Finding Balance: Simplifying Lock File Management
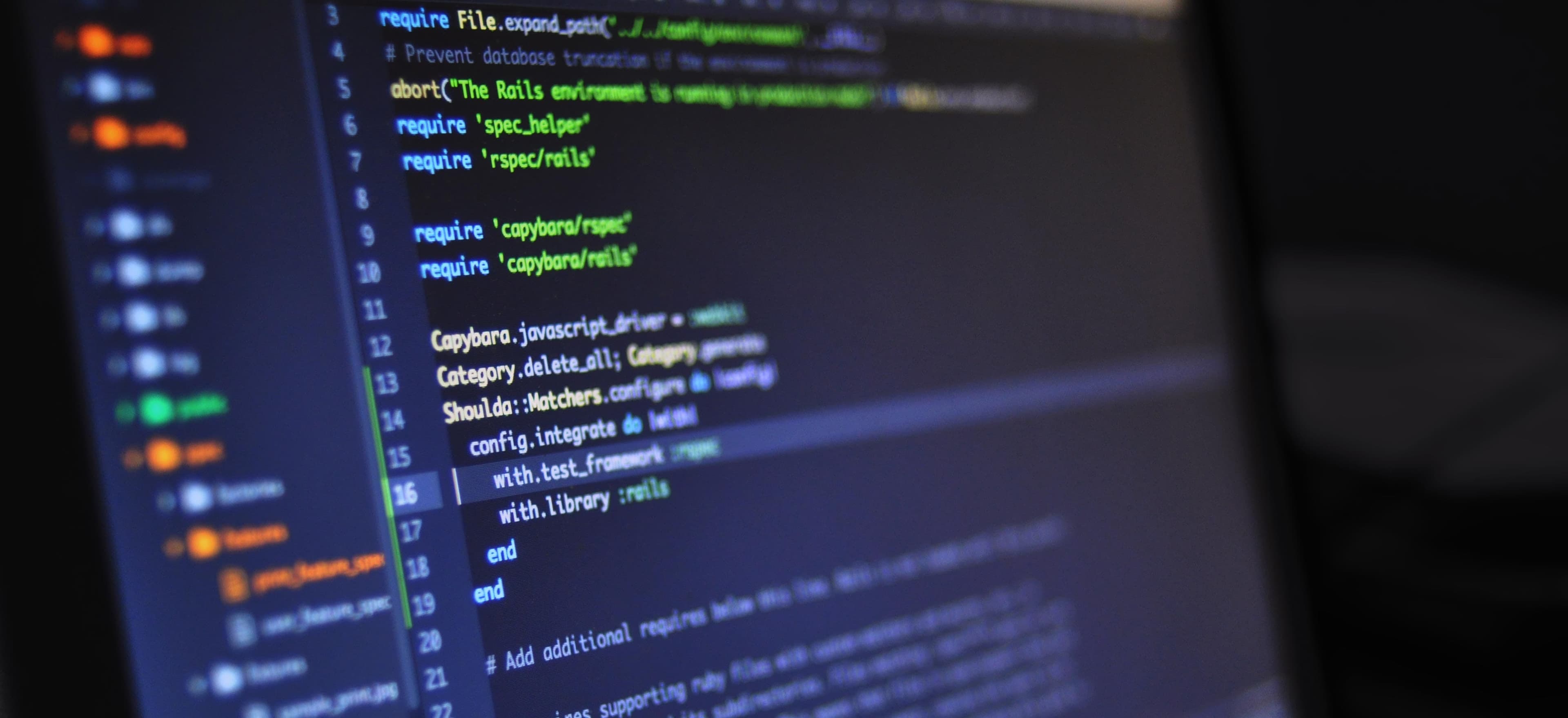
- Published on
Finding Balance: Simplifying Lock File Management in Java
In the world of software development, managing dependencies is one of the crucial tasks that developers encounter. Among the complexities of managing dependencies, lock files play a vital role in ensuring the reliability and stability of applications. In this blog post, we will explore what lock files are, why they are essential in Java dependency management, and how to simplify their management.
What are Lock Files?
Lock files, generally known as dependency lock files, help to lock the versions of the libraries and packages that a project depends on. When you run a build tool (like Maven or Gradle), the lock file ensures that the same version of a dependency is used every time the build is executed. This guarantees consistent builds across different environments.
