Resolving IncompatibleClassChangeError in Java Applications
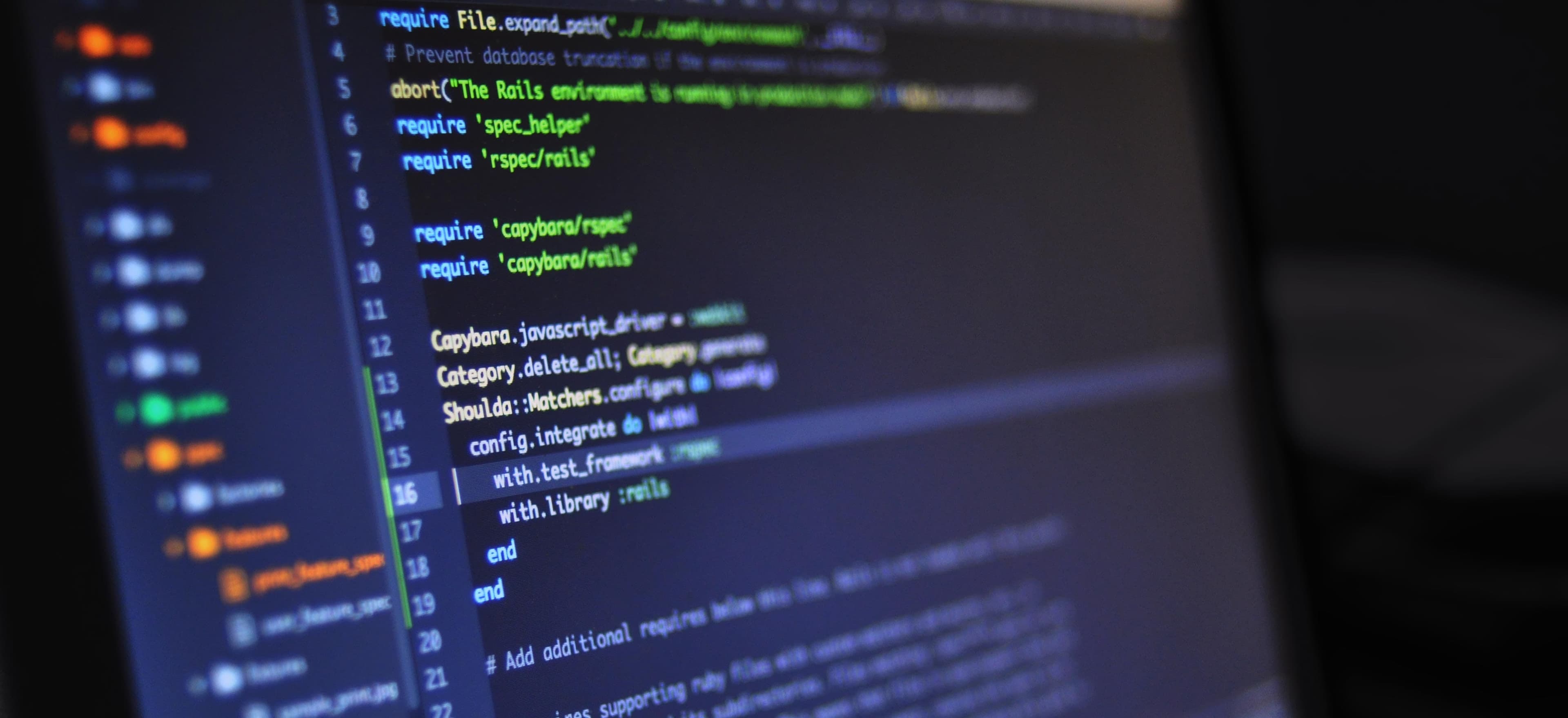
- Published on
Resolving IncompatibleClassChangeError in Java Applications
Java is a powerful programming language known for its portability and robust architecture. However, like any complex technology, developers can encounter various exceptions, one of the most perplexing being IncompatibleClassChangeError
. This error occurs during runtime, usually signaling underlying issues in the application's structure. In this blog post, we'll explore what causes IncompatibleClassChangeError
, how you can identify it, and practical steps to resolve it.
