Mastering Lazy Loading in PrimeFaces DataTable: A Guide
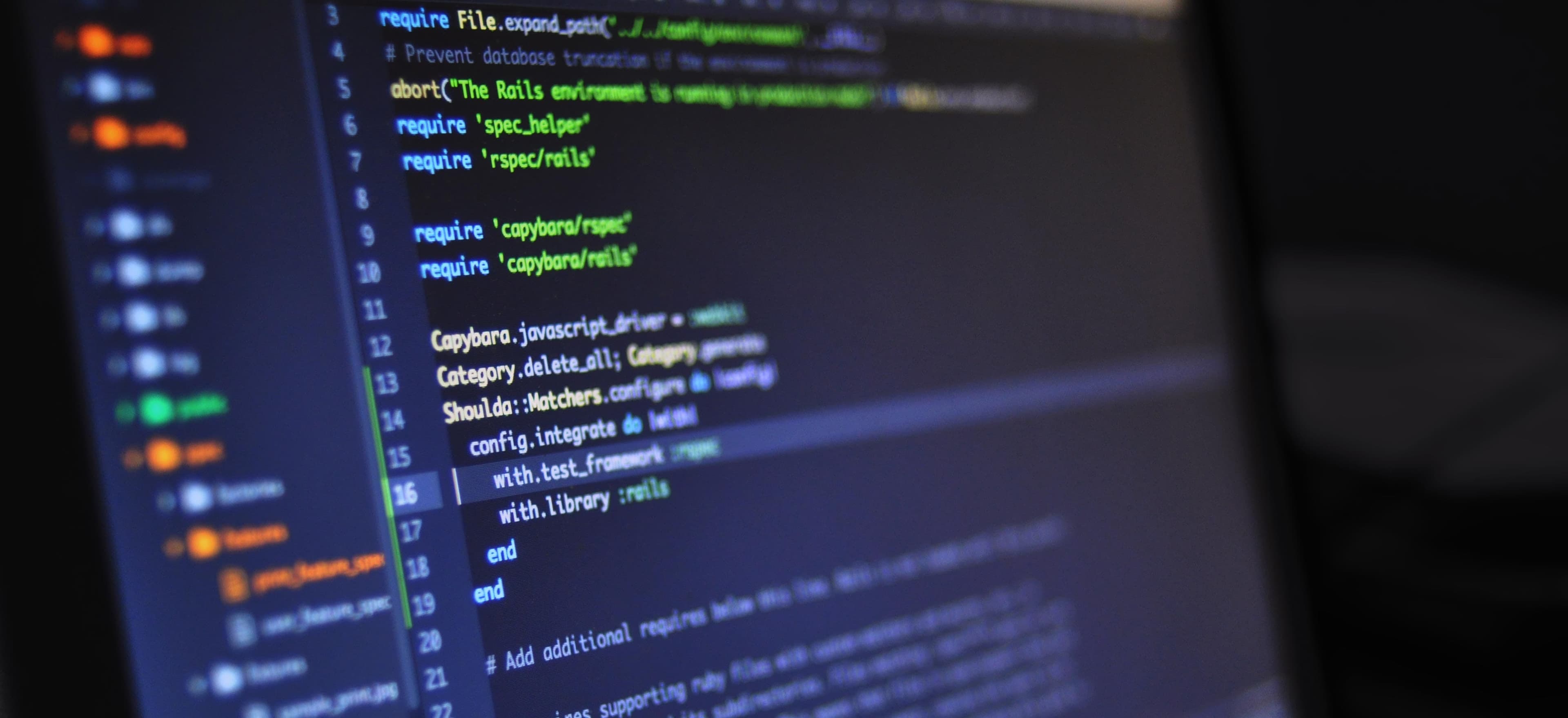
- Published on
Mastering Lazy Loading in PrimeFaces DataTable: A Guide
Lazy loading is an essential technique in modern web applications, especially when dealing with extensive datasets. It allows for the efficient loading of data on demand, thereby minimizing the initial load time and enhancing user experience. In this post, we'll explore the concept of lazy loading as it pertains to PrimeFaces DataTable. We'll also delve into implementation examples, its benefits, and why it is a valuable feature for developers.
Understanding Lazy Loading
What is Lazy Loading?
Lazy loading is a design pattern that postpones loading an object until it is necessary. In the context of web applications, it generally refers to loading data only when it is being requested by the user, rather than fetching an entire dataset upfront.
