Mastering Immutable Maps in Java: Common Pitfalls
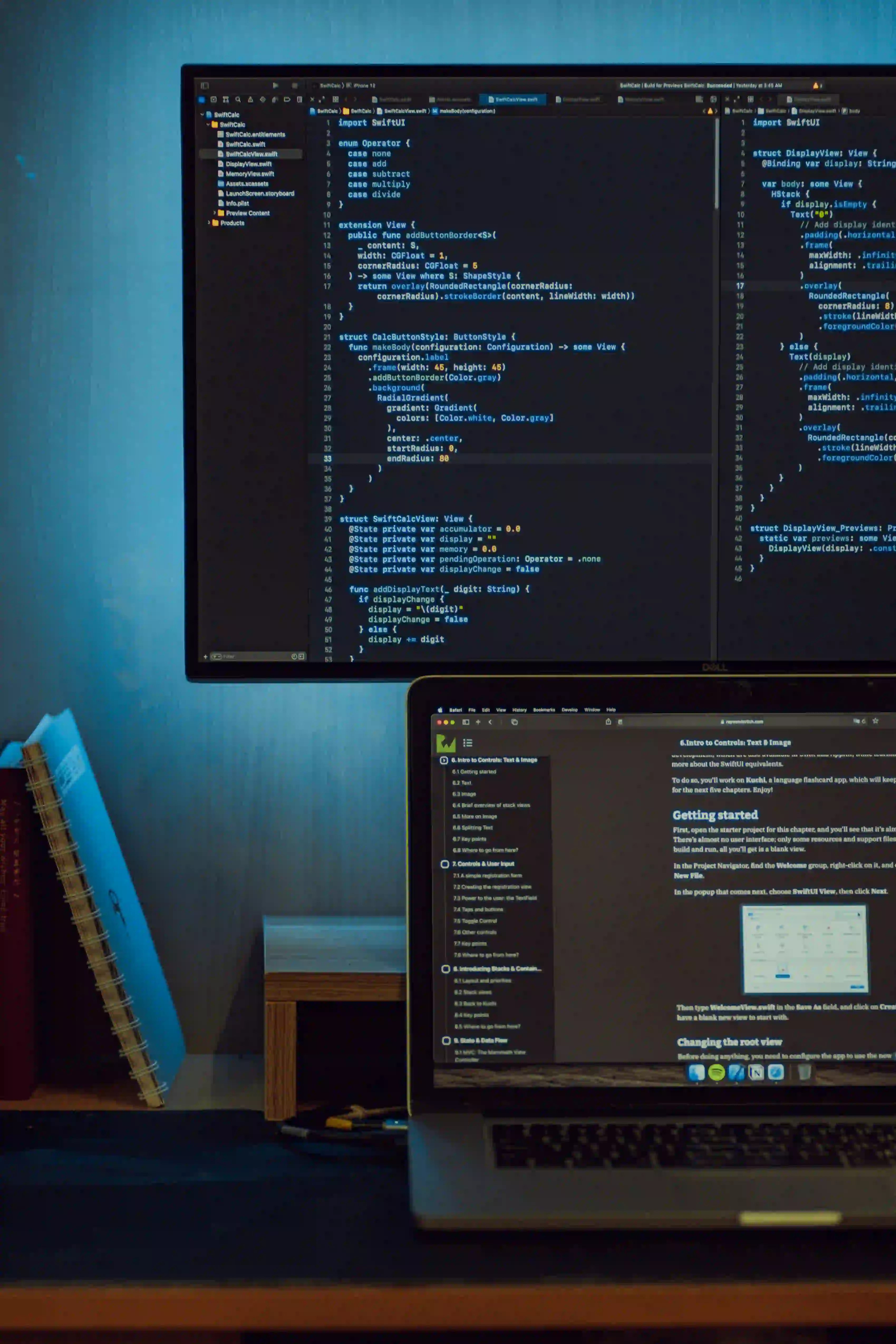
Mastering Immutable Maps in Java: Common Pitfalls
Java developers frequently encounter the need to use maps for data storage. Among the varied map implementations, immutable maps have grown in popularity due to their thread-safety, ease of use, and significant performance benefits. However, even seasoned Java developers can fall into certain traps when working with immutable maps. In this blog post, we will explore immutable maps in Java, discuss common pitfalls, and provide valuable insights into maximizing their use.
What is an Immutable Map?
