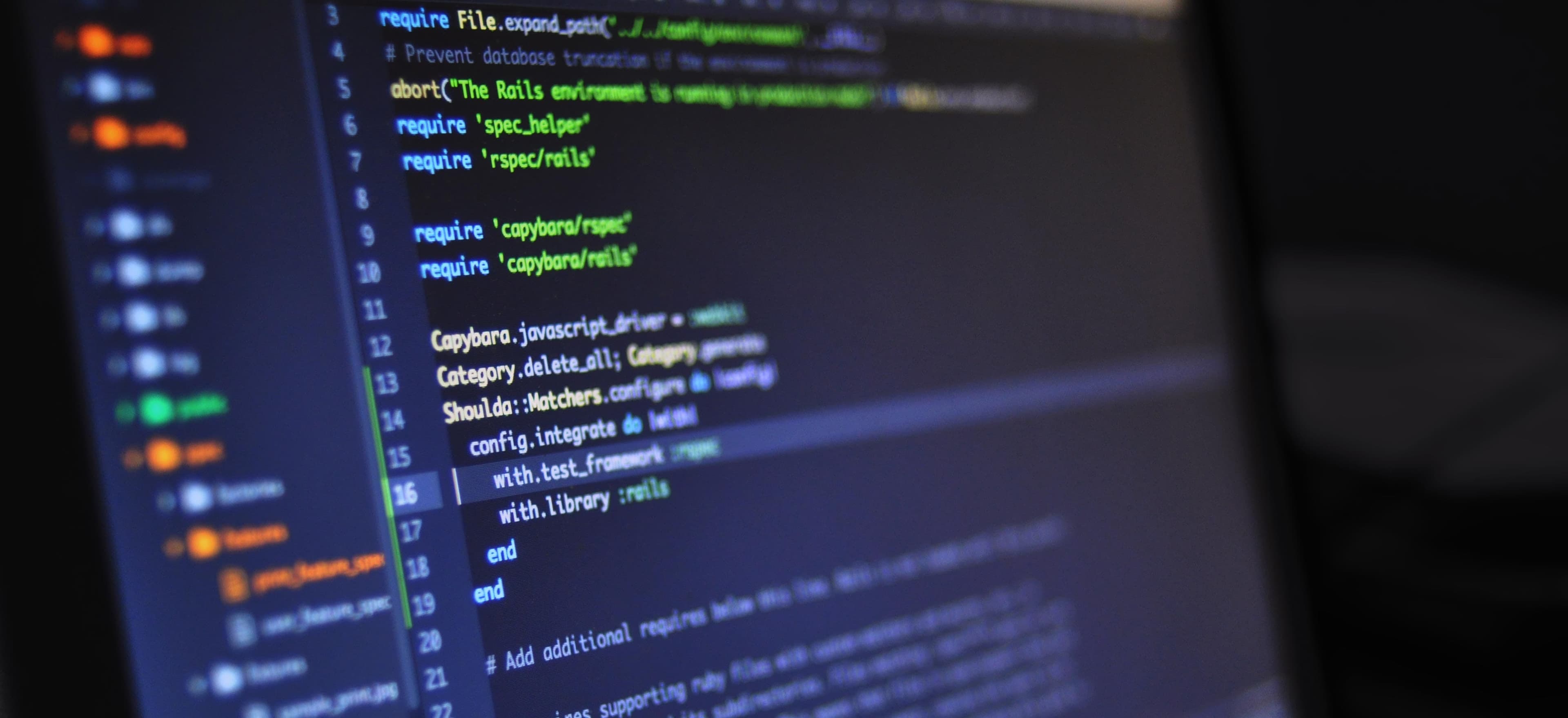
- Published on
Balancing Fun and Scalability in Team Projects
In the ever-evolving landscape of software development, one significant aspect that teams often grapple with is the balance between making work enjoyable and ensuring scalability. While fun fosters creativity and keeps team morale high, scalability ensures that projects can grow efficiently. In this blog post, we'll explore how to maintain this crucial balance, emphasizing practical strategies that your team can implement.
The Importance of Fun in Team Projects
Incorporating fun into team projects is vital for several reasons:
-
Boosted Morale: When team members enjoy their work, they are more motivated. Happy developers are productive developers.
