Boost Code Quality: Eliminate Bugs with High Cohesion
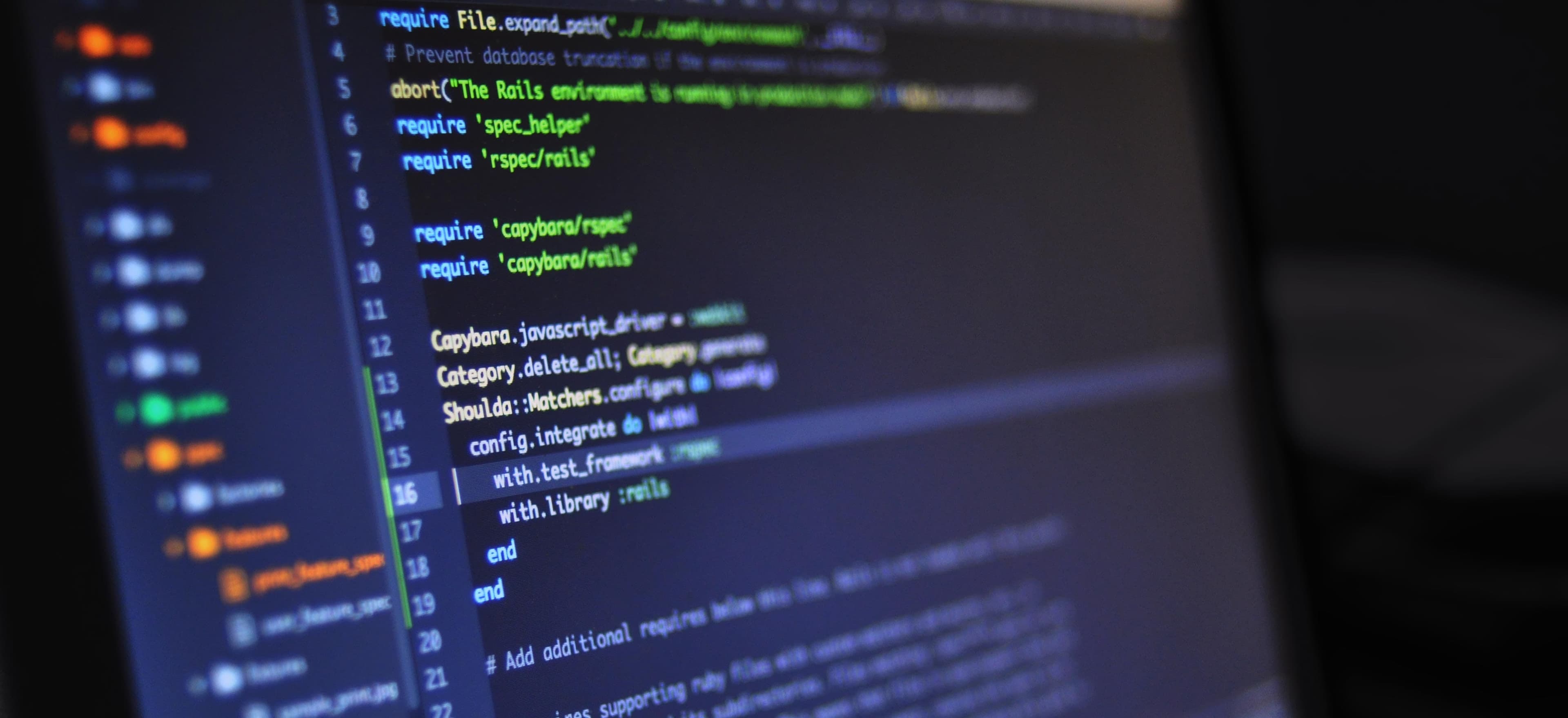
- Published on
Boost Code Quality: Eliminate Bugs with High Cohesion
In the ever-evolving landscape of software development, ensuring high code quality has become a fundamental objective. One of the core principles to achieving this is high cohesion. In this blog post, we will explore what high cohesion means, its benefits, and how you can implement it in your Java code to eliminate bugs effectively.
What is High Cohesion?
Cohesion refers to how closely related and focused the responsibilities of a single module, class, or function are. In simpler terms, a module with high cohesion performs one task exceptionally well and does so reliably. High cohesion leads to code that is easier to maintain and less prone to bugs, as the functionality is encapsulated within a self-contained unit.
Types of Cohesion
Cohesion can be categorized into several types:
- Functional Cohesion: Every part of the module is necessary for a single, well-defined task.
