Handling Suppressed Exceptions in Java 7: A Guide
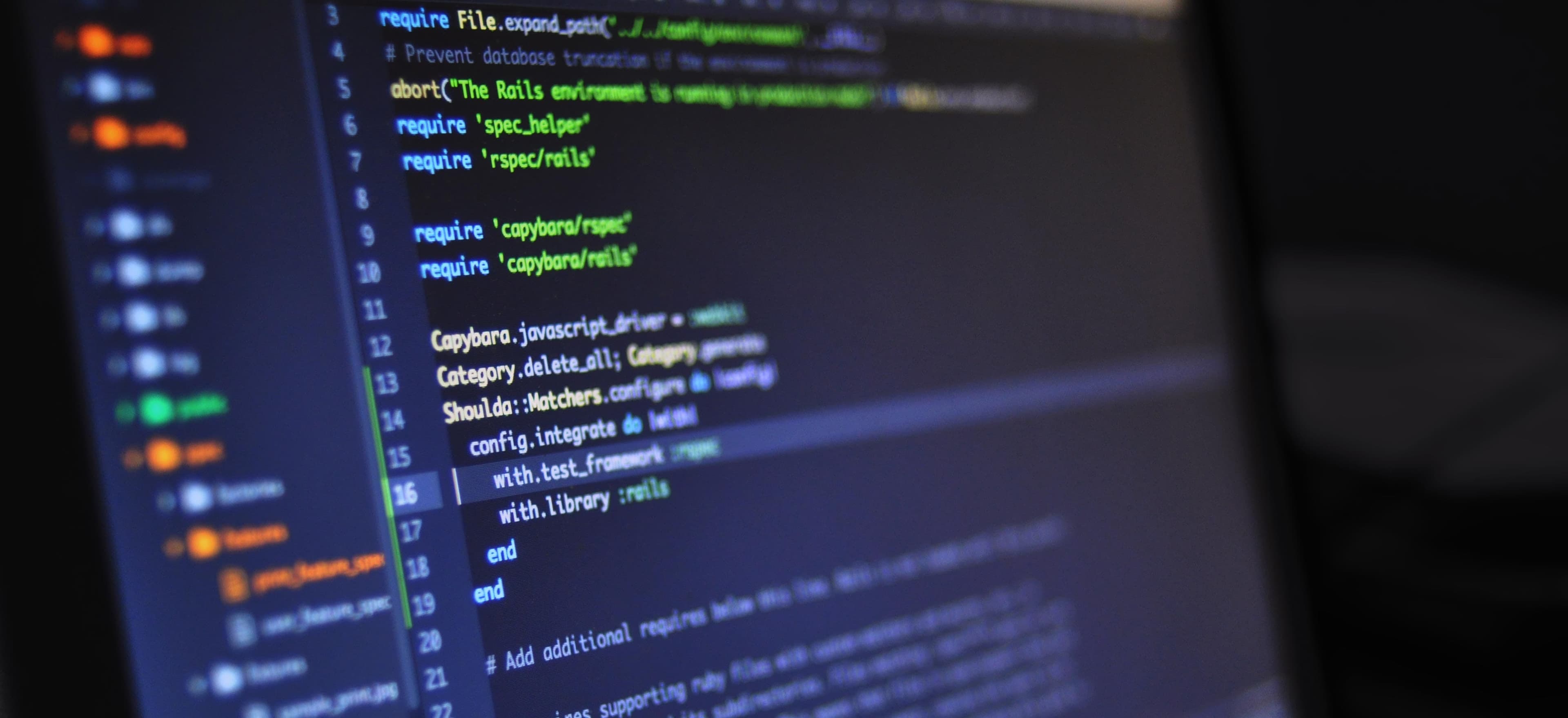
- Published on
Handling Suppressed Exceptions in Java 7: A Guide
Java has long been celebrated for its robust exception handling. With the introduction of Java 7, the language took a significant step forward in this aspect by introducing the concept of suppressed exceptions. This feature allows developers to manage multiple exceptions that may arise in a single try-with-resources block.
In this blog post, we will explore what suppressed exceptions are, why they are essential, and how you can effectively handle them in Java 7.
