Common Pitfalls When Using ArchUnit for Code Validation
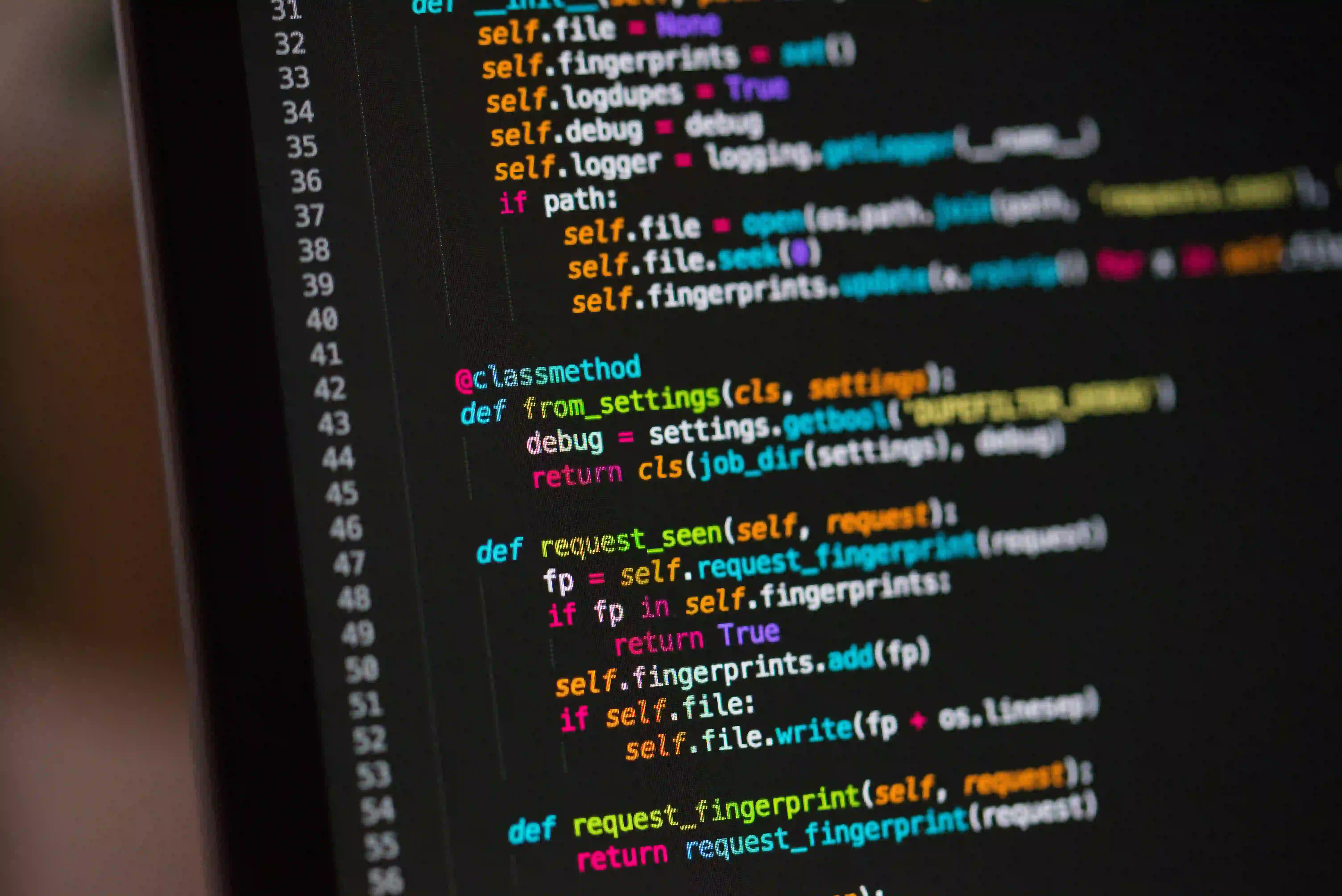
Common Pitfalls When Using ArchUnit for Code Validation
ArchUnit is a powerful library for checking architectural constraints in Java applications, ensuring that your code adheres to defined rules. It allows developers to write unit tests to validate the structure of their codebase—this includes package dependencies, class visibility, and naming conventions among others. However, despite its powerful features, several pitfalls can arise when integrating ArchUnit into your projects. This blog post will discuss common pitfalls when using ArchUnit for code validation and provide guidance on how to navigate them effectively.
What is ArchUnit?
Before we delve into the common pitfalls, let’s quickly summarize what ArchUnit is. ArchUnit is a testing library for checking architectural rules in Java code. It allows developers to write rules in Java that validate whether the code adheres to its intended architecture. For a complete overview of its functionality, visit ArchUnit Documentation.