Why Use Lazy Loading?
- Performance: By loading only a subset of data when needed, the application requires less memory and network bandwidth.
- Scalability: It can handle large datasets more effectively, allowing your application to scale without performance degradation.
- User Experience: Users can interact with the application sooner, as they are not waiting for all data to load at once.
PrimeFaces DataTable Overview
PrimeFaces is a popular UI component library for JavaServer Faces (JSF) applications. It provides a rich set of components, including the DataTable, which can display and manage data in a structured format.
Benefits of PrimeFaces DataTable
- Rich User Interface: Provides various built-in features such as pagination, sorting, and filtering.
- Customization: Offers a flexible API that allows developers to customize components easily.
- Integration: Works seamlessly with JSF and other Java enterprise applications.
Implementing Lazy Loading in PrimeFaces DataTable
Step 1: Setting Up Your Environment
Ensure you have a Java EE environment set up with PrimeFaces integrated into your JSF project. You can add PrimeFaces dependencies through Maven:
<dependency>
<groupId>org.primefaces</groupId>
<artifactId>primefaces</artifactId>
<version>PF_VERSION_HERE</version> <!-- Use the latest version -->
</dependency>
Step 2: Create Your Data Model
For this guide, let’s assume you already have an Entity called Product
. Here is an example of the Product
class:
public class Product {
private Long id;
private String name;
private double price;
// Getters and Setters
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
Step 3: Implement LazyDataModel
In order to enable lazy loading, we create a custom implementation of the LazyDataModel
class. Below is an example:
import org.primefaces.model.LazyDataModel;
import org.primefaces.model.SortOrder;
import javax.faces.model.DataModel;
import java.util.List;
import java.util.Map;
public class ProductLazyDataModel extends LazyDataModel<Product> {
private ProductService productService;
public ProductLazyDataModel(ProductService productService) {
this.productService = productService;
}
@Override
public List<Product> load(int first, int pageSize, String sortField, SortOrder sortOrder, Map<String, Object> filters) {
// Fetch data from service layer with lazy loading parameters
List<Product> products = productService.getProducts(first, pageSize, sortField, sortOrder, filters);
this.setRowCount(productService.countProducts(filters)); // Set the total row count
return products; // Return the data
}
}
Why Use LazyDataModel?
- Encapsulates Logic: It encapsulates the logic for loading data in a way that can be extended or modified easily.
- Automatic Pagination: PrimeFaces automatically manages pagination for you, saving you boilerplate code.
- Reduced Database Load: By only fetching the requested data, this method reduces the load on your database.
Step 4: Setting Up the ProductService
Your service class should implement the logic to retrieve products based on provided parameters, focusing on pagination and filtering.
public class ProductService {
// Implement your data repository or database access layer as needed
public List<Product> getProducts(int first, int pageSize, String sortField, SortOrder sortOrder, Map<String, Object> filters) {
// You should apply your business logic here by using a data repository
// Example: Using JPA Criteria API to create dynamic queries
return // fetched product list;
}
public int countProducts(Map<String, Object> filters) {
// Return total number of products based on filters
return // count;
}
}
Step 5: Configuring Your JSF Page
Finally, connect your lazy data model to a PrimeFaces DataTable in your JSF page.
<h:form>
<p:dataTable value="#{productBean.lazyModel}" var="product"
lazy="true" rows="10" paginator="true"
id="datatable">
<p:column sortBy="#{product.name}" filterBy="#{product.name}">
<f:facet name="header">Name</f:facet>
#{product.name}
</p:column>
<p:column sortBy="#{product.price}" filterBy="#{product.price}">
<f:facet name="header">Price</f:facet>
#{product.price}
</p:column>
</p:dataTable>
</h:form>
Benefits of This Implementation
- Efficient Data Handling: You only load what is required.
- Simple Integration: The components fit well within the JSF lifecycle.
- Robust Customization: You have the flexibility to expand and modify as needed.
In Conclusion, Here is What Matters
Mastering lazy loading in PrimeFaces DataTable can drastically enhance the performance and usability of your web applications. By fetching data as needed, you optimize resource usage and improve user interaction.
For more detailed information on implementing lazy loading, consider visiting the PrimeFaces User Guide and exploring more about Java Data Management. Thoroughly understanding this pattern can be a game-changer in how you develop data-rich applications.
Happy Coding!
Checkout our other articles