To better understand this, let's take a look at a simplified version of what a lock file might look like in a Java project using Maven:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.example</groupId>
<artifactId>my-library</artifactId>
<version>1.0.0</version>
</dependency>
<dependency>
<groupId>org.another</groupId>
<artifactId>another-library</artifactId>
<version>2.3.1</version>
</dependency>
</dependencies>
</dependencyManagement>
Here, this lock file specifies the exact versions of the dependencies that should be used. Having fixed versions not only helps in maintaining the stability of the application but also prevents scenarios where inconsistencies lead to bugs during runtime.
Why are Lock Files Important?
Lock files serve several key purposes:
-
Version Control: They help maintain control over the versions of dependencies, ensuring that the same version is used in development, testing, and production environments.
-
Faster Installation: Lock files make dependency resolution faster because the build tool does not need to evaluate the version constraints again, it knows exactly which versions to download.
-
Security: By locking the versions of libraries, developers can avoid automatically picking up updates that may introduce vulnerabilities or breaking changes.
-
Collaboration: In team projects, lock files ensure that all team members are using the same set of dependencies, thus avoiding the "It works on my machine" syndrome.
Simplifying Lock File Management
While the importance of lock files is clear, managing them can lead to complexities. Here are some best practices and tools that can simplify lock file management in Java:
1. Use Dependency Management Tools
Java's ecosystem provides several tools that simplify managing lock files. Two of the most popular build tools, Maven and Gradle, have built-in mechanisms for managing dependencies and their versions.
Using Maven
Maven's pom.xml
is a powerful tool for managing dependencies. Below is an example of how you might define dependencies:
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
In this instance, we are adding JUnit as a test dependency. After this, running the mvn install
command will create a lock file that reflects these versions.
Using Gradle
Gradle offers a more flexible approach—here's a sample configuration for managing dependencies:
dependencies {
testImplementation 'junit:junit:4.12'
}
Running gradle build
will resolve the dependencies and generate a lock file (gradle.lockfile
) that will contain all relevant version information.
2. Utilize the dependency:tree
Command
In Maven, you can use the dependency:tree
command to visualize the dependency graph of your project. This command helps in understanding the project's dependencies, their versions, and where conflicts exist.
mvn dependency:tree
By analyzing this tree, you can make informed decisions about version conflicts and which updates to apply.
3. Leverage Version Ranges Sparingly
Although it is tempting to use version ranges (like [1.0,2.0)
), it’s advisable to do so sparingly. Version ranges introduce uncertainty in your builds. A lock file should ideally specify exact versions to enhance repeatability.
4. Regularly Update Dependencies
Keeping your dependencies up to date is critical, but so is managing this process efficiently. Tools like Dependabot monitor your repositories and automatically create pull requests for outdated dependencies. Implementing such tools can streamline the update process, allowing you to focus on other tasks.
5. Use Strong Hashing for Integrity
Another practice is to utilize strong checksums to guarantee the integrity of the dependencies you are pulling in. Furthermore, validating checksums as part of your build process ensures that the libraries you depend on are what you expect and haven’t been tampered with.
<dependency>
<groupId>org.example</groupId>
<artifactId>my-library</artifactId>
<version>1.0.0</version>
<scope>compile</scope>
</dependency>
<dependencies>
<!-- Add checksum validation here -->
</dependencies>
This approach not only secures the build but also enhances trust in the dependencies being used.
6. Document and Enforce Policies
It is also a good idea to document and enforce policies dictating how dependencies should be added and maintained in your codebase. Regular code reviews should scrutinize any changes to lock files to ensure compliance with best practices.
Closing the Chapter
Lock file management doesn’t have to be an overwhelming task in Java development. By leveraging the tools and practices we discussed, you can simplify the dependency locking process, enhance the stability of your applications, and foster collaboration among team members.
Remember, lock files are your friends; they provide a safeguard against the chaos of dependency resolution. As you continue your development journey, let these practices guide you toward creating stable and reliable applications.
For more information on dependency management in Java, check out the respective documentation for Maven and Gradle.
By mastering lock file management, you set a strong foundation for your project's success. Happy coding!