Mastering Thread Safety: Avoiding Common Pitfalls
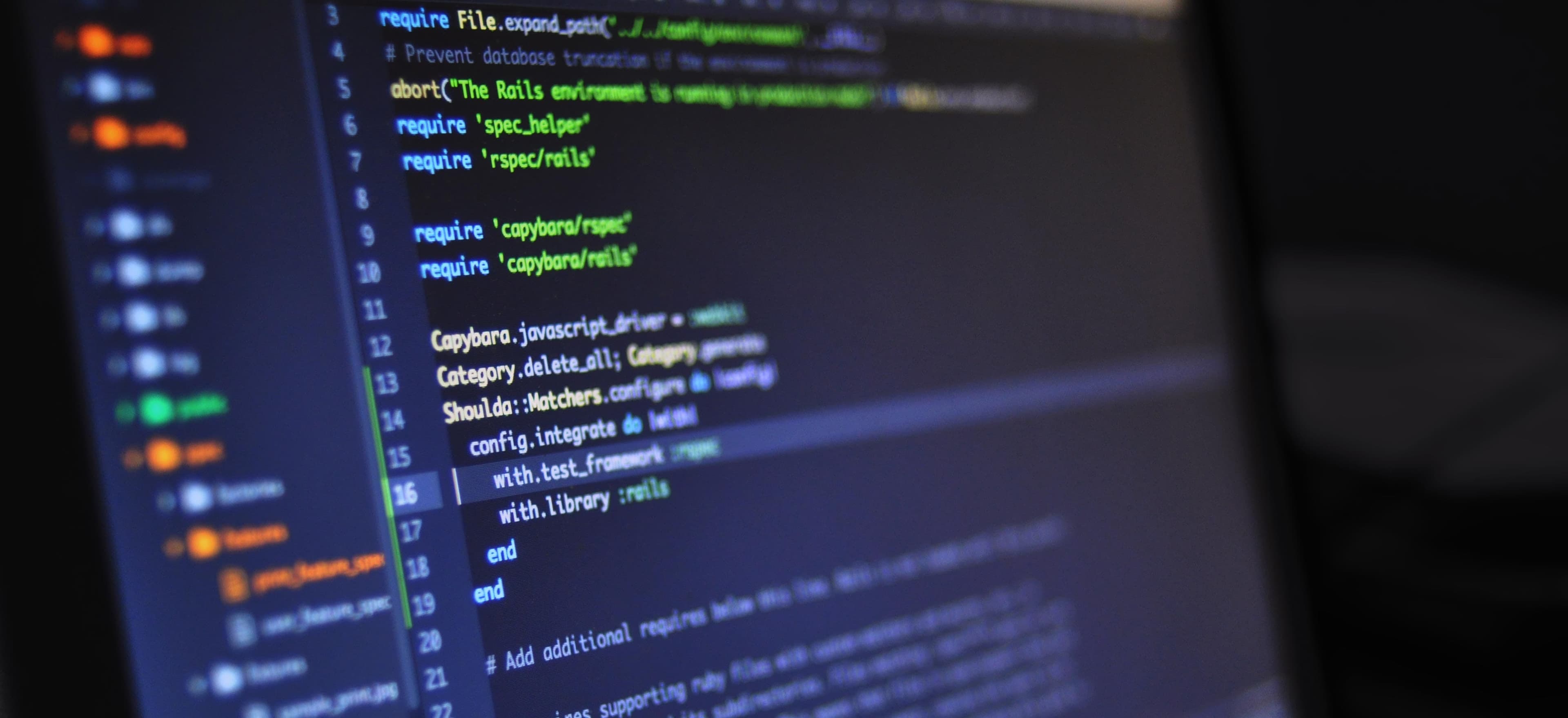
- Published on
Mastering Thread Safety: Avoiding Common Pitfalls
In today's fast-paced programming world, multi-threading is a pivotal concept that enhances application performance and responsiveness. However, it comes with its own set of challenges, primarily around thread safety. In this blog post, we will explore what thread safety means, the common pitfalls developers encounter, and ways to sidestep those pitfalls with practical examples in Java.
Understanding Thread Safety
Thread safety refers to a program's ability to function correctly during simultaneous execution by multiple threads. When multiple threads access shared resources (like variables, data structures, etc.), there is a risk of encountering unexpected behavior or data corruption. Ensuring thread safety is crucial in multi-threaded applications.
Why is Thread Safety Important?
- Data Integrity: Prevents corrupted or inconsistent data.
- Predictability: Builds trust that system behavior remains consistent.
