Top Strategies to Prevent Memory Leaks in Java Apps
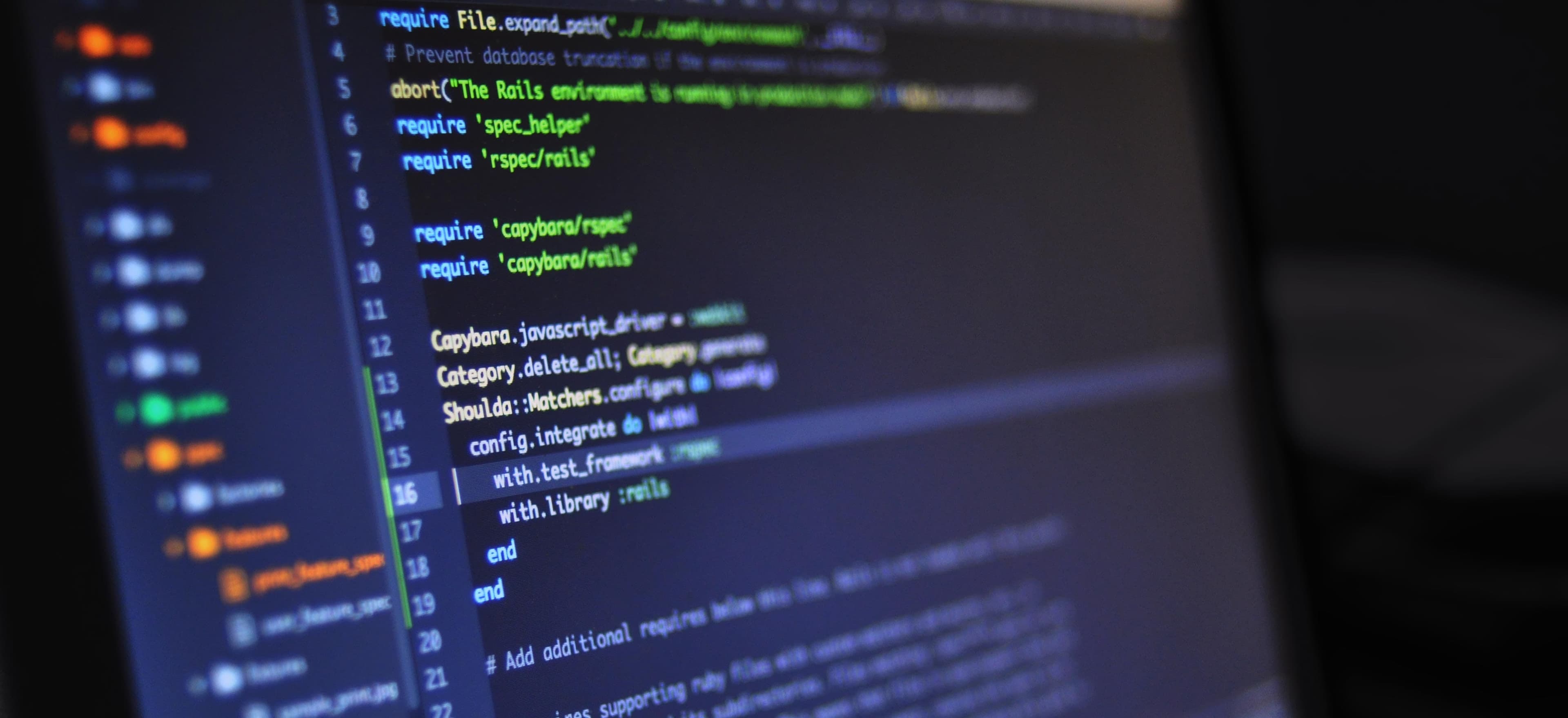
- Published on
Top Strategies to Prevent Memory Leaks in Java Apps
As developers, managing memory effectively in Java applications is crucial. A memory leak occurs when the application unintentionally retains references to objects that are no longer needed, preventing the Java Garbage Collector from reclaiming that memory. This can lead to increased memory consumption and eventually result in application crashes. In this post, we'll explore some of the top strategies to prevent memory leaks in Java applications.
Understanding Memory Management in Java
Before diving into the strategies, it’s essential to have a solid understanding of how memory management works in Java. Java employs automatic garbage collection, which means developers don't need to manually manage memory allocation and deallocation as in languages like C or C++. However, this doesn’t mean we can ignore memory management.
Key Concepts
- Garbage Collector (GC): The component responsible for reclaiming memory by removing objects that are no longer reachable in the program.
