Unlocking Performance: Reducing Lock Contention in Concurrency
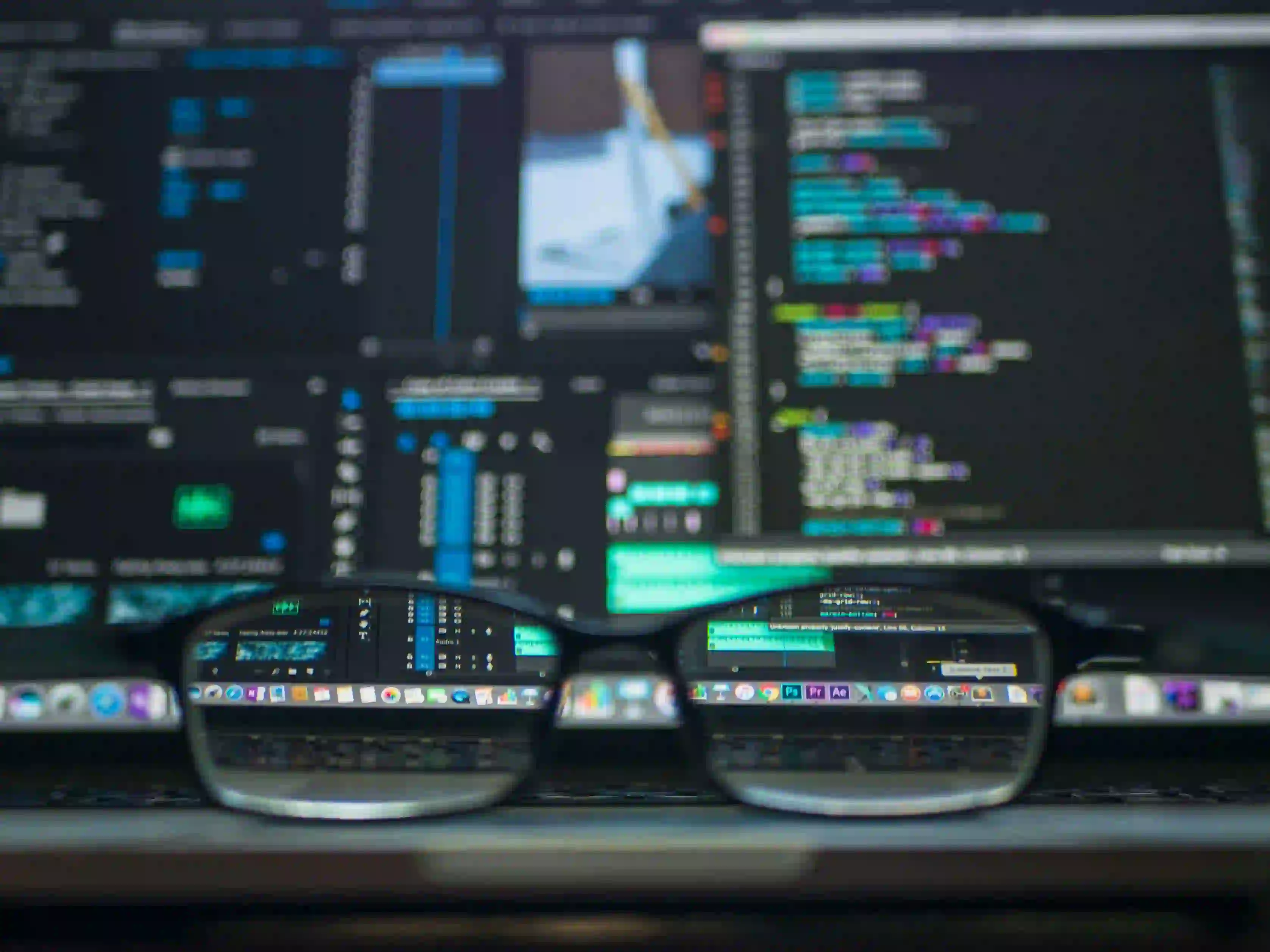
Unlocking Performance: Reducing Lock Contention in Concurrency
Concurrency in Java is a fundamental aspect of developing high-performance applications. However, it is also one of the trickiest areas to handle, especially when dealing with lock contention. This blog post aims to explore lock contention in Java concurrency, why it matters, and various techniques to reduce it.
Understanding Lock Contention
Lock contention occurs when multiple threads attempt to acquire the same lock simultaneously. Imagine a busy restaurant where several customers try to enter through a single door. The more customers there are, the slower the entry process becomes. In programming, excessive lock contention can lead to inefficient thread utilization and can degrade application performance significantly.
Why Lock Contention Matters
- Performance Issues: High lock contention can lead to increased wait times for threads, causing latency in critical operations.
- Thread Starvation: Threads may be blocked indefinitely if other threads hold locks for a prolonged duration.
- Deadlocks: In extreme cases, lock contention can lead to deadlock situations where two or more threads are unable to proceed.