An immutable map is a data structure that, once created, cannot be modified. Any operation that tries to change the map, such as adding or removing elements, results in the creation of a new map instead. This can lead to clearer and safer code, especially in multi-threaded applications where shared state can lead to issues.
In Java, the most common immutable map implementation is offered by the java.util.Collections
class and through the Map.of()
method that was introduced in Java 9.
Here’s an example of creating an immutable map using Map.of()
:
import java.util.Map;
public class ImmutableMapExample {
public static void main(String[] args) {
Map<String, Integer> immutableMap = Map.of("Apple", 1, "Banana", 2);
System.out.println(immutableMap);
}
}
Why Use Immutable Maps?
- Thread Safety: Since the state does not change, immutable maps are inherently thread-safe.
- Simplicity: Reduces complexity when dealing with concurrent modifications.
- Performance: Immutable objects can be optimized by the JVM, enhancing performance.
Common Pitfalls When Using Immutable Maps
With an understanding of immutable maps laid out, let’s delve into some common pitfalls developers might encounter.
1. Overusing Immutable Maps When Mutability is Needed
One of the most significant mistakes is overreliance on immutable maps in contexts where mutability is not only acceptable but needed. If your application requires frequent updates to the data structure or needs to perform operations like sorting or filtering, immutable maps can introduce unnecessary overhead.
Consider a simple example of modifying a map:
import java.util.HashMap;
import java.util.Map;
public class MutableMapExample {
public static void main(String[] args) {
Map<String, Integer> mutableMap = new HashMap<>();
mutableMap.put("Apple", 1);
mutableMap.put("Banana", 2);
// Mutate the map
mutableMap.put("Orange", 3);
System.out.println(mutableMap);
}
}
In this case, a mutable map (like HashMap
) is the right choice because frequent updates are performed.
2. Ignoring Performance Considerations with Large Data
When large immutable maps are created, the act of creating new versions of maps can lead to performance degradation. Each modification requiring a new instance can lead to increased memory consumption and garbage collection overhead.
A good practice is to concatenate data pre-emptively to design cycles, minimizing the number of immutable map creations.
For example:
import java.util.Map;
import java.util.HashMap;
public class LargeImmutableMapExample {
public static void main(String[] args) {
Map<String, Integer> largeMap = new HashMap<>();
// Populate the mutable map...
for (int i = 0; i < 1000; i++) {
largeMap.put("Key" + i, i);
}
// Transform to immutable
Map<String, Integer> immutableMap = Map.copyOf(largeMap);
System.out.println(immutableMap);
}
}
3. Not Understanding Null Handling
Immutable maps in Java do not allow null key/value pairs. Attempting to include a null key or value will throw a NullPointerException
. This can lead to runtime failures if you are not cautious.
Example:
try {
Map<String, Integer> mapWithNull = Map.of("Key", null);
} catch (NullPointerException e) {
System.err.println("Cannot use null as a value!");
}
To handle potential nulls, ensure to validate your data before creating the map, or utilize methods that can effectively handle null values.
4. Lack of Awareness for Copying Mechanisms
Creating copies of immutable maps comes with its own set of corners. For instance, using Map.copyOf()
is an easy way to generate a new immutable map based on an existing one, but it requires the source map to be mutable.
5. Ignoring Java's Functional Programming Inputs
Java's Stream API and functional interfaces can be a boon when working with immutable maps. However, many developers do not fully leverage these techniques.
For instance, consider the following code that creates an immutable map with transformed keys:
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class StreamImmutableMapExample {
public static void main(String[] args) {
Map<String, Integer> transformedMap = Stream.of("Apple", "Banana", "Orange")
.collect(Collectors.toMap(
String::toUpperCase,
String::length
));
System.out.println(transformedMap);
}
}
Utilizing Java Streams can enhance performance, especially when handling larger datasets, while also making code more concise.
Closing the Chapter
Immutable maps bring significant benefits to Java applications, particularly in terms of thread safety and clarity. However, pitfalls abound, from situations requiring mutability to performance issues with larger datasets and null handling.
For developers looking to deepen their understanding of collections in Java, consider reading Java Collections Tutorial. This resource provides extensive knowledge that can further the ability to manage data effectively.
Ultimately, mastering immutable maps involves understanding their nuances and avoiding common traps. As you practice and experiment with your code, you will grow more comfortable and adept at leveraging the power of immutability in your Java programs. Happy coding!