Common Pitfalls
1. Overcomplicating Rules
The Issue
One of the most common pitfalls is creating overly complicated rules that are difficult to understand and maintain. When rules are convoluted, they hinder the development process rather than help it.
The Solution
Keep rules simple and digestible. Clearly document each rule, explaining its purpose and why it exists. Simplicity is key to effective code validation.
Code Example
import static com.tngtech.archunit.lang.syntax.ArchRuleDefinition.classes;
classes()
.that().resideInAPackage("..service..")
.should().not dependOnClassesThat().resideInAnyPackage("..controller..")
.because("Service classes should not depend directly on controller classes.")
.check(importedClasses);
In this example, the rule is straightforward—it states that service classes should not depend on controller classes. The because
clause clarifies the rationale behind the rule, enhancing understanding.
2. Ignoring Feedback and Warnings
The Issue
Developers often overlook the feedback provided by ArchUnit when rules are violated. This can lead to buildup of architectural debts, making future refactoring more cumbersome.
The Solution
Treat ArchUnit warnings seriously. Regularly run your architectural tests as part of your Continuous Integration (CI) pipeline. This encourages prompt attention to architectural violations.
3. Neglecting to Update Rules
The Issue
As codebases evolve, architectural rules need to adapt. However, some teams forget to revise existing rules, leading to obsolete checks that produce false positives.
The Solution
Establish a routine for reviewing and updating ArchUnit rules. This might involve quarterly check-ins to assess whether the existing rules are still relevant.
Code Example
import com.tngtech.archunit.core.importer.ClassFileImporter;
public class ArchitectureTest {
@Test
public void serviceClassesShouldNotDependOnControllers() {
classes().that().resideInAPackage("..service..")
.should().notDependOnClassesThat().resideInAPackage("..controller..")
.check(new ClassFileImporter().importPackages("com.myapp"));
}
}
In this test method, we are verifying the dependency rule. If the package structure changes, ensure the corresponding rules reflect those alterations.
4. Not Running Tests Frequently
The Issue
Another common mistake is running architectural tests infrequently. Doing so can set the project up for large-scale issues that are harder to fix.
The Solution
Integrate your ArchUnit tests into your standard test suite to ensure they run with every build. This practice helps maintain adherence to architectural rules over time.
5. Underestimating the Power of Grouping
The Issue
Failing to group related architectural rules can lead to fragmented and confusing tests. Each test class may hold disparate rules that aren't easily understood in context.
The Solution
Group related rules logically into single test classes. This makes it easier for teams to manage architectural checks and promotes a clearer understanding of why certain rules exist.
Code Example
import static com.tngtech.archunit.lang.syntax.ArchRuleDefinition.classes;
public class ServiceArchitectureTest {
@Test
public void serviceClassesShouldNotDependOnControllers() {
classes().that().resideInAPackage("..service..")
.should().notDependOnClassesThat().resideInAPackage("..controller..")
.check(importedClasses);
}
@Test
public void serviceClassesShouldBePublic() {
classes().that().resideInAPackage("..service..")
.should().bePublic()
.check(importedClasses);
}
}
Each rule here assesses a different aspect of the service layer architecture, making it clearer that both checks pertain specifically to service classes.
6. Missing Out on Automatic Imports
The Issue
ArchUnit allows you to import classes automatically, but some developers may neglect this feature, resulting in repetitive code and human error.
The Solution
Take advantage of automatic imports. Use the ClassFileImporter
effectively to pull in all relevant classes for your architectural checks.
Code Example
import com.tngtech.archunit.core.importer.ClassFileImporter;
ClassFileImporter classFileImporter = new ClassFileImporter();
importedClasses = classFileImporter.importPackages("com.myapp");
This snippet automatically imports all classes from the specified package, ensuring that the rules have access to the correct context without manual imports.
7. Over-Relying on ArchUnit
The Issue
While ArchUnit provides excellent validation for architecture, it cannot replace overall code quality practices. Developers might shift their focus solely to ArchUnit checks while neglecting crucial code reviews, unit tests, and integration tests.
The Solution
Be holistic in your approach to quality. Use ArchUnit as part of a larger suite of practices, including regular code reviews and comprehensive testing strategies.
In Conclusion, Here is What Matters
Integrating ArchUnit into your Java projects can vastly improve how you enforce and validate architectural rules. However, it's crucial to avoid the common pitfalls discussed above. Keep your rules simple, run tests frequently, and ensure they adapt alongside your project. By doing so, you can leverage ArchUnit’s capabilities while maintaining a clean and efficient codebase.
For more information and best practices on using ArchUnit effectively, visit the ArchUnit GitHub repository or check out real-world examples on Medium.
Happy coding!