Navigating the Pitfalls of Serverless Architecture
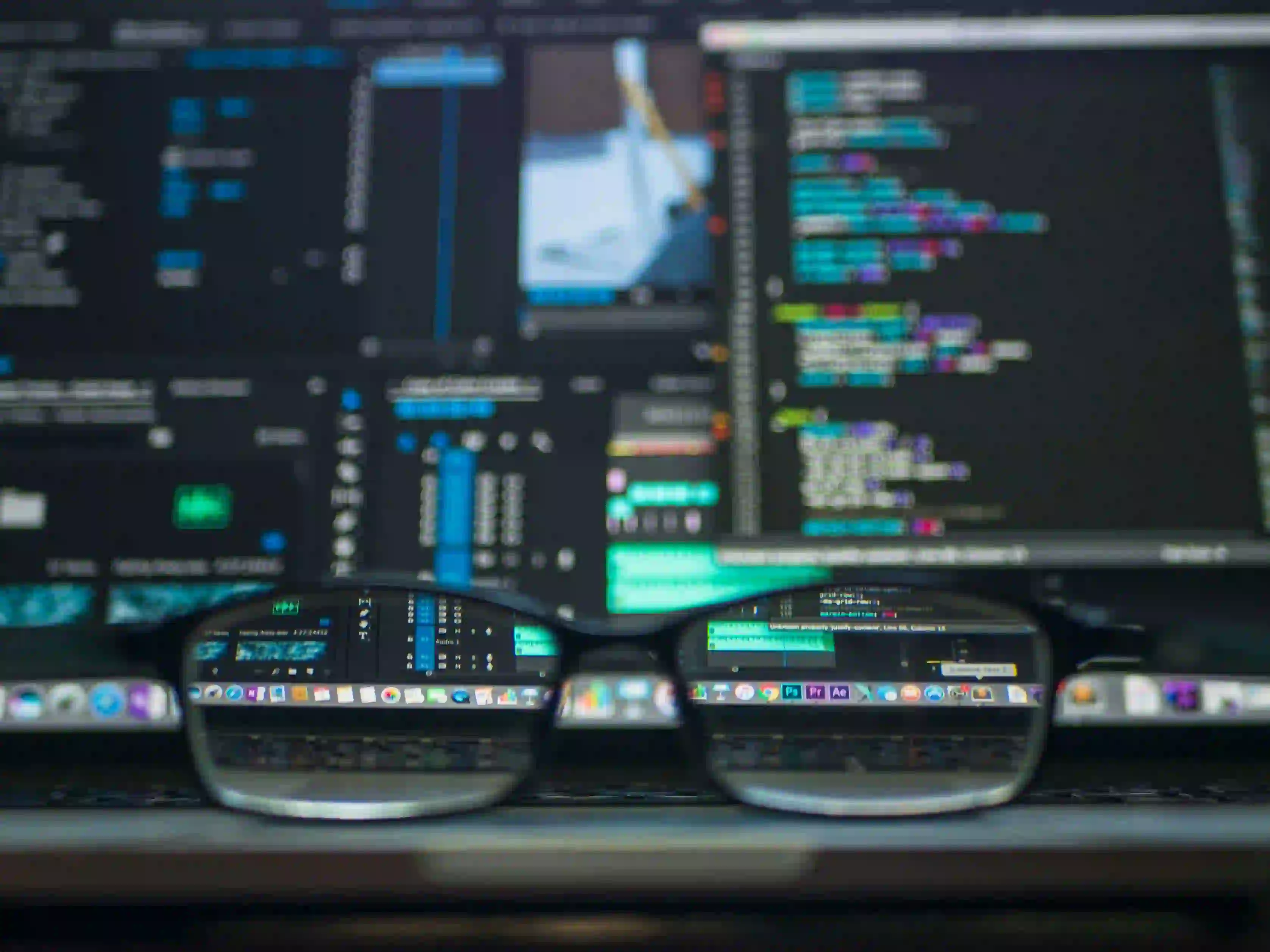
Navigating the Pitfalls of Serverless Architecture
Serverless architecture is a game changer for modern application development. It allows developers to focus more on code and less on the underlying infrastructure. Yet, it is not without its pitfalls. In this blog post, we will explore some common challenges faced when adopting serverless architecture and how to mitigate them.
Diving Into the Subject to Serverless Architecture
Serverless architecture refers to a cloud computing model where the cloud provider dynamically manages the allocation and provisioning of servers. This architecture allows developers to build and run applications without worrying about server management. Instead, they can focus on writing code.
