Debugging Common Issues in Serverless Functions
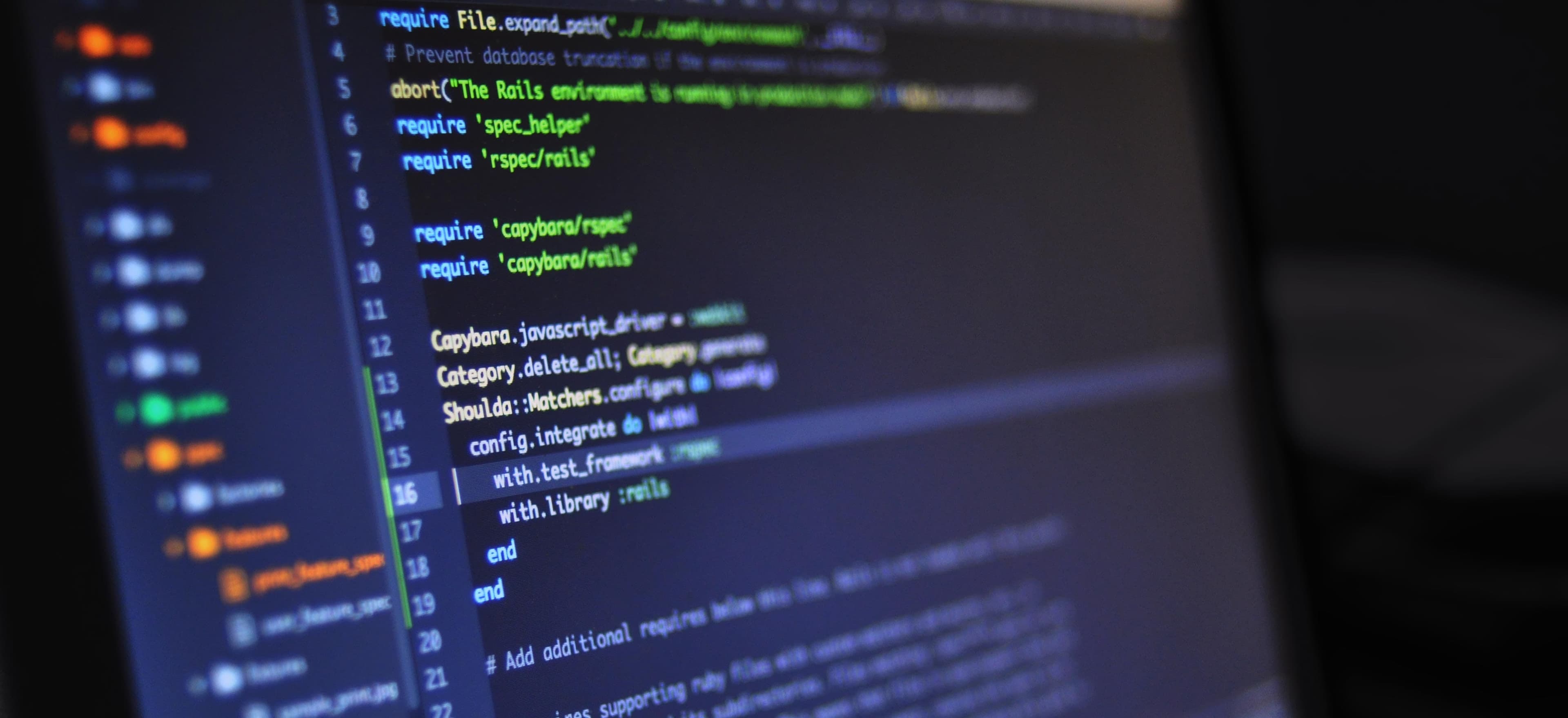
- Published on
Debugging Common Issues in Serverless Functions
Serverless computing has emerged as a viable solution for building scalable applications without the complexity of managing physical servers. Despite its advantages, serverless functions can often lead to perplexing debugging challenges. In this blog post, we’ll explore common issues encountered in serverless functions and provide actionable strategies to debug them effectively.
1. The Nature of Serverless Functions
Before diving into debugging, it's essential to understand what makes serverless functions unique. Serverless, as the name implies, abstracts the server management layer. This means:
- Event-driven Architecture: Serverless functions are typically triggered by events, which may be HTTP requests, timers, or database changes.
- Statelessness: Each execution is stateless, which means that you cannot rely on the function retaining information from one invocation to the next.