-
Enhanced Creativity: Fun environments encourage out-of-the-box thinking, leading to innovative solutions.
-
Cohesion and Collaboration: Fun activities foster stronger relationships, facilitating better communication and collaboration among team members.
Practical Strategies to Inject Fun
-
Gamification: Introduce game-like elements into your daily tasks. This could be as simple as leaderboards for code reviews or fun challenges that reward creative solutions.
-
Hackathons: Organize regular hackathons where team members can work on passion projects. This grants them freedom while enhancing their skills.
-
Celebrations: Celebrate goals, whether big or small. Simple rewards or team lunches can create an upbeat atmosphere.
Why Scalability Matters
On the other hand, scalability is equally crucial:
-
Growth: As your user base expands, your system must handle increased loads without degradation in performance.
-
Efficiency: Scalable systems often consume resources more efficiently, saving both time and costs.
-
Future-Proofing: A scalable architecture can accommodate future technologies and methodologies, ensuring your product remains relevant.
Strategies for Ensuring Scalability
-
Design Patterns: Applying well-known design patterns helps in creating a structure that accommodates growth and changes effectively. For example, the Singleton pattern ensures that a class has only one instance, controlling access globally.
-
Microservices Architecture: Consider breaking your application into smaller, independent services. Each service can be developed, deployed, and scaled independently.
-
Load Testing: Regularly test your application under heavy loads to identify bottlenecks and ensure performance remains optimal.
Finding the Balance
Now that we've established the importance of both fun and scalability, the real question is: how do we find a balance?
1. Chat about Your Choices
Regular team meetings provide an excellent platform to discuss what elements of your current project are enjoyable and what works against scalability. Encourage candid feedback.
2. Inject Fun into Scalability
Try to incorporate fun into scalability discussions. For instance, why not turn a session on database optimization into a friendly competition? This creates a more engaging atmosphere while solving critical issues.
Example of Balancing Fun and Scalability in Java
To illustrate this balance, let’s consider a Java-based project that utilizes both fun and scalable practices.
Here’s a simple Java class following the Singleton pattern and using gamification concepts:
public class GameScoreManager {
private static GameScoreManager instance;
private int highScore;
// Private constructor to prevent instantiation
private GameScoreManager() {
this.highScore = 0; // Initialize highScore
}
// Method to get the instance of GameScoreManager
public static GameScoreManager getInstance() {
if (instance == null) {
instance = new GameScoreManager();
}
return instance;
}
// Method to update high score
public void updateHighScore(int score) {
if (score > highScore) {
highScore = score;
System.out.println("New high score: " + highScore);
} else {
System.out.println("Try again to beat the high score!");
}
}
}
Why this Code Works
-
Singleton Pattern: This pattern ensures only one instance of the GameScoreManager. This is useful because it prevents conflicting scores in a team setting.
-
Encouraging Engagement: The feedback messages ("New high score" or "Try again") foster a competitive spirit, encouraging fun.
-
Scalability Considerations: In a scenario where you expand this to multiple games, having a centralized score manager allows for easy expansion into multi-game statistics.
Continuous Improvement: Agile Methodology
Many teams benefit from adopting an Agile approach, which inherently supports adapting to both fun and scalability. Agile promotes iterative development, allowing teams to assess how strategies are working and pivot if necessary.
Steps to Implement Agile
-
Sprints: Break the project into smaller time frames, allowing for regular reassessment of team dynamics.
-
Reviews: Use sprint reviews to discuss what fun activities worked and how the project scaled.
-
Retrospectives: Talk about what can be improved in the next sprint.
The Last Word
In conclusion, achieving a balance between fun and scalability within team projects is a dynamic process. Successful teams find ways to integrate enjoyable experiences while maintaining a focus on growth and efficiency. By mixing both elements, teams can foster innovation while building robust applications.
For further reading on design patterns, consider checking out Refactoring Guru for great examples. Also, research Agile methodologies at Agile Alliance, which can provide insights into achieving this balance in your projects.
Remember, creating an enjoyable work environment doesn't compromise scalability; instead, it enhances it. So, keep experimenting, stay engaged, and most importantly, have fun while you build!
Checkout our other articles