Serverless platforms, such as AWS Lambda, Azure Functions, and Google Cloud Functions, allow developers to deploy code in response to events, automatically scaling as per demand. However, the ease of use comes with its own set of challenges.
Understanding the Pitfalls
As with any new technology, these pitfalls can be categorized in various ways. Let’s delve into some common challenges that developers face when adopting serverless architecture.
1. Cold Starts
When a serverless function is invoked after being idle for a period, it might take longer to respond due to the time it takes to initialize a new instance. This is commonly referred to as a "cold start."
Solution
To mitigate cold starts, consider the following:
-
Keep Functions Lightweight: Maintain minimal code within each function. The smaller the deployment, the quicker the cold start.
-
Warm-Up Strategies: Schedule periodic invocations of your functions to keep them “warm.” Simple cron jobs can serve this purpose.
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
public class WarmUpFunction implements RequestHandler<String, String> {
@Override
public String handleRequest(String input, Context context) {
return "AWake up, I'm warm!";
}
}
2. Vendor Lock-In
Using serverless architecture often means relying on specific cloud services and APIs, leading to vendor lock-in. Migrating applications to another service can become cumbersome.
Solution
Opt for multi-cloud strategies where feasible. Use standardized APIs and frameworks to build your services. Containerization technologies like Docker can help abstract application dependencies from the underlying infrastructure.
3. Debugging Challenges
Debugging serverless functions can be more challenging than traditional applications due to their statelessness and distributed nature.
Solution
Implement proper logging and monitoring. Utilize tools such as AWS CloudWatch, Azure Application Insights, or Google Cloud Logging to gather relevant logs and metrics.
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
public class ExampleFunction implements RequestHandler<String, String> {
@Override
public String handleRequest(String input, Context context) {
context.getLogger().log("Input: " + input);
return "Processed input: " + input;
}
}
This snippet demonstrates how to log incoming requests, making it easier to trace issues later on.
4. Security Concerns
Serverless applications are vulnerable to various security threats, including overly permissive IAM roles and insecure APIs.
Solution
-
Least Privilege Principle: Assign the least privilege necessary to your functions. Use IAM roles wisely.
-
API Gateway: Always route your functions through a secured API Gateway that checks authentication and authorization.
5. Performance Issues
Latency can become a challenge, especially if functions require multiple invocations to complete a task. This can lead to performance bottlenecks.
Solution
Optimize the design of your serverless functions:
-
Combine Functions: When possible, combine several smaller functions into a single function. This reduces the number of invocations.
-
Asynchronous Processing: Consider asynchronous processing using queues or event streams, such as AWS SQS or Kinesis, to handle large workloads without blocking.
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
public class EventDrivenFunction implements RequestHandler<String, String> {
@Override
public String handleRequest(String input, Context context) {
// Process input asynchronously
return "Input is being processed!";
}
}
This example shows how you might declare that processing is ongoing without blocking, improving overall performance.
6. Monitoring and Observability
With applications running across numerous isolated functions, it often becomes complicated to monitor system performance effectively.
Solution
Implement comprehensive monitoring using tools like ELK Stack, Datadog, or cloud-native solutions (like AWS X-Ray) for tracing calls.
7. Testing Difficulties
Traditional testing frameworks may not be designed to work well with serverless functions, leading to inconsistent test environments.
Solution
Utilize local simulation tools, such as AWS SAM or Serverless Framework, that allow you to test your functions locally before deploying them.
# Starting the local test server using AWS SAM
sam local start-api
This command launches a local API Gateway, enabling you to invoke your function as if it's running on AWS.
8. Complexity in Orchestration
Orchestrating workflows across various serverless functions can be complex and lead to challenges in error handling and retries.
Solution
Use serverless orchestration services such as AWS Step Functions or Azure Durable Functions that allow you to visualize workflows and manage states effectively.
Final Thoughts
Serverless architecture is undeniably powerful and liberating for developers, but it is vital to recognize and plan for its pitfalls. From cold starts to orchestration complexities, there are multiple aspects to address.
To ensure successful serverless implementations, keep your functions efficient, secure, and maintainable. Utilize proper logging to facilitate debugging and carefully consider vendor strategies to avoid lock-in.
Additionally, investing in monitoring and orchestration tools can significantly ease the management of a serverless environment. Embrace the advantages, but navigate thoughtfully.
By understanding these challenges and proactively addressing them, you can effectively harness the power of serverless architecture for your applications.
For further reading, you may want to check out AWS’s guide to serverless application development and MIT’s principles of cloud computing for deeper insights.
Happy coding!