Mastering Functional Style in Java: Common Pitfalls to Avoid
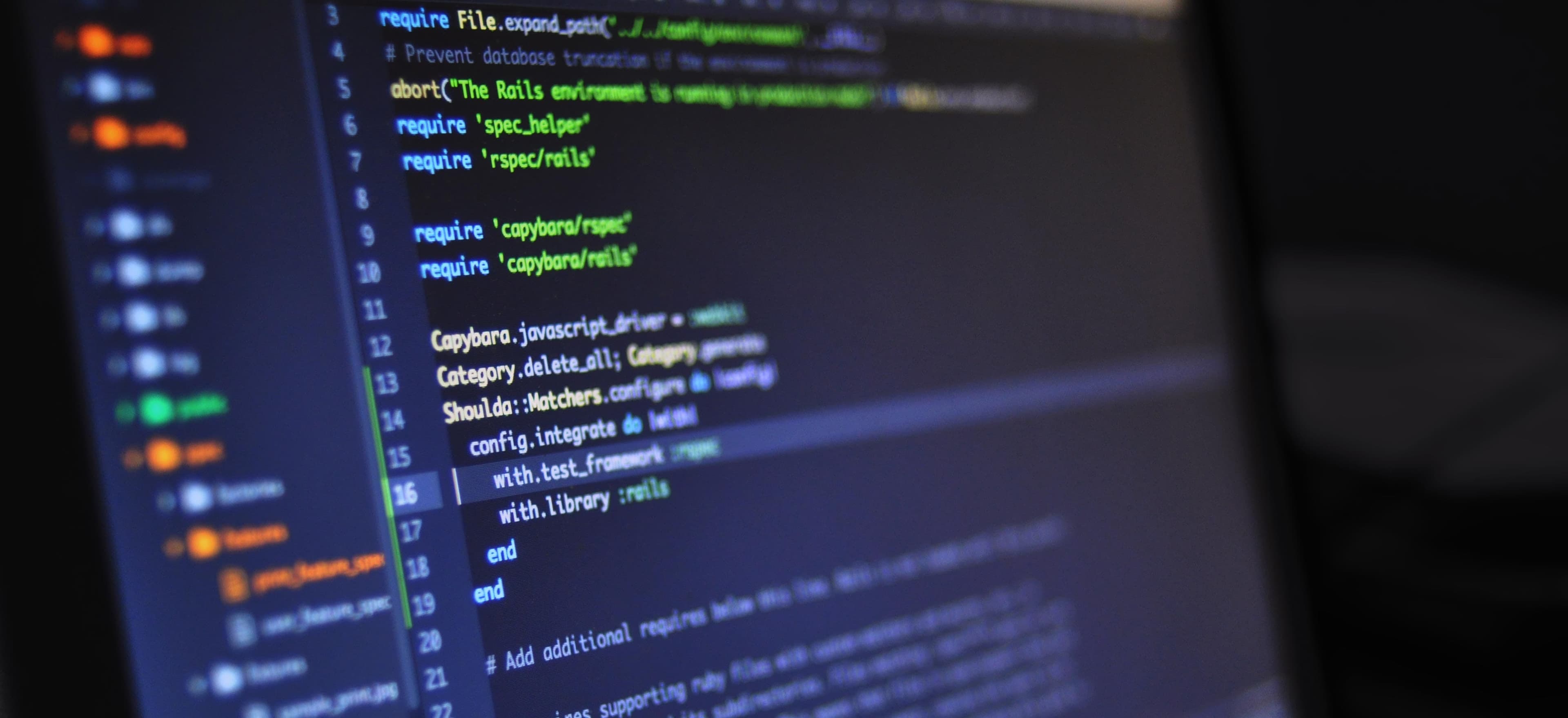
- Published on
Mastering Functional Style in Java: Common Pitfalls to Avoid
Java has made impressively large strides over the past few years, especially with the introduction of functional programming paradigms in Java 8. This evolution allows developers to write cleaner, more maintainable code. But as with any paradigm shift, transitioning to a functional style in Java can introduce its own set of pitfalls. This blog post will explore common mistakes developers make and offer guidelines on how to avoid them.
Understanding the Functional Paradigm in Java
Functional programming (FP) emphasizes the use of functions as first-class citizens. In this paradigm, functions can be assigned to variables, passed as arguments, or returned from other functions. FP often entails a shift in mindset compared to imperative programming, focusing on what to solve rather than how to solve it.
