Mastering VBox and HBox: Common Layout Issues in JavaFX 20
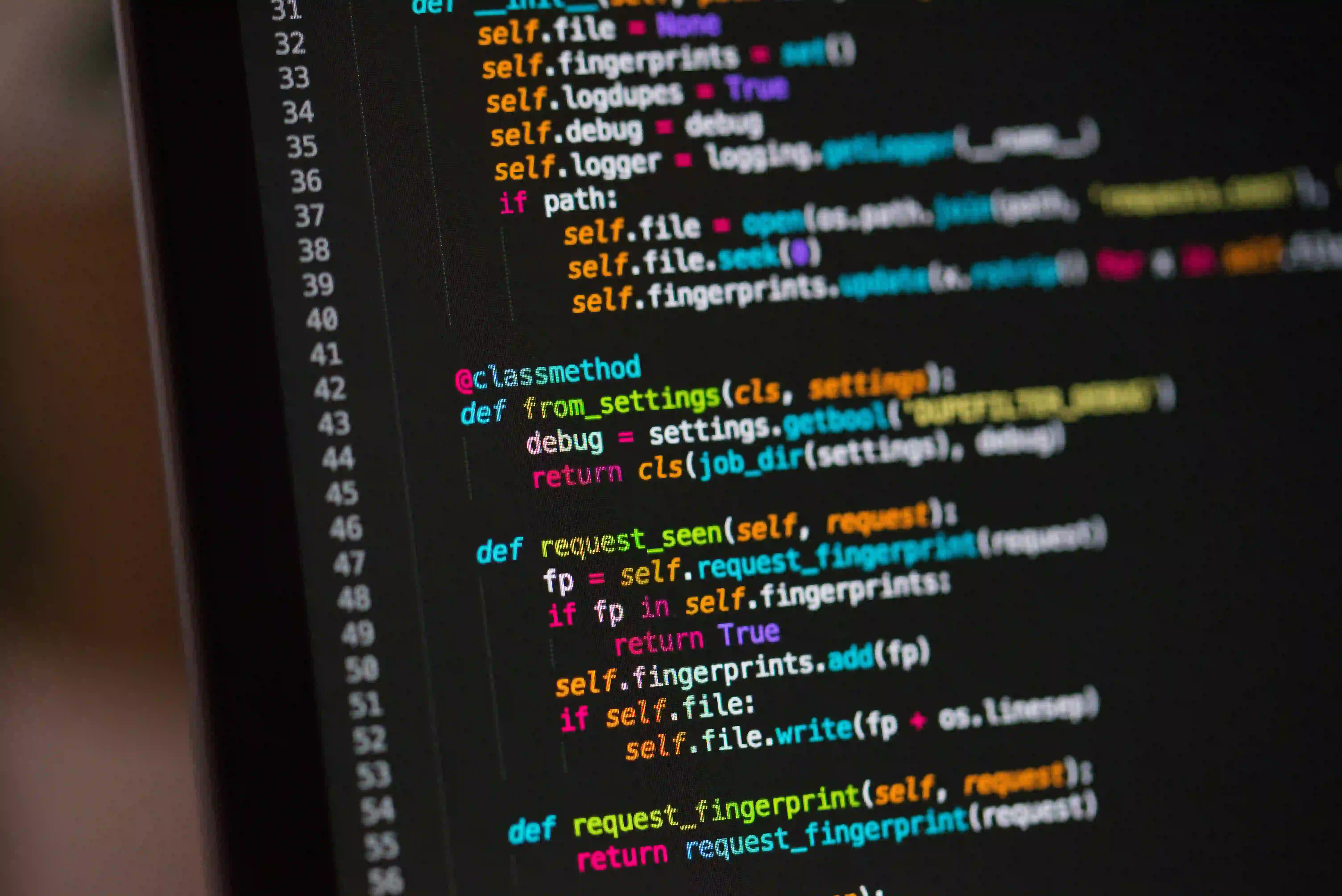
Mastering VBox and HBox: Common Layout Issues in JavaFX 20
JavaFX is a powerful toolkit for building rich client applications in Java. Among the many features it offers, layout management is crucial for designing responsive user interfaces. In this blog post, we will dive into two essential layout classes, VBox
and HBox
, common issues developers face, and best practices to master them in JavaFX 20.
