Mastering Optional Parameters in Java: Avoiding Common Pitfalls
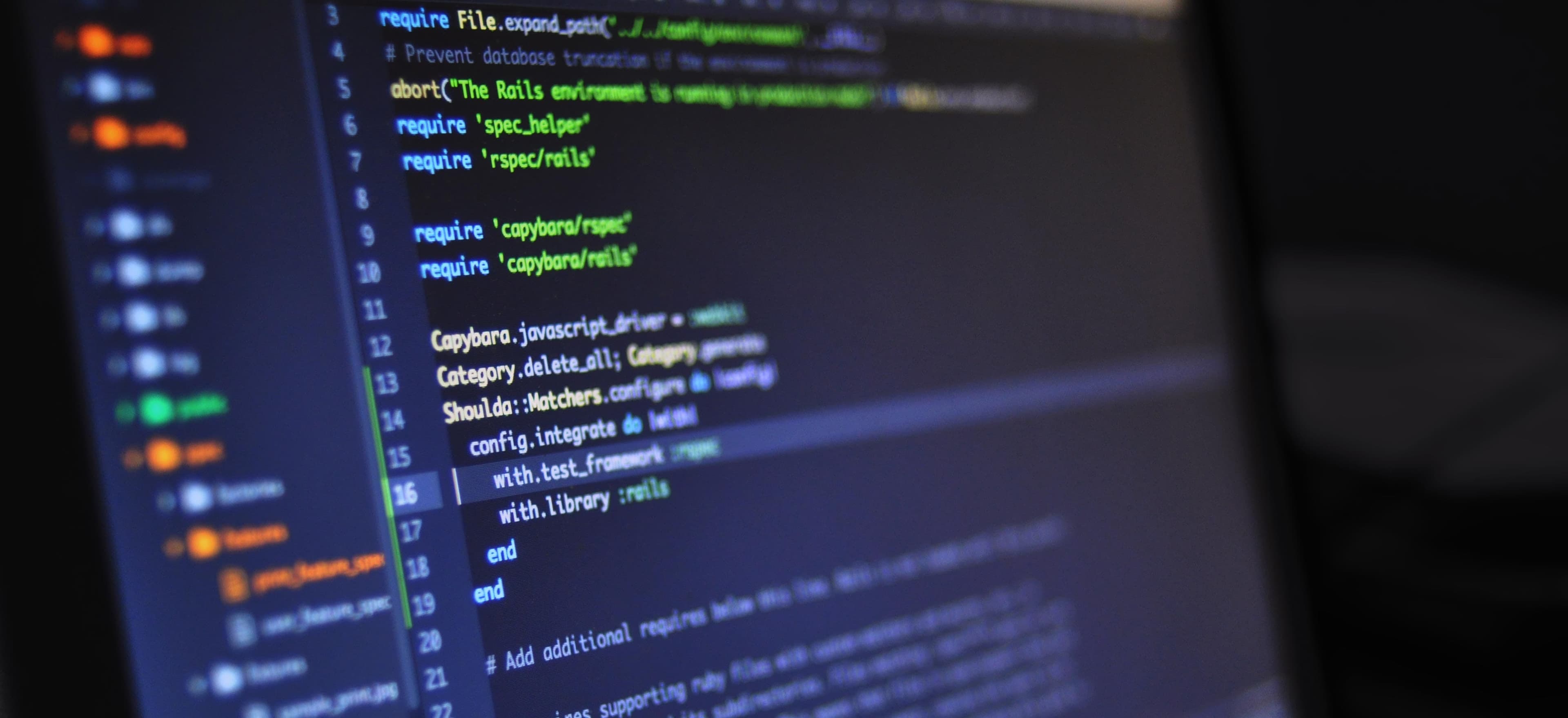
- Published on
Mastering Optional Parameters in Java: Avoiding Common Pitfalls
Java is a robust programming language that emphasizes readability and maintainability. One of its structured approaches is how it handles method parameters. While Java does not natively support optional parameters the way other languages do (like Python), developers often seek to implement similar functionality. This blog post will explore optional parameters in Java, common pitfalls to avoid, and best practices to utilize effectively.
What are Optional Parameters?
Optional parameters allow methods to be called with varying numbers of arguments. This means you can define a method that can accept one, two, or even three parameters, where only a subset of these parameters needs to be supplied. Although Java doesn’t directly support optional parameters, there are several strategies to achieve this.
