Common Pitfalls in Lightweight Integration Tests for Eclipse Extensions
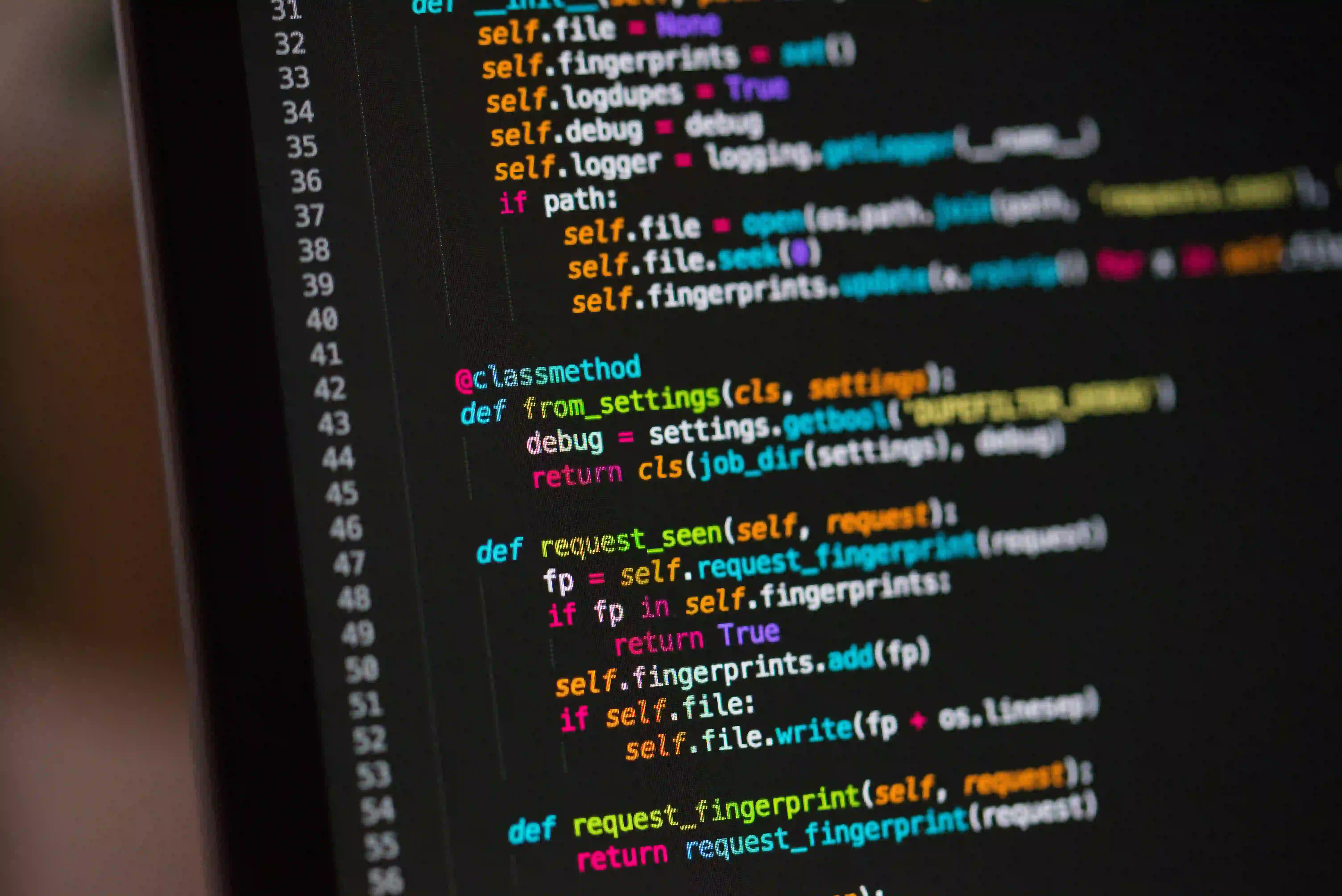
Common Pitfalls in Lightweight Integration Tests for Eclipse Extensions
Integration testing is a critical phase in the development lifecycle of any software, and this holds true especially for Eclipse extensions. However, when dealing with Lightweight Integration Tests (LIT), developers often encounter various pitfalls that can lead to misleading results and additional debugging time. This blog post will explore common pitfalls when writing lightweight integration tests for Eclipse extensions, providing practical code examples and insights to help you avoid these traps.