Understanding IncompatibleClassChangeError
What is IncompatibleClassChangeError?
IncompatibleClassChangeError
is a subclass of LinkageError
that arises when the Java Virtual Machine (JVM) finds a class that has changed in a way that is incompatible with its previous version. This can occur when you alter class definitions, interfaces, or superclass relationships that other classes depend on.
When Does This Error Occur?
This error can pop up in several scenarios, including:
- Changes in class structure across different versions of a library.
- Inconsistent implementation of interfaces.
- Loading multiple versions of the same class or jar file.
Example Scenario
Imagine you have a library that performs certain operations using an interface. If you switch to a newer version of this library where the interface has changed its method signatures or class hierarchy, you may encounter an IncompatibleClassChangeError
.
Here’s a simplified example:
Library Version 1:
// Version 1 of MyLibrary
public interface MyInterface {
void doSomething();
}
public class MyClass implements MyInterface {
public void doSomething() {
System.out.println("Doing something in version 1.");
}
}
Library Version 2:
// Version 2 of MyLibrary
public interface MyInterface {
void doSomething(String param);
}
public class MyClass implements MyInterface {
public void doSomething(String param) {
System.out.println("Doing something in version 2 with param: " + param);
}
}
If you try to run code that uses an instance of MyClass
from Version 1 while the library is at Version 2, it can lead to an IncompatibleClassChangeError
.
Diagnosing the Error
To effectively resolve this error, you first need to identify the source of the issue. Here are steps to help you diagnose it:
-
Check Your Classpath: Ensure that you are not including multiple versions of the same library in your project dependencies. Use tools like Maven or Gradle to visualize your dependencies.
-
Review Changes: If you've updated libraries, check the changelog for breaking changes or alterations in class hierarchies.
-
Debugging: Use debugging tools to trace through the stack at the point where the error occurs. Look for discrepancies in method calls and their signatures.
-
Version Control: Ensure you are using the correct versions of the libraries across all parts of your application. Consistency is key.
Resolution Steps
Once you've diagnosed the issue, follow these steps to resolve it:
Step 1: Clean Your Project
Sometimes build artifacts can cause inconsistencies. Make sure to clean your project using your IDE or build tool:
-
For Maven:
mvn clean install
-
For Gradle:
./gradlew clean build
Step 2: Update Dependencies
If you find that your libraries are outdated or inconsistent, update them to compatible versions. Use a dependency management tool like Maven or Gradle.
Example Maven Dependency Update:
<dependency>
<groupId>com.example</groupId>
<artifactId>my-library</artifactId>
<version>2.0.0</version>
</dependency>
Step 3: Align Interfaces and Implementations
Ensure that all classes implementing interfaces or extending superclass methods conform to the signatures expected by the superclass or interface:
public class MyClass implements MyInterface {
// Ensure that this matches the new method signature
public void doSomething(String param) {
System.out.println("Doing something in version 2 with param: " + param);
}
}
Step 4: Check for Shadowed Classes
If you're using third-party libraries, ensure you are not accidentally shadowing other classes. Avoiding the same package structure in custom code as in libraries can prevent class loading issues.
Step 5: Use Version Constraints
In multi-module or microservices architectures, consider using tools such as Maven Enforcer Plugin to enforce dependency constraints.
Step 6: Testing
After making changes, rigorously test your application. Automated tests can help catch potential issues early before deploying to production.
Conclusion
While IncompatibleClassChangeError
can be frustrating, understanding the causes and implementing systematic resolution strategies can mitigate its impact on your development workflow. Keeping your dependencies updated and consistent is fundamental for any Java application.
For a deeper dive into managing Java dependencies, consider checking out Maven Dependency Management or Gradle Dependency Management.
Additionally, if you want to enhance your Java skills, exploring topics like Java Exception Handling can be invaluable in navigating error handling effectively.
By adopting good practices and remaining vigilant about dependency changes, you can successfully navigate the complexities of Java error handling in your applications. Happy coding!