Why Parallel Testing Can Save You Time and Money
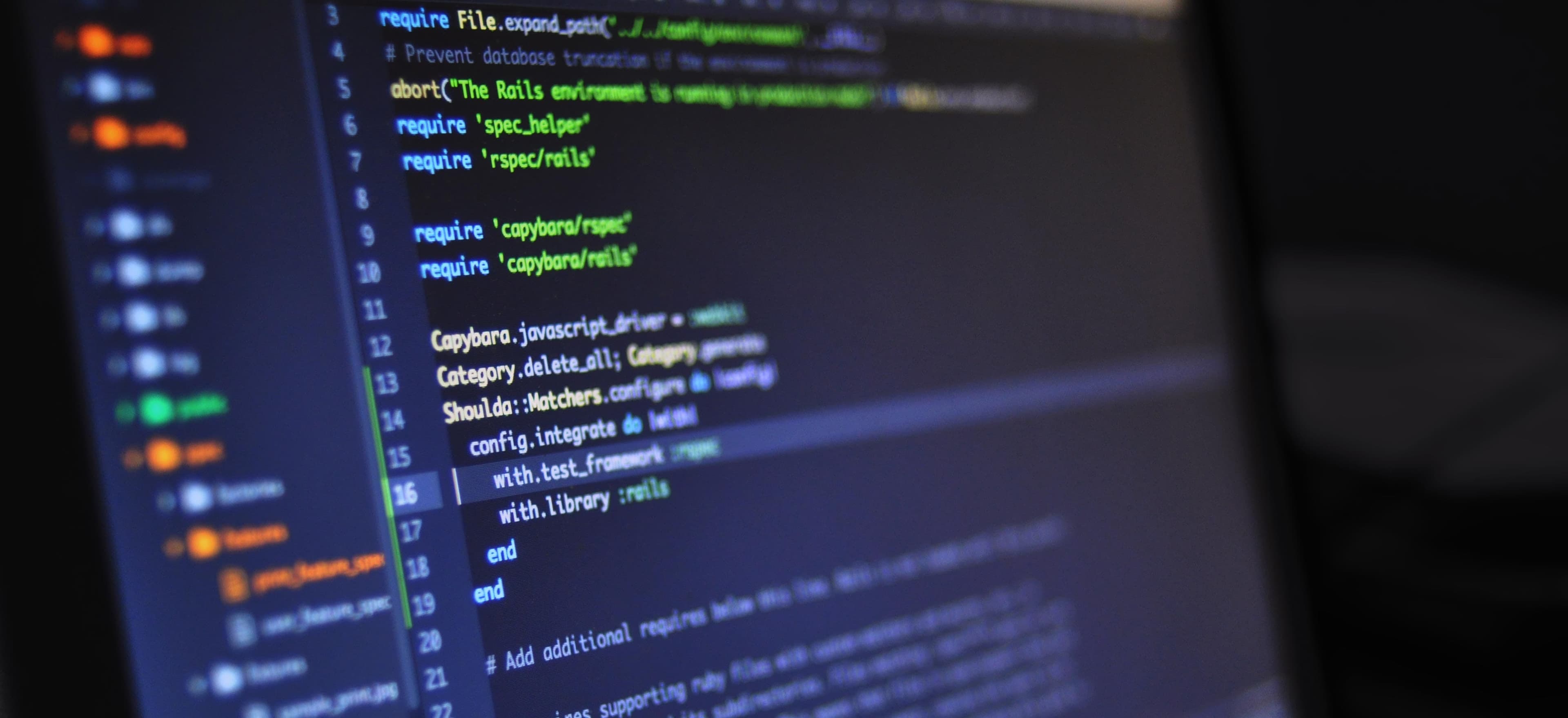
- Published on
Why Parallel Testing Can Save You Time and Money
In today’s fast-paced development environment, software testing remains a critical component of the software development lifecycle. Traditionally, testing has been conducted sequentially, which can often lead to bottlenecks. However, parallel testing has emerged as a game-changer. In this article, we will explore what parallel testing is, how it differs from traditional testing methods, and why it can save you both time and money.
What Is Parallel Testing?
Parallel testing involves executing multiple tests at the same time across different environments or configurations. This contrasts with serial testing, where tests are executed one after another. By running tests concurrently, development teams can identify issues more quickly, improve turnaround times, and enhance the overall quality of the software product.