Understanding Suppressed Exceptions
In simple terms, a suppressed exception occurs when a close method (like closing a FileInputStream
or BufferedReader
) throws an exception while another exception is already being thrown. Instead of losing the first exception due to the second one, Java 7 lets you "suppress" the secondary exception, allowing you to report on both issues.
Why Are Suppressed Exceptions Important?
-
Data Integrity: In real-world applications, resource management is crucial. For example, if you open a database connection and also read from a file, both operations could fail, and you would like to know about both failures instead of only one.
-
Enhanced Debugging: Developers get more comprehensive error logs, making debugging easier. When you see that multiple exceptions occurred, you can narrow down the issues more effectively.
-
Cleaner Code: Java's try-with-resources statement not only makes code cleaner by handling resource closing automatically, but it also returns the ability to handle multiple exceptions neatly.
Syntax of Try-With-Resources
The try-with-resources statement automatically closes resources when they are no longer needed. Here’s a basic structure:
try (ResourceType resource = new ResourceType()) {
// Use the resource
} catch (ExceptionType e) {
// Handle exception
}
Code Snippet
Let’s look at a practical example:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class ResourceHandling {
public static void readFile(String filePath) {
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException ex) {
System.err.println("IOException: " + ex.getMessage());
// Here the IOException from FileReader is caught.
}
}
public static void main(String[] args) {
readFile("sample.txt");
}
}
Explanation of the Code
- BufferedReader and FileReader: We are using these classes to read a file line by line.
- try-with-resources: Automatically closes
BufferedReader
even if an exception occurs. - Exception Handling: An
IOException
is caught and printed.
Handling Multiple Exceptions
When working with multiple resources, you might encounter scenarios where exceptions can be thrown from more than one resource. Here’s how suppressed exceptions work under such conditions.
Code Snippet with Suppressed Exceptions
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class SuppressedExample {
public static void readFiles(String file1, String file2) {
try (BufferedReader br1 = new BufferedReader(new FileReader(file1));
BufferedReader br2 = new BufferedReader(new FileReader(file2))) {
String line;
while ((line = br1.readLine()) != null) {
System.out.println(line);
}
// Simulate an error in reading the second file.
String line2;
while ((line2 = br2.readLine()) != null) {
System.out.println(line2);
}
} catch (IOException e) {
System.err.println("Caught IOException: " + e.getMessage());
for (Throwable t : e.getSuppressed()) {
System.err.println("Suppressed: " + t.getMessage());
}
}
}
public static void main(String[] args) {
readFiles("sample1.txt", "sample2.txt");
}
}
Explanation of the Enhanced Code
- Multiple Resources: We are trying to read from two files simultaneously.
- Handling Exception: If there is an error in reading either file, it catches
IOException
. - Suppressed Exceptions: We loop through the suppressed exceptions using
getSuppressed()
, which returns an array ofThrowable
.
Notes on Suppressed Exceptions
-
Equality Method: The
Throwable
class overrides theaddSuppressed
method to ensure exceptions captured as suppressed are equal to instances that occur within the same try-catch block. -
Java SE Documentation: For more detailed information, refer to the Java SE Documentation.
-
Best Practices: Always check and log suppressed exceptions when catching multiple exceptions. This will provide better insight into what went wrong.
Use Cases for Suppressed Exceptions
-
File Handling: As illustrated above, when dealing with multiple file operations, suppressed exceptions provide a complete picture of any issues.
-
Database Operations: When performing multiple database queries, if a connection to the database fails, but there were also errors in executing queries, suppressed exceptions will let you log both types of failures.
-
Network Connections: While sending and closing network requests or responses, if either operation fails, suppressed exceptions help capture both exceptions.
To Wrap Things Up
Java 7’s introduction of suppressed exceptions fundamentally changed the way developers handle multiple exceptions arising from try-with-resources statements. The clarity and comprehensiveness provided by this feature are invaluable for writing robust applications.
As you incorporate these practices into your coding habits, you'll find that handling multiple exceptions doesn’t just improve your error logs but also increases the reliability of your applications.
This blog post should act as a starting point for understanding and implementing suppressed exceptions effectively in your Java applications. Happy coding!