- Avoids Bugs: Reduces the potential for hard-to-diagnose concurrency bugs.
Common Pitfalls in Java Thread Safety
1. Race Conditions
A race condition occurs when two or more threads access shared resources concurrently, and at least one thread modifies that resource. This often leads to unexpected results.
Example of a Race Condition
Here's a code snippet illustrating a race condition:
public class RaceConditionExample {
private int counter = 0;
public void increment() {
counter++; // Not thread-safe!
}
public int getCounter() {
return counter;
}
}
In this case, if multiple threads call the increment()
method at the same time, the final value of counter
could be incorrect due to concurrent updates. To learn more about race conditions, check out the Java Concurrency Tutorial.
Solution: Synchronization
You can use the synchronized
keyword to ensure that only one thread can execute a method or block of code at a time:
public class SynchronizedCounter {
private int counter = 0;
public synchronized void increment() {
counter++;
}
public synchronized int getCounter() {
return counter;
}
}
2. Deadlocks
Deadlock occurs when two or more threads are waiting for each other to release resources, creating a cycle of dependencies that halts execution.
Example of Deadlock
Consider the following scenario:
public class DeadlockExample {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void methodA() {
synchronized (lock1) {
synchronized (lock2) {
// Perform actions
}
}
}
public void methodB() {
synchronized (lock2) {
synchronized (lock1) {
// Perform actions
}
}
}
}
Here, methodA
locks lock1
and then lock2
, while methodB
locks lock2
and then lock1
. If both methods are invoked simultaneously from two different threads, they may end up waiting for each other indefinitely.
Solution: Lock Ordering
One way to prevent deadlock is to always acquire locks in a consistent order:
public void methodA() {
synchronized (lock1) {
synchronized (lock2) {
// Perform actions
}
}
}
public void methodB() {
synchronized (lock1) {
synchronized (lock2) {
// Perform actions
}
}
}
By acquiring lock1
before lock2
in both methods, you reduce the risk for deadlocks.
3. Starvation
Starvation occurs when a thread is perpetually denied access to the resources it needs for execution, due to other threads constantly being prioritized.
Example of Starvation
If you implement a priority-based threading model in Java, you might inadvertently create conditions for starvation:
public class StarvationExample {
public static void main(String[] args) {
Thread thread1 = new Thread(() -> {
while (true) {
// High priority task
}
});
Thread thread2 = new Thread(() -> {
while (true) {
// Low priority task
}
});
thread1.setPriority(Thread.MAX_PRIORITY);
thread2.setPriority(Thread.MIN_PRIORITY);
thread1.start();
thread2.start();
}
}
In this case, thread2
, with a low priority, might never get CPU time to run.
Solution: Fair Locking
Java’s ReentrantLock
provides an optional fairness parameter which ensures that threads acquire locks in the order they're waiting:
import java.util.concurrent.locks.ReentrantLock;
public class FairLockExample {
private final ReentrantLock lock = new ReentrantLock(true); // Fairness
public void action() {
lock.lock();
try {
// Perform actions
} finally {
lock.unlock();
}
}
}
By setting true
, you're creating a fair lock that serves waiting threads in the order they requested access.
Thread-Safe Collections
Java’s collections framework also provides thread-safe alternatives. For instance:
- Use
ConcurrentHashMap
instead ofHashMap
. - Consider
CopyOnWriteArrayList
for lists if read operations vastly outnumber writes.
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentMapExample {
private ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
public void safePut(String key, Integer value) {
map.put(key, value); // Thread-safe put operation
}
public Integer safeGet(String key) {
return map.get(key); // Thread-safe get operation
}
}
Best Practices for Achieving Thread Safety
- Minimize Shared State: The less shared data, the fewer the chances of conflict.
- Use Immutable Objects: Immutable classes are inherently thread-safe. Consider using them where feasible.
- Opt for Higher-Level Concurrency APIs: Java's
java.util.concurrent
package provides more advanced tools that can help manage complexity. - Test Concurrent Code: Use tools and techniques to identify potential thread safety issues during development and testing.
Final Thoughts
Mastering thread safety is essential for any Java developer who wants to build robust multi-threaded applications. Understanding common pitfalls such as race conditions, deadlocks, and starvation—and knowing how to mitigate them—can significantly improve the stability and performance of your applications.
By incorporating synchronization, enforcing lock ordering, and choosing the right data structures, you can effectively navigate the complexity of concurrent programming in Java. For further reading, check Java Concurrency in Practice, a foundational book on the subject.
As you embark on diving deeper into multi-threading, remember: always keep thread safety in your mind. Happy coding!
Checkout our other articles