- Reference Types: Java offers several types of references—strong, weak, soft, and phantom—which influence how the garbage collector treats them.
For a more in-depth look at how Java handles memory, you may reference the official Oracle documentation.
Top Strategies to Prevent Memory Leaks
1. Use Weak References for Cache
When building caches, a common practice is to use WeakReference
objects. These references allow the garbage collector to collect the object when memory is low, thus preventing memory leaks.
import java.lang.ref.WeakReference;
import java.util.HashMap;
import java.util.Map;
public class Cache {
private Map<String, WeakReference<Object>> cache = new HashMap<>();
public void put(String key, Object value) {
cache.put(key, new WeakReference<>(value));
}
public Object get(String key) {
WeakReference<Object> weakReference = cache.get(key);
return weakReference != null ? weakReference.get() : null;
}
}
Why this works: By using WeakReference
, the Cache
class allows objects to be garbage collected when necessary, preventing unnecessary retention of large amounts of memory.
2. Avoid Static References
Static fields can unintentionally hold onto objects for as long as the class is loaded, leading to memory leaks. Use static fields judiciously, and consider encapsulating them within appropriate lifetimes.
public class UserSession {
private static List<User> activeUsers = new ArrayList<>();
public static void addUser(User user) {
activeUsers.add(user); // Memory leak if user is not removed
}
public static void clearUsers() {
activeUsers.clear(); // Explicitly allowing garbage collection
}
}
Why this matters: If activeUsers
holds references to user sessions forever, it keeps those User objects alive even when they should be eligible for garbage collection.
3. Properly Clean Up Event Listeners
Event listeners can also cause leaks when they keep references to objects. Always ensure to unregister listeners when an object is no longer needed.
public class EventGenerator {
private List<EventListener> listeners = new ArrayList<>();
public void addListener(EventListener listener) {
listeners.add(listener);
}
public void removeListener(EventListener listener) {
listeners.remove(listener);
}
public void notifyListeners() {
for (EventListener listener : listeners) {
listener.onEvent();
}
}
}
Why this is critical: If listeners are not removed, they may retain references to the objects that no longer need to exist. Regular cleanup helps to mitigate this risk.
4. Implement Finalize
Method Wisely
The finalize
method can be overridden to perform cleanup actions before an object gets garbage collected. However, it is generally discouraged in modern Java as it can lead to further complications and is no longer guaranteed to be called promptly.
Note: Instead, prefer using try-with-resources
or implementing the AutoCloseable
interface when dealing with resources such as file streams or database connections.
public class Resource implements AutoCloseable {
@Override
public void close() {
// Cleanup code here
}
}
Consideration: Using finalize
should be avoided when there are better alternatives for resource management.
5. Use Profiling Tools
Utilize tools like VisualVM, Eclipse Memory Analyzer, or JProfiler to help detect memory leaks. These tools can aid in visualizing memory usage and understanding which objects are consuming memory unnecessarily.
- VisualVM: A powerful tool for monitoring and profiling Java applications.
- Eclipse Memory Analyzer: A tool for finding memory leaks and reducing memory consumption.
These tools can produce heap dumps, allowing you to inspect objects and their references, making it easier to identify potential leaks.
6. Manage Thread Lifecycles Properly
Thread management in Java can also lead to memory leaks if not handled correctly. Always clean up thread usage by terminating threads that are no longer needed.
public class WorkerThread extends Thread {
@Override
public void run() {
// Perform work here
// Ensure proper cleanup when work is done
Thread.currentThread().interrupt();
}
}
Why proper management counts: Unmanaged threads can live longer than intended, holding onto resources and causing leaks.
7. Leverage Strong Encapsulation
Encapsulate your objects and their relationships. By tightly managing how objects are referred to, you minimize the chances of inadvertently keeping references that can lead to leaks.
public class OrderProcessor {
private List<Order> orders = new ArrayList<>();
public void addOrder(Order order) {
orders.add(order);
}
public void clearOrders() {
orders.clear(); // Prevents memory retention
}
}
Significance: Strong encapsulation ensures that objects do not remain in memory longer than necessary.
A Final Look
Memory leaks can significantly degrade the performance of Java applications. By implementing memory management best practices—using weak references for caches, removing unused static fields, cleaning up event listeners, avoiding finalize
, profiling tools, managing threads efficiently, and leveraging encapsulation—developers can keep their applications running smoothly and efficiently.
For further reading on Java memory management, check out Java Performance: The Definitive Guide for deeper insights into optimizing Java applications.
By employing these strategies, you not only enhance the performance of your Java applications but also gain better control over resource management. Happy coding!
Checkout our other articles