Key Features of Functional Programming in Java
- Anonymous Functions (Lambdas): Java introduced lambda expressions, making it easier to write concise and functional-style code.
- Higher-order Functions: These are functions that can take other functions as parameters or return them as results.
- Streams API: This lets you process collections of objects in a functional manner.
Here’s a simple example of a lambda expression:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name));
In this snippet, the forEach
method applies the lambda to each element in the names
list, printing them. The beauty of this is in its simplicity and clarity.
Common Pitfalls in Functional Programming with Java
Even with the advantages, you can easily fall into traps. Let's dive into some common pitfalls and how you can sidestep them.
1. Overusing Lambdas
Pitfall: While lambdas can enhance code readability, overusing them—or using them inappropriately—can lead to confusing code. Functions should remain simple and focused.
Solution: Limit the use of lambdas to straightforward cases. If your function is too complex, it’s usually better to define it as a named method.
// Overly complex lambda
names.sort((name1, name2) -> {
if(name1.length() > name2.length()) return -1;
else if(name1.length() < name2.length()) return 1;
return 0;
});
// Recommended named function
Comparator<String> compareByLength = (name1, name2) -> {
return Integer.compare(name1.length(), name2.length());
};
names.sort(compareByLength);
2. Mutable State in Streams
Pitfall: A common mistake is modifying external variables from within a stream operation. This can lead to unpredictable behavior and bugs that are hard to trace.
Solution: Aim to use immutable data. When using streams, focus on transformations and avoid side effects.
// Wrong: Modifying external variable
List<Integer> numbers = Arrays.asList(1, 2, 3);
List<Integer> results = new ArrayList<>();
numbers.forEach(num -> {
if (num % 2 == 0) results.add(num);
});
// Right: Using filter to avoid side effects
List<Integer> results = numbers.stream()
.filter(num -> num % 2 == 0)
.collect(Collectors.toList());
3. Not Leveraging Optional Properly
Pitfall: Java provides Optional
to handle nullability gracefully, but over-complicating things with excessive conditionals can lead to a convoluted codebase.
Solution: Use Optional
for return types when a method may not have a result. Avoid using it for every variable.
// Ineffective approach
Optional<User> userOpt = findUserById(id);
if (userOpt.isPresent()) {
return userOpt.get().getName();
}
return "Unknown";
// Effective approach
return findUserById(id)
.map(User::getName)
.orElse("Unknown");
4. Ignoring Performance
Pitfall: Functional programming in Java can sometimes lead to performance drops, particularly if not used judiciously. Creating unnecessary objects or using operations that aren’t efficient can slow down applications.
Solution: Profile and benchmark your code. Always look for balancing readability and performance.
// Potentially inefficient use of flatMap leading to object creation
List<List<String>> listOfLists = Arrays.asList(
Arrays.asList("One", "Two"),
Arrays.asList("Three", "Four")
);
List<String> flatList = listOfLists.stream()
.flatMap(Collection::stream)
.collect(Collectors.toList());
// Consider using parallelStream if performance is a concern
List<String> flatListParallel = listOfLists.parallelStream()
.flatMap(Collection::stream)
.collect(Collectors.toList());
5. Not Embracing Functional Interfaces
Pitfall: Java offers various built-in functional interfaces like Function
, Consumer
, Supplier
, and Predicate
. Not utilizing these can hinder writing more generic code.
Solution: Use those interfaces to define more flexible and reusable code snippets.
Function<Integer, Integer> squareFunction = x -> x * x;
System.out.println(squareFunction.apply(4)); // Outputs 16
// Predicate example to filter values
Predicate<Integer> isEven = n -> n % 2 == 0;
System.out.println(isEven.test(2)); // Outputs true
Summary
Adopting a functional style in Java can vastly improve your code's clarity and expressiveness. However, falling into common pitfalls can negate these advantages. Remember to:
- Use lambdas judiciously.
- Avoid modifying external state from within streams.
- Leverage
Optional
appropriately. - Balance performance with readability.
- Utilize built-in functional interfaces for better flexibility.
By understanding these pitfalls and employing thoughtful strategies, you can master functional programming in Java.
For further reading on functional programming principles and Java, check out Oracle's Documentation on Java Functional Programming and consider exploring Practical Functional Programming for more comprehensive insights.
Happy coding!