- Limited Execution Context: Serverless functions usually have time and memory limits.
These characteristics lead to unique challenges that necessitate a different approach to debugging.
2. Common Issues in Serverless Functions
Let's take a look at some of the issues that developers frequently encounter when working with serverless functions:
2.1 Cold Starts
Cold starts refer to the latency introduced when a serverless function is invoked after being idle for some time. This occurs because the cloud provider has to initialize the environment and load the function.
Solution:
To mitigate cold starts:
- Keep your functions warm by using a scheduled event to invoke them periodically.
- Optimize your code and configuration to reduce the cold start time.
Here’s an example of keeping a function warm in AWS Lambda using a CloudWatch Event rule:
{
"ScheduleExpression": "rate(5 minutes)",
"Targets": [
{
"Arn": "arn:aws:lambda:REGION:ACCOUNT_ID:function:FUNCTION_NAME",
"Id": "KeepWarm"
}
]
}
This rule triggers the function every 5 minutes, keeping it warm and reducing latency in user interactions.
2.2 Timeouts
Serverless functions often have strict timeout limits. Exceeding these limits can cause functions to exit prematurely, leading to incomplete or failed executions.
Solution:
Utilize logging and fine-tuning to handle timeouts effectively:
public class MyFunction implements RequestHandler<RequestType, ResponseType> {
public ResponseType handleRequest(RequestType request, Context context) {
context.getLogger().log("Received request: " + request);
// Your logic here
// Ensure that the operation is efficient
}
}
In this code, we're logging the request to track execution time. Monitor logs in your cloud provider's console to identify long-running operations.
2.3 Error Handling
Error handling in serverless environments can become convoluted due to the asynchronous nature of events. Unhandled exceptions can cause failures that don’t provide much context.
Solution:
Implement structured error handling:
try {
// Your code
} catch (SpecificException e) {
context.getLogger().log("Specific handler: " + e.getMessage());
// Return custom error response
} catch (Exception e) {
context.getLogger().log("Generic handler: " + e.getMessage());
// Return custom error response
}
This code snippet demonstrates how to catch specific exceptions first and handle them appropriately. By logging errors, you can better understand what went wrong during execution.
2.4 Dependency Management
Serverless functions often rely on external libraries or services. Incorrectly configured dependencies can lead to execution errors.
Solution:
Use dependency management tools specific to your language. For Java, Maven is prevalent. Ensure you include all dependencies:
<dependency>
<groupId>com.example</groupId>
<artifactId>mylibrary</artifactId>
<version>1.0.0</version>
</dependency>
If you encounter ClassNotFoundException
, check your packaging and deployment configurations to ensure that all dependencies are bundled correctly.
2.5 Configuration Errors
Configuration files often lead to confusion. Misconfigured environment variables or incorrect permissions can halt the function's execution.
Solution:
Double-check all configurations and use environment variables judiciously:
String dbUrl = System.getenv("DATABASE_URL");
if (dbUrl == null) {
throw new IllegalArgumentException("DATABASE_URL is not configured.");
}
Using environmental variables allows for easier configuration changes without altering the codebase. Always validate these variables during the function's initialization phase.
3. Debugging Strategies
Understanding common issues is only half the battle. Here are strategies to effectively debug serverless functions:
3.1 Use Logging Extensively
Cloud providers like AWS and Azure offer logging services. Use logging to capture important events, variable states, and error information.
context.getLogger().log("Starting execution");
3.2 Utilize Monitoring Tools
Tools like AWS CloudWatch and Azure Monitor can help track performance metrics and errors.
3.3 Local Testing
Use local development tools that simulate serverless environments. For instance, AWS SAM allows you to emulate Lambda functions locally.
Here is a simple command to run a local Lambda function:
sam local invoke MyFunction
3.4 Integration Tests
Incorporate comprehensive tests that check the integration of your serverless functions with other components. Testing against a staging environment mimics production behavior without risk.
4. Conclusion
Debugging serverless functions can indeed be tricky due to their stateless and ephemeral nature. Understanding common issues—ranging from cold starts to configuration errors—is pivotal in developing robust serverless applications. By adopting strategic debugging practices, you can streamline your development process and deliver high-quality, reliable cloud functions.
For further reading and enhancements in your serverless architecture, consider exploring the following resources:
- AWS Lambda Best Practices
- Azure Functions Documentation
Embrace the power of serverless computing, and with effective debugging strategies, your serverless functions will run smoothly and efficiently!
Checkout our other articles