Understanding Lightweight Integration Tests
Before diving into the common pitfalls, let's clarify what Lightweight Integration Tests are. LITs are designed to test components of an application without needing the entire application environment. In the context of an Eclipse extension, this means testing specific features with minimal overhead, essentially speeding up the testing process.
Why Use Lightweight Integration Tests?
- Speed: Since they’re less resource-intensive, LITs run faster than full integration or system tests.
- Focused Testing: LITs enable developers to target specific components, ensuring they work independently.
- Rapid Feedback: Faster tests lead to quicker feedback, which is essential in agile development environments.
Common Pitfalls
1. Ignoring Dependencies
One of the significant pitfalls developers face is ignoring dependencies between components. LITs can produce false positives when key dependencies are mocked but not adequately representing the actual environment.
Example Code Snippet:
MyService myService = mock(MyService.class);
when(myService.getData()).thenReturn("Mock Data");
MyController controller = new MyController(myService);
String result = controller.fetchData();
assertEquals("Mock Data", result);
Why This Can Be a Problem: While the test passes, if MyService
has dependencies on other services or components (like databases or external APIs), your test won't expose issues that arise in a more integrated context.
Solution: Use integration tests to ensure that all components work harmoniously together. Consider tools like JUnit or TestNG to manage complex test scenarios.
2. Over-Mocking
While using mocks can simplify testing by isolating behavior, over-mocking can lead to rigid tests that don't actually verify behavior effectively.
Example Code Snippet:
SomeDependency dependency = mock(SomeDependency.class);
when(dependency.process()).thenReturn("Processed Mock");
MainClass mainClass = new MainClass(dependency);
String result = mainClass.execute();
Why This Is a Risk: If SomeDependency
undergoes changes in implementation, it may lead to failures that the test won’t catch because it relies on a mock response.
Solution: Aim for a balanced approach. Use mocks where necessary, but allow for some real component interactions to ensure real behavior is tested.
3. Unclear Test Objectives
Each test should aim for clarity in its purpose. Vague tests make it impossible to determine what's being validated.
Example Code Snippet:
@Test
public void testFunction() {
// ... Test logic
}
Why This Is Ineffective: Without a clear test name or description, you cannot unambiguously understand what this test covers or what it's supposed to validate.
Solution: Adopt clear naming conventions and include comments explaining the purpose of the test. Naming could be like testServiceReturnsCorrectDataOnSuccess
.
4. Not Cleaning Up After Tests
Failing to clean up between tests can lead to state leakage. This may result in tests impacting one another, which can produce inconsistent results.
Example Code Snippet:
@Test
public void testA() {
// Setup some global state
}
@Test
public void testB() {
// Assumes state from testA is not affected.
}
Why This Matters: If testA
modifies a global state or a static member, testB
may behave unexpectedly.
Solution: Use setup and teardown methods in JUnit, such as @Before
and @After
, to ensure the tests are clean and self-contained:
@Before
public void setUp() {
// Initialize state before each test
}
@After
public void tearDown() {
// Reset state after each test
}
5. Lack of Testing Edge Cases
Testing edge cases is often overlooked, focusing instead on typical usage scenarios.
Example Code Snippet:
public int divide(int a, int b) {
return a / b;
}
Why This Is a Problem: If there are no tests for edge cases, such as dividing by zero, you may encounter runtime errors that are not caught during testing.
Solution: Ensure that all edge cases are covered. This might look like:
@Test(expected = ArithmeticException.class)
public void testDivideByZero() {
divide(1, 0);
}
Tools and Best Practices
Employing the right tools and adhering to best practices can significantly reduce potential pitfalls.
- Testing Frameworks: Use JUnit or Mockito for efficient unit testing and mocking.
- Continuous Integration (CI): Integrate your tests into CI to ensure they run with every build and prevent regression.
- Code Reviews: Engage your peers in code reviews focused on testing strategies and coverage.
For those who want a deeper understanding of testing in Eclipse, check out the Eclipse Developer Resources for guidelines and strategies.
Wrapping Up
Lightweight integration tests are indispensable for validating the functionality of Eclipse extensions, but avoiding the aforementioned pitfalls is crucial. By being mindful of dependencies, maintaining clarity of test objectives, and ensuring thorough testing practices, you can enhance the reliability of your tests.
Integrate effective testing strategies, and you'll pave the way toward a smoother development experience. Don’t forget, the efficiency of your testing process mirrors the quality of your software—invest the time to get it right.
For further reading on integration testing strategies, consider visiting Martinfowler.com. Happy coding!