- Sequential Cohesion: Output from one part of the module serves as input for another.
- Communicational Cohesion: Modules operate on the same data but may perform different tasks.
- Temporal Cohesion: Elements are grouped based on a time they are executed.
- Procedural Cohesion: Tasks must be performed in a specific sequence.
- Logical Cohesion: Elements are grouped by similar characteristics, even if they are in no way related.
For the best code quality, aim for functional cohesion, where modules perform a single task.
Benefits of High Cohesion
High cohesion provides several advantages that can significantly enhance code quality:
- Easier Maintenance: When modules focus on a single task, changes or bug fixes can be made within that module, reducing the risk of unintentionally affecting other functionalities.
- Improved Readability: Code that is cohesive is often more understandable. Developers can quickly grasp what a module is meant to do.
- Simplified Testing: When a module is tightly focused, it becomes easier to test to ensure it behaves as expected, resulting in fewer bugs.
Achieving High Cohesion in Java
Implementing high cohesion in Java involves strategic design decisions and best coding practices. Below are several approaches you can take to enhance cohesion in your Java projects.
1. Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change. This principle promotes high cohesion by ensuring that a class encapsulates a single functionality.
Example Code Snippet
// Before applying SRP
public class UserProfile {
private String username;
private String email;
public void saveProfile() {
// logic to save profile
}
public void sendEmail() {
// logic to send email
}
}
// After applying SRP
public class UserProfile {
private String username;
private String email;
public void saveProfile() {
// logic to save profile
}
}
public class EmailService {
public void sendEmail(String recipient, String message) {
// logic to send email
}
}
Commentary: In the first example, the UserProfile
class mixes profile management with email functionality. After applying SRP, we separate the email functionality into an EmailService
class, leading to high cohesion and easier maintenance.
2. Avoid Long Parameter Lists
Long parameter lists can make code difficult to read and maintain. Instead of passing numerous parameters to a function, encapsulate them in a class or structure.
Example Code Snippet
// Before: Long parameter list
public void createOrder(String productName, int quantity, String userAddress) {
// logic to create an order
}
// After: Encapsulating parameters
public class OrderRequest {
private String productName;
private int quantity;
private String userAddress;
// Constructor, Getters, and Setters
}
public void createOrder(OrderRequest request) {
// logic to create an order
}
Commentary: Instead of passing individual parameters, we create an OrderRequest
class. This enhances readability and focuses the createOrder
method on its primary responsibility.
3. Utilize Interfaces for Clear Contracts
Using interfaces can lead to better cohesion by defining clear contracts that classes need to fulfill. This encourages modules to do one thing and do it well.
Example Code Snippet
public interface PaymentProcessor {
void processPayment(double amount);
}
public class CreditCardProcessor implements PaymentProcessor {
@Override
public void processPayment(double amount) {
// logic to process credit card payment
}
}
public class PayPalProcessor implements PaymentProcessor {
@Override
public void processPayment(double amount) {
// logic to process PayPal payment
}
}
Commentary: Here, we define a PaymentProcessor
interface with a single method. Each payment processor class implements its payment logic, adhering to a single responsibility. This makes it easier to add new payment methods without modifying existing code.
4. Favor Composition over Inheritance
While inheritance can lead to code reuse, it can also introduce unnecessary coupling, which can affect cohesion. Favor composition, where classes are composed of other classes, to enhance functionality while maintaining high cohesion.
Example Code Snippet
public class Order {
private PaymentProcessor paymentProcessor;
public Order(PaymentProcessor paymentProcessor) {
this.paymentProcessor = paymentProcessor;
}
public void completeOrder(double amount) {
paymentProcessor.processPayment(amount);
// logic for completing the order
}
}
// Usage
PaymentProcessor payment = new CreditCardProcessor();
Order order = new Order(payment);
order.completeOrder(100.00);
Commentary: By using composition, the Order
class does not inherit from PaymentProcessor
but utilizes it. This keeps each class focused on its specific role, promoting high cohesion.
To Wrap Things Up
High cohesion is a crucial principle in software engineering that significantly enhances the maintainability, readability, and testability of code. By adhering to principles like the Single Responsibility Principle, avoiding long parameter lists, utilizing interfaces, and favoring composition, you can promote high cohesion in your Java applications.
Additional Resources
- Design Principles - Object-Oriented Programming
- Effective Java by Joshua Bloch
- Refactoring Guru - SOLID Principles
By implementing these strategies, you not only improve code quality but also foster a culture of excellence within your team. Start applying high cohesion in your projects today and watch your bug count drop significantly!