Overcoming Common Challenges in Apache Isis Development
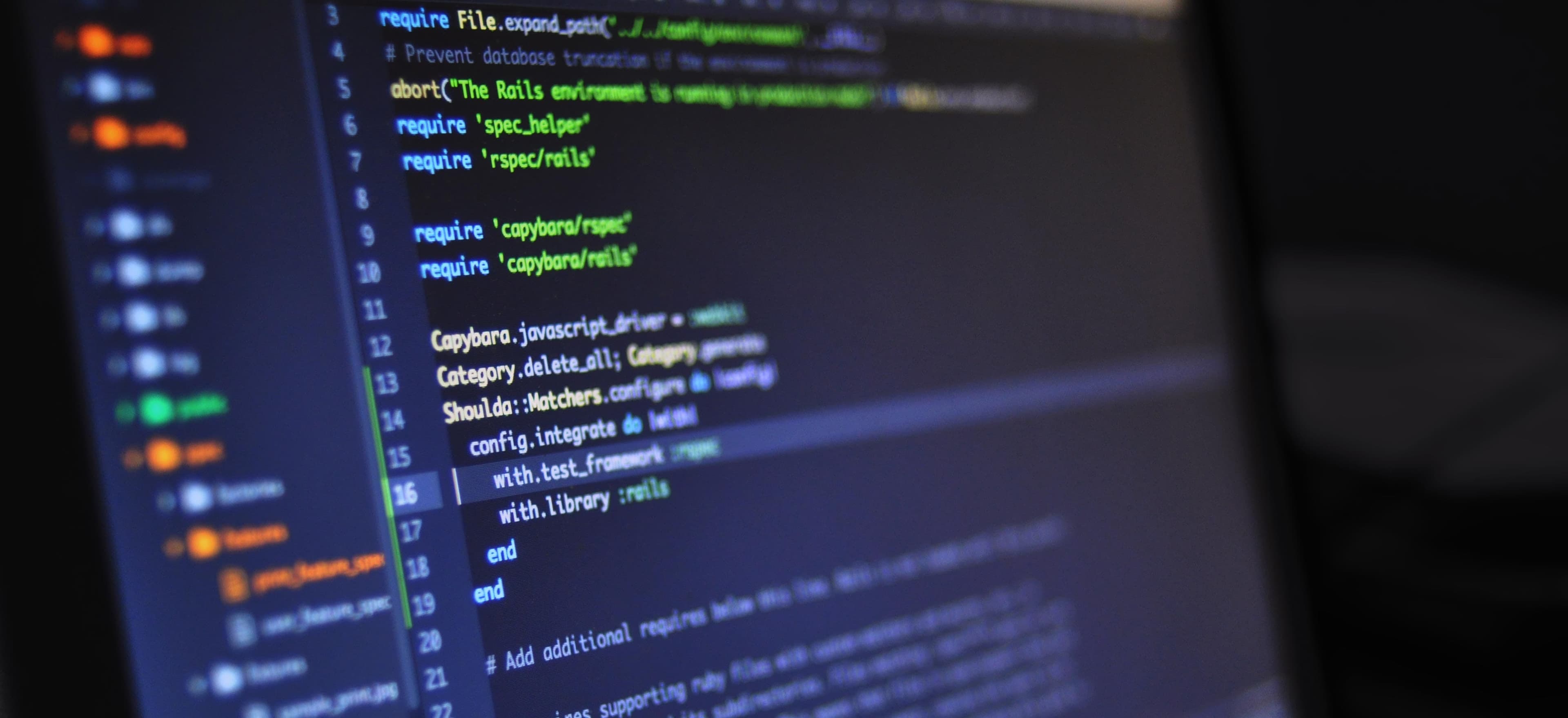
- Published on
Overcoming Common Challenges in Apache Isis Development
Apache Isis is a powerful framework that facilitates the development of domain-driven applications with an emphasis on simplicity and maintainability. Despite its strengths, developers often encounter various challenges while using Apache Isis. In this blog post, we will explore these common challenges and offer practical strategies for overcoming them.
Table of Contents
- Understanding Apache Isis
- Common Challenges in Apache Isis Development
- Complexity in Domain Model Design
- User Interface Customization
- Testing Difficulties
- Handling Business Logic
- Overcoming the Challenges
- Best Practices for Apache Isis Development
- Conclusion
Understanding Apache Isis
Apache Isis is a framework for building business applications in Java. It offers rich domain modeling capabilities and abstracts much of the complexity of web application development. With its help, you can rapidly create applications by focusing on your domain model rather than the underlying infrastructure.