Understanding the causes of lock contention is critical for effective system design and optimization strategies.
Locking Mechanisms in Java
Java provides several locking mechanisms, including intrinsic locks (using synchronized
) and explicit locks (using Lock
interface from the java.util.concurrent.locks
package).
Intrinsic Locks
The simplest form of locking is by using the synchronized
keyword. This mechanism locks the entire method or block:
public synchronized void safeMethod() {
// critical section
}
Using intrinsic locks is simple but can lead to high contention as it locks the entire method scope. For finer control, Java provides the Lock
interface.
Explicit Locks
Explicit locks like ReentrantLock
provide more features, such as try-lock capabilities, fair ordering, and more granular control. Here's a basic example:
import java.util.concurrent.locks.ReentrantLock;
public class Counter {
private final ReentrantLock lock = new ReentrantLock();
private int count = 0;
public void increment() {
lock.lock(); // Acquire the lock
try {
count++;
} finally {
lock.unlock(); // Always unlock in the finally block
}
}
}
The try-finally
block ensures that the lock is released even if an exception occurs while performing the operation. This pattern minimizes the risk of lock leaks.
Strategies to Reduce Lock Contention
Achieving lower lock contention involves a mix of software design strategies and specific programming techniques. Here are several effective approaches:
1. Fine-Grained Locking
Instead of locking entire resources, consider breaking them down into smaller parts. This permits multiple threads to operate concurrently on different parts.
public class DataContainer {
private final ReentrantLock[] locks = new ReentrantLock[10]; // One lock for each partition
private final int[] data = new int[10];
public DataContainer() {
for (int i = 0; i < locks.length; i++) {
locks[i] = new ReentrantLock();
}
}
public void update(int index, int value) {
if (index < 0 || index >= data.length) {
throw new IndexOutOfBoundsException();
}
locks[index].lock(); // Lock for specific index
try {
data[index] = value;
} finally {
locks[index].unlock();
}
}
}
In this example, each data partition has its lock, allowing independent updates without interference.
2. Lock-Free Data Structures
Java provides some thread-safe data structures such as ConcurrentHashMap
and ConcurrentLinkedQueue
that utilize non-blocking algorithms. These structures allow safe access to shared data without traditional locks.
import java.util.concurrent.ConcurrentHashMap;
public class Cache {
private final ConcurrentHashMap<String, String> cache = new ConcurrentHashMap<>();
public void put(String key, String value) {
cache.put(key, value);
}
public String get(String key) {
return cache.get(key);
}
}
Using ConcurrentHashMap
enables concurrent reads and writes without locking, drastically reducing contention.
3. Reduce Lock Hold Time
Keep the critical section as short as possible. If locks are held for long periods, they block other threads unnecessarily.
public void processItem(Item item) {
lock.lock();
try {
if (item.isValid()) {
// Do processing, but keep this brief.
item.process();
}
} finally {
lock.unlock();
}
}
By keeping processing concise and effective, you improve throughput, as locks are released sooner.
4. Use Read/Write Locks
For scenarios where reads significantly outnumber writes, ReadWriteLock
can be beneficial. It allows multiple readers but gives exclusive access to writers.
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class ReadWriteMap {
private final ReentrantReadWriteLock rwLock = new ReentrantReadWriteLock();
private final ConcurrentHashMap<String, String> map = new ConcurrentHashMap<>();
public String read(String key) {
rwLock.readLock().lock();
try {
return map.get(key);
} finally {
rwLock.readLock().unlock();
}
}
public void write(String key, String value) {
rwLock.writeLock().lock();
try {
map.put(key, value);
} finally {
rwLock.writeLock().unlock();
}
}
}
This pattern enables higher read concurrency, thus minimizing contention.
5. Task Distribution
Segregate tasks that require lock access from those that do not. Use message-passing or asynchronous processing where feasible.
public class AsyncProcessor {
private final ExecutorService executor = Executors.newFixedThreadPool(2);
public void process(Task task) {
executor.submit(() -> {
// Non-locking logic
task.perform();
});
}
}
Utilizing an ExecutorService
for asynchronous processing helps smoothen bottlenecks associated with locks.
To Wrap Things Up
Reducing lock contention is essential in creating high-performance, scalable Java applications. By employing fine-grained locking, leveraging lock-free data structures, minimizing the hold time of locks, using suitable locking mechanisms, and distributing tasks efficiently, you can vastly improve application responsiveness.
With challenges like lock contention addressed, you can focus on writing more robust and efficient applications. If you're looking to dive deeper into building concurrent applications in Java, I recommend reading the official Java Concurrency documentation here.
For further reading on advanced concurrency patterns, consider exploring "Java Concurrency in Practice" by Brian Goetz, which is an excellent resource for tackling complex concurrency problems in Java.
By understanding and implementing these strategies effectively, you can unlock the potential of concurrent programming while maintaining system performance and stability.