Common Strategies to Implement Optional Parameters
- Method Overloading
- Varargs (Variable Arguments)
- Builder Pattern
- Using
Optional
Class
Method Overloading
Method overloading is one of the most common techniques used to simulate optional parameters in Java. By defining multiple methods with the same name but different signatures, developers can achieve the desired flexibility.
Here’s an example:
public class Greeting {
public void sayHello(String name) {
System.out.println("Hello, " + name + "!");
}
public void sayHello() {
sayHello("Guest"); // Default parameter
}
}
Explanation
In the example above, the sayHello
method is overloaded to allow for a default greeting. If no name is provided, it defaults to "Guest". This method is straightforward and easy to understand, ensuring that users can call the method without having to provide excessive parameters.
Varargs (Variable Arguments)
Java also has a feature called varargs, which allows developers to pass a variable number of arguments to a method. This provides another way to implement optional parameters without using method overloading.
Here’s how you can use varargs:
public class Logger {
public void log(String... messages) {
for (String message : messages) {
System.out.println(message);
}
}
}
Explanation
In this example, the log
method can accept a variable number of String
messages. The flexibility here is significant; however, care should be taken when using varargs as it can lead to code that is less transparent and harder to debug.
Builder Pattern
The builder pattern is a more advanced solution that is particularly useful when dealing with multiple parameters. It is best utilized for constructing complex objects with numerous optional parameters.
public class User {
private String username;
private int age;
private String email;
private User(Builder builder) {
this.username = builder.username;
this.age = builder.age;
this.email = builder.email;
}
public static class Builder {
private String username;
private int age;
private String email;
public Builder(String username) {
this.username = username;
}
public Builder age(int age) {
this.age = age;
return this;
}
public Builder email(String email) {
this.email = email;
return this;
}
public User build() {
return new User(this);
}
}
}
Explanation
The builder pattern allows for the construction of User
objects in a more readable way. This pattern reduces the number of parameters in a constructor and makes the code cleaner. By providing setter-like methods, you can specify only the options you want. Moreover, it prevents the problems associated with constructor overloading.
Using the Optional
Class
In addition to the above methods, Java 8 introduced the Optional
class, which can be utilized to represent optionality more explicitly.
import java.util.Optional;
public class Configuration {
private Optional<String> theme;
private Optional<Boolean> notificationsEnabled;
public Configuration(Optional<String> theme, Optional<Boolean> notificationsEnabled) {
this.theme = theme;
this.notificationsEnabled = notificationsEnabled;
}
public void displaySettings() {
System.out.println("Theme: " + theme.orElse("Default"));
System.out.println("Notifications: " + notificationsEnabled.orElse(false));
}
}
Explanation
The Optional
class allows you to handle the absence of a parameter rather elegantly. By using Optional
, intent is made clear—parameters can be absent, and code handling those cases can be managed seamlessly through methods like orElse
.
Common Pitfalls to Avoid
While implementing optional parameters in Java, there are several common pitfalls that developers should avoid:
-
Overusing Method Overloading: While method overloading can be handy, overdoing it can lead to code that is hard to read and maintain. Always strive for clarity.
-
Confusing Varargs Behavior: When using varargs, make sure to differentiate between passing a single parameter versus multiple. It can lead to unintended behavior.
-
Neglecting Null Handling: If you opt for traditional default values (like using
null
), always handle potentialNullPointerExceptions
. -
Misusing the
Optional
Class: WhileOptional
is powerful, it should not be used for every parameter. Use it wisely to retain clarity.
Best Practices for Using Optional Parameters
-
Clarify Default Values: When using optional parameters, make the default values clear through documentation or method names.
-
Leverage Documentation: Always provide JavaDoc comments explaining which parameters are optional and what their default values are.
-
Consistency: Keep your approach consistent across your codebase. Whether you decide to use method overloading or builders, stick with it to minimize confusion.
-
Limit Usage: Don’t go overboard with optional parameters. Too many can complicate code and reduce readability.
-
Use Enums for Fixed Sets of Parameters: For a set of options, consider using enums instead of boolean flags, making the code more self-explanatory.
The Closing Argument
Mastering optional parameters in Java plays a pivotal role in writing flexible, clean, and maintainable code. Whether you choose method overloading, varargs, the builder pattern, or the Optional
class, understanding the strengths and drawbacks of each method is critical. By avoiding common pitfalls and adhering to best practices, you can enhance both the readability and functionality of your applications.
For more on Java best practices, check out the Java documentation and resources like Effective Java by Joshua Bloch.
By leveraging these concepts, you can take your Java programming skills to the next level, ensuring your code remains effective and user-friendly. Happy coding!
Checkout our other articles