Here's a simple illustration of the difference between the two methods:
- Sequential Testing:
- Test A runs.
- Once Test A completes, Test B starts.
- Parallel Testing:
- Test A and Test B run simultaneously.
Benefits of Parallel Testing
-
Faster Feedback: By running tests in parallel, teams can receive feedback more quickly. This allows for a prompt response to any issues, facilitating faster iterations.
-
Cost Efficiency: Less time spent on testing means reduced resource allocation and faster time to market, leading to potential cost savings.
-
Increased Test Coverage: Parallel testing allows teams to cover more test cases a day, increasing the overall test coverage.
-
Improved Morale: Teams feel more accomplished when they see results faster, which contributes to enhanced productivity and employee satisfaction.
Parallel Testing vs. Traditional Testing
To further illustrate the advantages of parallel testing, let’s take a look at a comparative example.
In traditional testing, if a new feature is introduced, it could take multiple days to run through the entire test suite. However, with parallel testing, multiple features can be tested simultaneously, significantly reducing the time required.
Here’s a simplified code snippet to demonstrate how you can implement parallel testing using Java's ExecutorService
:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ParallelTester {
public static void main(String[] args) {
// Create a fixed thread pool for parallel execution
ExecutorService executor = Executors.newFixedThreadPool(3);
// Submit test tasks to the executor
executor.submit(() -> runTest("Test A"));
executor.submit(() -> runTest("Test B"));
executor.submit(() -> runTest("Test C"));
// Shutdown the executor
executor.shutdown();
}
public static void runTest(String testName) {
System.out.println(testName + " is running...");
// Simulated test logic here, which could take varying amounts of time
try {
Thread.sleep(2000); // Simulates test execution time
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
System.out.println(testName + " completed.");
}
}
Explanation of the Code
-
ExecutorService: This Java interface is part of the
java.util.concurrent
package. It allows for the management of a pool of threads, making it easier to execute tasks asynchronously. -
Fixed Thread Pool: Here,
newFixedThreadPool(3)
creates a pool that can execute 3 threads simultaneously. This is crucial for parallel testing as it dictates the level of concurrency. -
Submitting Tasks: The
submit()
method is used to assign tasks to the executor, allowing Test A, Test B, and Test C to run concurrently. -
Shutdown: Finally, the
shutdown()
method is called to clean up after the tests have been completed.
Implementing Parallel Testing: Best Practices
While parallel testing offers tremendous benefits, it is essential to implement it correctly. Here are some best practices:
-
Isolation: Ensure that tests are isolated to avoid shared state issues. Each test should have its own data and environment.
-
Resource Management: Monitor resource consumption to avoid overloading servers or database connections, which can lead to inaccurate results.
-
Error Logging: Implement robust error logging to ensure that any failures during parallel execution are easily traceable.
Tools for Parallel Testing
Several tools can facilitate parallel testing in Java, enhancing efficiency and effectiveness. Here are a few notable ones:
-
JUnit: This widely used testing framework now supports parallel test execution with JUnit 5.
-
TestNG: TestNG is another powerful testing tool specifically designed for parallel execution, offering enhanced features like data-driven testing.
-
Selenium Grid: For web applications, Selenium Grid can be utilized to run tests in parallel across different browser instances and operating systems.
For more details on how you can set up Selenium Grid for parallel testing, visit Selenium Documentation.
Real-World Case Studies
Case Study 1: E-commerce Platform
A leading e-commerce platform faced bottlenecks during peak sales hours. Their testing was sequential, delaying updates and new feature launches. After moving to parallel testing, they managed to reduce the regression testing cycle from two days to just six hours. This led to a quicker deployment of features, greatly enhancing their competitive edge.
Case Study 2: Banking Application
A banking application implemented parallel testing to ensure regulatory compliance on new updates. With parallel execution, they tested multiple scenarios simultaneously, improving their compliance testing process. This saved both time and costs—allowing their developers to focus on adding valuable features rather than merely ensuring legal standards were met.
A Final Look
In summary, parallel testing is more than just a trend; it is a strategy that can yield significant time and cost savings. The ability to run multiple tests simultaneously offers faster feedback, promotes better resource utilization, and, ultimately, enhances software quality.
As the demand for rapid development increases, adopting parallel testing methodologies could set companies apart, making them agile and responsive.
For more information on effective testing strategies, check out Agile Testing: A Practical Guide.
Embrace the power of parallel testing and transform your development process today!