For a detailed introduction to Apache Isis, visit the official documentation.
Common Challenges in Apache Isis Development
Complexity in Domain Model Design
When working with Apache Isis, developers often struggle with designing an effective domain model. The domain model is foundational to your application, and complexity can scale quickly as more features are added.
Why Is This a Problem?
A complex domain model can lead to difficulties in maintenance, increased chances of bugs, and a steeper learning curve for new developers joining the project.
User Interface Customization
While Apache Isis provides built-in UI components, there may be times when you require a more customized user experience. The default UI might not fit specific business needs, leading to dissatisfaction among end-users.
Why Is This a Problem?
If the UI is neither user-friendly nor tailored to the users’ needs, it can hinder the adoption of your application and affect overall productivity.
Testing Difficulties
Testing an application built with Apache Isis can present challenges due to the framework's unique architecture. Developers may find it hard to isolate components for unit testing or struggling with mocking components.
Why Is This a Problem?
Insufficient testing can lead to undetected bugs, increased technical debt, and, ultimately, a less reliable application.
Handling Business Logic
Separating business logic from the domain model is essential for clean architecture. However, Apache Isis encourages embedding business logic directly within domain entities.
Why Is This a Problem?
Placing too much business logic in the domain model increases complexity and slows down development when updates or changes are required.
Overcoming the Challenges
Simplifying Domain Model Design
-
Start Small: Begin with a minimal domain model and gradually expand as requirements become clearer. This iterative approach allows you to accommodate change without getting overwhelmed.
-
Refactor Regularly: Make a habit of reviewing and refactoring your domain model. This ensures that it stays relevant to your evolving application.
-
Utilize Value Objects: Use value objects for representing measurable attributes and avoid complex entities where unnecessary.
@Value
public class Money {
private final BigDecimal amount;
private final Currency currency;
// Constructors, getters, and other domain methods
}
Here, Money is a value object which simplifies the model by focusing only on the amount and currency, thus adding clarity.
Enhancing User Interface Customization
-
Leverage UI Customization Options: Apache Isis allows developers to create 'decorators' to customize how entities are displayed and manipulated.
-
Consider Third-Party UI Libraries: If the built-in features fall short, consider integrating libraries like Bootstrap or custom JavaScript for enhanced interactivity.
@Action
public Product createProduct(
@Parameter(description = "Product Name") String name,
@Parameter(description = "Product Price") Money price) {
// Product Creation Logic
}
Custom parameter descriptions can improve UI clarity and user understanding.
Improving Testing Practices
-
Employ Test Fragments: Utilize test fragments to simplify isolating components. This modularity promotes better test management.
-
Use Mocking Frameworks Wisely: Frameworks like Mockito assist in mocking dependencies effectively.
@RunWith(MockitoJUnitRunner.class)
public class ProductTest {
@Mock
ProductRepository repository;
@InjectMocks
ProductService service;
@Test
public void testProductCreation() {
// Mocking behavior and testing
}
}
Here, Mockito is used to test a service without relying on actual database calls.
Separating Business Logic
- Utilize Domain Services: Create services that encapsulate business logic. This helps reduce the burden on your domain entities.
@DomainService
public class PriceCalculator {
public Money calculateDiscountedPrice(Product product, BigDecimal discount) {
// Business logic for calculating discounted price
}
}
In this example, business logic is separated from the entity, allowing for a cleaner design and making it easier to maintain.
Best Practices for Apache Isis Development
-
Documentation is Key: Maintain comprehensive documentation to aid new developers and ensure consistency within the team.
-
Version Control: Use Git or another version control system to manage changes effectively.
-
Code Reviews: Regular code reviews foster collaboration and catch potential pitfalls early.
-
Stay Updated: Follow the development community around Apache Isis. Engaging with others can provide insight into best practices and the latest updates.
The Bottom Line
While Apache Isis presents several challenges during development, understanding and strategically addressing these issues can lead to better outcomes. By focusing on the design of the domain model, customizing the user interface, enhancing testing practices, and separating business logic, developers can create robust and maintainable applications.
The key lies in iterative improvement, collaboration, and engagement with the community and resources available. For further exploration of Apache Isis, check out the community resources that offer insightful discussions and shared experiences among developers.
Ultimately, embracing a proactive mindset will empower you to effectively overcome these challenges and leverage the full potential of Apache Isis in your development endeavors. Happy coding!
Checkout our other articles