Understanding VBox and HBox
What is VBox?
VBox
is a layout manager that arranges its children vertically in a single column. It allows for various layout configurations, such as specifying spacing between elements, alignment, and fill behavior.
What is HBox?
On the other hand, HBox
arranges its children horizontally in a single row. Similar to VBox
, it provides properties to control spacing, alignment, and other layout behaviors.
Here’s a simple example to illustrate both VBox
and HBox
:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class LayoutExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button1 = new Button("Button 1");
Button button2 = new Button("Button 2");
Button button3 = new Button("Button 3");
// HBox instance for horizontal layout
HBox hBox = new HBox(10); // 10 pixels of spacing
hBox.getChildren().addAll(button1, button2, button3);
Button button4 = new Button("Button 4");
Button button5 = new Button("Button 5");
// VBox instance for vertical layout
VBox vBox = new VBox(10); // 10 pixels of spacing
vBox.getChildren().addAll(hBox, button4, button5);
Scene scene = new Scene(vBox, 300, 250);
primaryStage.setTitle("VBox and HBox Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Commentary on the Code
In the provided code, we create a simple JavaFX application that utilizes both VBox
and HBox
.
HBox
holds three buttons arranged horizontally, demonstrating how components line up in one direction.- The
VBox
element then contains theHBox
alongside two additional buttons, showcasing vertical alignment.
Common Layout Issues and Solutions
While VBox
and HBox
are straightforward, they can lead to unexpected results if not handled properly. Below, we discuss a few common issues.
1. Overflow and Clipping
Sometimes, child elements may not fit in the designated layout area, leading to overflow. This typically happens when fixed sizes are defined for HBox
or VBox
, which exceeds the window size.
Solution: Use minWidth
, maxWidth
, minHeight
, and maxHeight
properties wisely. It can also help to leverage setPrefSize()
instead of fixed sizes.
Example modification:
hBox.setMinWidth(200);
hBox.setMaxWidth(300);
2. Misalignment
Misalignment in components can arise if alignment properties are not properly set. For instance, buttons in an HBox
might get pushed to the left, making the layout look off.
Solution: Utilize setAlignment()
to define how the children should be aligned.
hBox.setAlignment(Pos.CENTER); // Aligns buttons to the center
3. Child Component Sizing
Each child component's preferred size can lead to inconsistent layouts. For example, a button may have a different preferred size based on its label, pushing other elements out of line.
Solution: Use setPrefWidth()
and setPrefHeight()
on HBox
and VBox
children to standardize sizes.
button1.setPrefWidth(100);
4. Unwanted Gaps
Gaps may appear due to unnecessary margins or padding defined on child elements, resulting in visual clutter.
Solution: Adjust the setMargin()
and setPadding()
properties.
Example of setting padding:
VBox.setMargin(button4, new Insets(10));
5. Responsiveness
In modern UI design, responsiveness is vital. Sometimes, components do not resize properly when the window is scaled.
Solution: Utilize layout properties carefully and avoid hardcoding sizes. Always consider using percentage-based sizes or bind properties.
hBox.prefWidthProperty().bind(scene.widthProperty().multiply(0.8)); // Binds width to 80% of the scene
Additional Layout Tools
JavaFX also provides other layout managers like GridPane
, BorderPane
, and more for complex interfaces. Each layout serves a purpose; understanding their behavior alongside VBox
and HBox
will enhance your design capabilities.
For those interested, JavaFX Layout Documentation is a valuable resource to familiarize yourself with these tools.
The Last Word
Mastering VBox
and HBox
requires an understanding of their properties, common issues, and how to leverage JavaFX’s flexibility for responsive design. By addressing the common pitfalls outlined above and employing best practices, you can create sleek, visually appealing interfaces.
Now it's your turn! Experiment with VBox
and HBox
in your JavaFX projects and see how these layouts can improve your application design. Happy coding!
Additional Resources
By grasping these concepts, you can significantly enhance the user experience in your JavaFX applications. Each layout manager has its strengths; choose wisely based on your application’s needs.